Event Listeners Go to the co_credit.js file in your editor. Create an event listener for the window load event that retrieves the field values attached to the query string of the page’s URL. Add the following to the event listener’s anonymous function: Create the orderData variable that stores the query string text from the URL. Slice the orderData text string to remove the first ? character, replace every occurrence of the + character with a blank space, and decode the URI-encoded characters. Split the orderData variable at every occurrence of a & or = character and store the substrings in the orderFields array variable. Write the following values from the orderFields array into the indicated fields of the order form: orderFields[3] into the modelName field orderFields[5] into the modelQty field orderFields[7] into the orderCost field orderFields[9] into the shippingType field orderFields[13] into the shippingCost field orderFields[15] into the subTotal field orderFields[17] into the salesTax field orderFields[19] into the cartTotal field Add another event listener for the window load event that runs different validation event handlers when the page is loaded by the browser. Add the following code to the anonymous function for load event: Run the runSubmit() function when the subButton is clicked. Run the validateName() function when a value is input into the cardHolder field. Run the validateNumber() function when a value is input into the cardNumber field. Run the validateDate() function when a value is input into the expDate field. Run the validateCVC() function when a value is input into the cvc field. JavaScript Functions Create the runSubmit() function that is run when the form is submitted. Within the function add commands to run the validateName(), validateCredit(), validateNumber(), validateDate(), and validateCVC() functions. Create the validateDate() function. The purpose of this function is to validate the credit card expiration date stored in the expDate field. Within the function, insert an if-else structure that tests the following: If no value has been entered for the expiration date, set the custom validation message to Enter the expiration date. If the expiration date does not match the regular expression pattern:/^(0[1-9]|1[0-2])\/20[12]\d$/set the custom validation message to Enter a valid expiration date. (Hint: Use the test() method.) Otherwise set the custom validation message to an empty text string. The remaining functions have already been entered for you. java------------------------------------------- "use strict"; /* New Perspectives on HTML5, CSS3, and JavaScript 6th Edition Tutorial 13 Review Assignment Credit Card Form Script Author: Date: Filename: co_credit.js Function List ============= runSubmit() Runs validation tests when the submit button is clicked validateCVC() Validates the credit card CVC number validateDate() Validates that the user has entered a valid expiration date for the credit card validateYear() Validates that the user has selected the expiration year of the credit card validateNumber() Validates that the user has entered a valid and legitimate card number validateCredit() Validates that the user has selected a credit card type validateName() Validates that the user has specified the name on the credit card sumDigits(numStr) Sums the digits characters in a text string luhn(idNum) Returns true of idNum satisfies the Luhn Algorithm */ function runSubmit() { } function validateDate() { } function validateName() { } function validateCredit() { } function validateNumber() { } function validateCVC() { } function sumDigits(numStr) { } function luhn(idNum) { var string1 = ""; var string2 = ""; // Retrieve the odd-numbered digits for (var i = idNum.length - 1; i >= 0; i-= 2) { string1 += idNum.charAt(i); } // Retrieve the even-numbered digits and double them for (var i = idNum.length - 2; i >= 0; i-= 2) { string2 += 2*idNum.charAt(i); } // Return whether the sum of the digits is divisible by 10 return sumDigits(string1 + string2)
Event Listeners
Go to the co_credit.js file in your editor. Create an event listener for the window load event that retrieves the field values attached to the query string of the page’s URL. Add the following to the event listener’s anonymous function:
- Create the orderData variable that stores the query string text from the URL. Slice the orderData text string to remove the first ? character, replace every occurrence of the + character with a blank space, and decode the URI-encoded characters.
- Split the orderData variable at every occurrence of a & or = character and store the substrings in the orderFields array variable.
- Write the following values from the orderFields array into the indicated fields of the order form:
- orderFields[3] into the modelName field
- orderFields[5] into the modelQty field
- orderFields[7] into the orderCost field
- orderFields[9] into the shippingType field
- orderFields[13] into the shippingCost field
- orderFields[15] into the subTotal field
- orderFields[17] into the salesTax field
- orderFields[19] into the cartTotal field
Add another event listener for the window load event that runs different validation event handlers when the page is loaded by the browser. Add the following code to the anonymous function for load event:
- Run the runSubmit() function when the subButton is clicked.
- Run the validateName() function when a value is input into the cardHolder field.
- Run the validateNumber() function when a value is input into the cardNumber field.
- Run the validateDate() function when a value is input into the expDate field.
- Run the validateCVC() function when a value is input into the cvc field.
JavaScript Functions
Create the runSubmit() function that is run when the form is submitted. Within the function add commands to run the validateName(), validateCredit(), validateNumber(), validateDate(), and validateCVC() functions.
Create the validateDate() function. The purpose of this function is to validate the credit card expiration date stored in the expDate field. Within the function, insert an if-else structure that tests the following:
- If no value has been entered for the expiration date, set the custom validation message to Enter the expiration date.
- If the expiration date does not match the regular expression pattern:/^(0[1-9]|1[0-2])\/20[12]\d$/set the custom validation message to Enter a valid expiration date. (Hint: Use the test() method.)
- Otherwise set the custom validation message to an empty text string.
The remaining functions have already been entered for you.
java-------------------------------------------

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

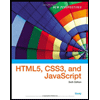
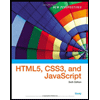