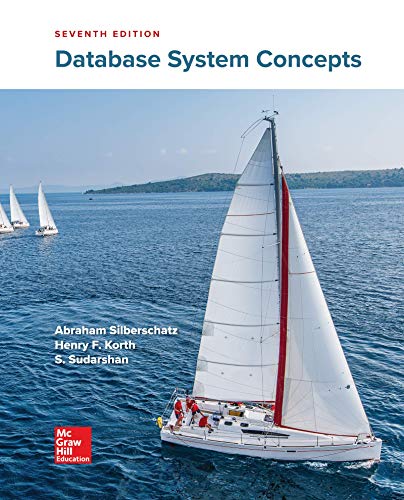
Concept explainers
For this
Carefully read the instructions and write a program that reads the following
information and prints a payroll statement.
Employee’s name (e.g., Smith)
Number of hours worked in a week (e.g., 10)
Hourly pay rate (e.g., 9.75)
Federal tax withholding rate (e.g., 20%)
State tax withholding rate (e.g., 9%)
design a program to
• Prompt user for 5 values and read the values using Scanner
o Use method .nextLine() to get the String for the name
o Use method .nextDouble() to get all other numeric values
• Calculating the Gross pay
o Gross pay = hours worked * hourly pay rate
• Calculating the Federal withholding
o Federal withholding = Gross pay * federal tax withholding rate
• Calculating the State withholding
o State withholding = Gross pay * state tax withholding rate
• Calculating the Total deduction
o Total deduction = Federal withholding + State withholding
• Calculating the Net Pay
o Net Pay = Gross pay – Total deduction
• Formatting the output same as the Sample run (Use ONLY printf)
o Correctly format 2 decimals and use $ (hint $%.2f)
o Indenting the under Deductions (hint \t)
o Printing % for state withholding and federal withholding rate (hint refer to
practice question below)
Include a comment at the start of the program with the following.
The computer must do all calculations.
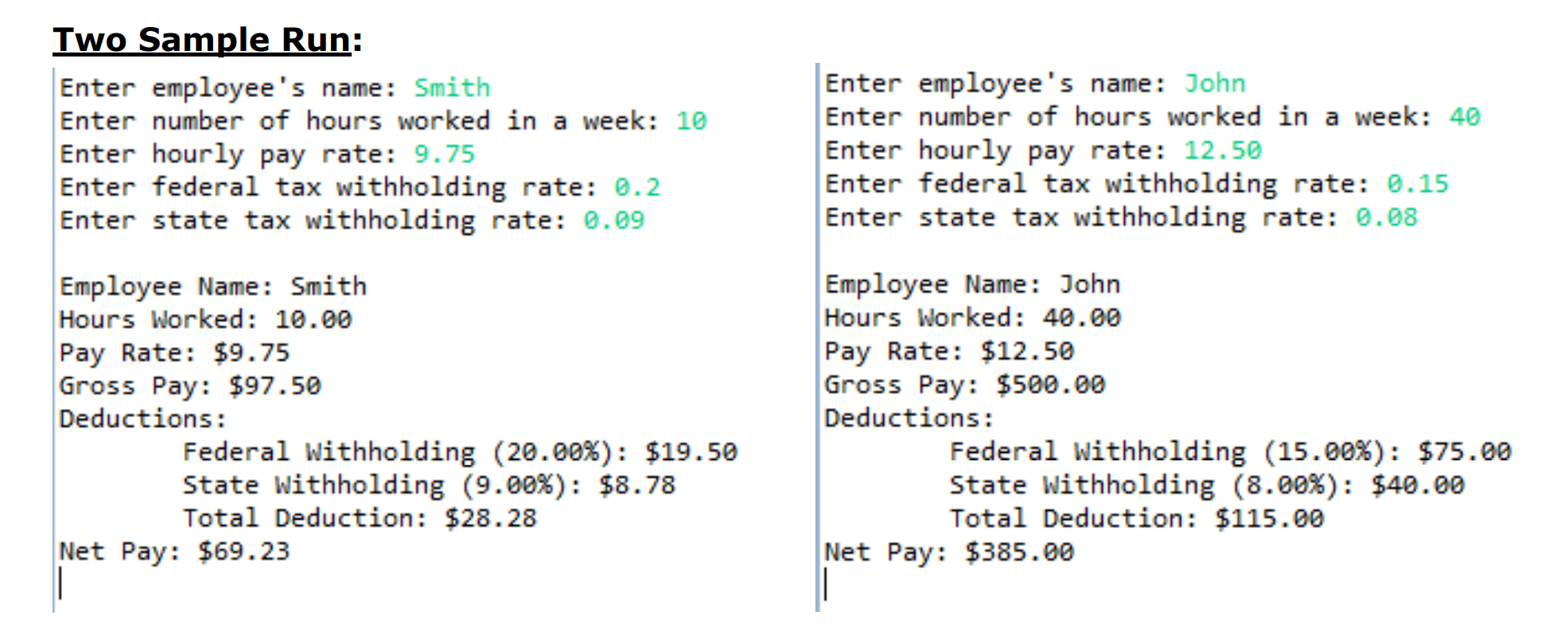

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- 4. Step through each line of the JavaScript program below. (This is the program from Figure 17.1.) Write down the value of each variable as you walk through it. Use an order of a 16 oz. latte with 2 shot. var price; var taxRate = 0.088; if (drink == "espresso") price = 1.40; if (drink == "latte" || drink == "cappuccino") { if (ounce == 8) price = 1.95; if (ounce == 12) price = 2.35; if (ounce == 16) price = 2.75; } if (drink == "Americano") price = 1.20 + .30 * (ounce/8); price = price + (shots - 1) * .50; price = price + price * taxRatearrow_forwardPhyton: A person can obtain their driver's license if they are of legal age and have official identification. Write a Python program that reads a person's age and if they have (y/n) official identification. Output must show Yes if you can obtain your license or No if you cannot obtain it Entry person's age The letter s or n indicates whether or not you have official identification. The letter is lowercase. Exit The word Yes or No depending on whether or not the person can get their driver's license. Note that the word goes with the first letter capitalized. Program execution example: >>>21 >>>s Yesarrow_forwardJAVA based program need helparrow_forward
- Note: write a Jave Progame below Question2: Suppose a retail business sells an item that is regularly priced at $98 and is planning to have a sale where the item s price will he reduced by 25 percent. You have been asked to write a program to calculate the sale price of the item. To determine the sale price you perform two calculations: you get the amount of the discount, which is 25 percent of the item's regular price. you subtract the discount amount from the item's regular price. This gives you the sale price.arrow_forwardScenario: You have been tasked with writing a program that reads the total price of the items brought into the hypermarket and calculates the value-added tax applied to the purchase based on the following rules. If the total price is between 0 and 100, no tax will be applied. Otherwise, if the total price is between 101 and 200, the program will apply a tax of 2% of the total price. Furthermore, if the total price is between 200 and 300, the tax is 5% of the total price. Finally, if the total price is greater than 300, the tax is 10% of the total price. hellp ):arrow_forwardPython Upvote for VERY QUICK answer. No need to comment the code. Thank you.arrow_forward
- Write a program in Python that reads the following information and prints a payroll statement: Employee’s name (e.g., Smith) Number of hours worked in a week (e.g., 10) Hourly pay rate (e.g., 9.75) Federal tax withholding rate (e.g., 20%) State tax withholding rate (e.g., 9%) A sample run is shown below: Enter employee's name: Smith Enter number of hours worked in a week: 10 Enter hourly pay rate: 9.75 Enter federal tax withholding rate: 0.20 Enter state tax withholding rate: 0.09 Employee Name: Smith Hours Worked: 10.0 Pay Rate: $9.75 Gross Pay: $97.5 Deductions: Federal Withholding (20.0%): $19.5 State Withholding (9.0%): $8.77 Total Deduction: $28.27 Net Pay: $69.22arrow_forwardC#arrow_forwardJava:arrow_forward
- The CarMin dealership has approached you to write a program to keep track of the sales commissions paid to the sales team each time they sell a used car. The amount of the commission that a salesperson earns is based on the type of vehicle sold. Currently CarMin is trying to incentivize it salesforce to try and sell Hybrids and SUVs because these vehicles generate the most profit within the dealership. vehicle types have the highest commissions. These Vehicle Type Bonus Commission Hybrid. 4.0% of Selling Price Coupe. 1.5% of Selling Price Sedan. 1.5% of Selling Price SUV 3.0% of Selling Price Minivan 2.5% of Selling Price Create a program for use by the sales manager to track commissions by vehicle type. It is unknown how many cars the dealership will sell in a given month. The program should allow the manager to continuously enter a vehicle type and selling price each time a vehicle is sold. You must validate the vehicle type and selling price using appropriate validation.…arrow_forwardASSIGNMENT: You are working for a lumber company, and your employer would like a program that calculates the cost of lumber for an order. The company sells pine, fir, cedar, maple, and oak lumber. Lumber is priced by board feet. One board foot equals one square foot that is one inch thick. The price per board foot is given in the following table: Pine 0.89 Fir 1.09 Cedar 2.26 Maple 4.50 Oak 3.10 The lumber is sold in different dimensions (specified in inches of width and height, and feet of length) that need to be converted to board feet. For example, a 2 x 4 x 8 piece is 2 inches wide, 4inches high, and 8 feet long, and is equivalent to 5.333 board feet (2 * 4 * 8 = 64, which when divided by 12 = 5.333 board feet). An entry from the user will be in the form of a letter and four integer numbers. The integers are the number of pieces, width, height, and length. The letter will be one of P, F, C, M, O (corresponding to the five kinds of wood) or T, meaning total. When the letter is T,…arrow_forwardI need a Pseudocode for this, please: # Rebecca Oyewole Project 4 This program calculates a customer receipt # showing each item ordered, the quantity,the price per item, the # amount owed for each item, with a total at the end. def get_order(): item = input() if not item: return '00' if item == 'Q': return 'QQ' quantity: int = int(input('How many? ')) return item, quantity register = 0 order = '' prices = 50, 60, 70, 75, 90 print('Welcome to the Lemonade Truck...') print('Ready to take orders!') while 1: print('What would you like to order? (1-3)? One at a time, please.') print('1. Glass of lemonade - $0.50\n' '2. Glass of orange juice - $0.60\n' '3. Glass of grapefruit juice - $0.70\n' '4. Glass of mango juice - $0.75\n' '5. Glass of water - $0.90') item, quantity = get_order() if item == 'Q': break if item == '0': quantities = list(order.count(obj) for obj in '123') for name, quantity…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
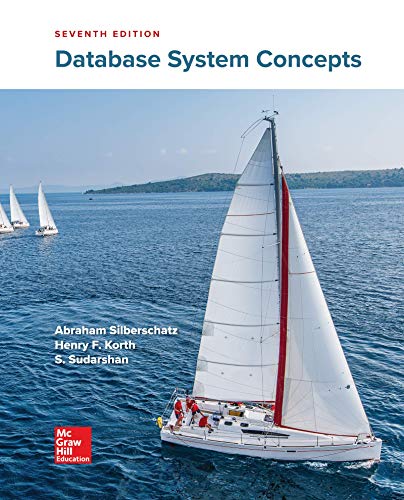
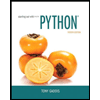
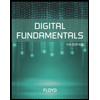
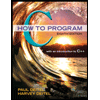
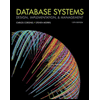
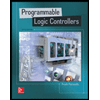