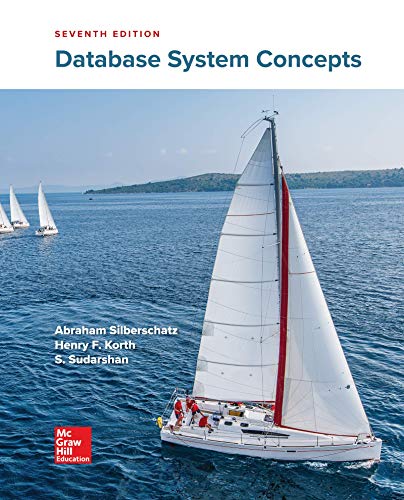
#SumCalculator Class:
-
Create a class named SumCalculator that extends the Thread class. This class calculates the sum of numbers within a provided range.
-
Declare three instance variables: start, end, and sum in the SumCalculator class . start and end are the lower and upper bounds of the range, respectively, while sum stores the sum of the numbers within the range.
-
Create a constructor that takes in two parameters: start and end, and initializes the corresponding instance variables. The sum variable should be initialized to 0.
-
Define the run method to calculate the sum of the numbers within the range. Inside of the run method use a for-loop to iterate over the numbers within the range and add each number to the sum variable.
-
Define the accessor method getSum that returns the value of the sum variable.
#Main Class:
-
Create a class named Main that contains the main method, the entry point of the program.
-
Create two objects of the SumCalculator class , with the first object calculating the sum of the numbers from 1 to 50, and the second object calculating the sum of the numbers from 51 to 100.
-
Call the start method on both instances of the SumCalculator class to start the calculation of the sum in separate threads.
-
Call the join method on both instances to wait for the calculation of the sum to complete in both threads.
-
Call the getSum method on both instances to get the sum of the numbers calculated in each thread. This should add the results to get the total sum.
-
Print the total sum to the console.
=======================================================================================

Step by stepSolved in 4 steps with 3 images

Try commenting out your thread code and summing all of the integers from 1 to 2000. How long does it take?
Now, uncomment that thread code and split your sums into the two portions,
How long does it take now?
What are some other ways you could improve this code? When the numbers got bigger, what did you notice? What surprised you?
Try commenting out your thread code and summing all of the integers from 1 to 2000. How long does it take?
Now, uncomment that thread code and split your sums into the two portions,
How long does it take now?
What are some other ways you could improve this code? When the numbers got bigger, what did you notice? What surprised you?
- Design a class called Post. This class models a StackOverflow post. It should have properties for title, description and the date/time it was created. We should be able to up-vote or down-vote a post. We should also be able to see the current vote value. In the main method, create a post, up-vote and down-vote it a few times and then display the the current vote value.arrow_forwardin visual c# (windows form app) no console or Console.WriteLine Design a non-static method that can be contained within an... Design a non-static method that can be contained within an instance/object of the MyCallback delegate type. This method should display values of two data fields contained within the object that is passed to the method. Make-up your own method definition that meets these requirements.arrow_forwardClass Circle A Circle will have a radius. The class will be able to keep track of the number of Circle objects created. It will also hold the total of the circumferences of all the Circle objects created. It will allow a client to create a Circle, passing in a double value for the radius into the Circle constructor. If the value for the radius that is passed in is 0 or negative, then the radius will be set to a value of 999. The constructor should add 1 to the count of the number of Circles created. The constructor should call a method to calculate the circumference and then add the circumference for that object to an accumulator. In addition to the constructor, the Circle class must have the following methods that return a boolean value: isCongruent(Circle c) – Compares two Circle objects to determine if they are congruent (radii are equal). isValid() – the radius may not be 0 or negative. equals (Triangle t) – compares two Triangle objects…arrow_forward
- #Testing Please test my code and see if it meet the follwing needs: The class should implement the Comparable interface. Circle one is less than Circle two if the radius of Circle one is less than the radius of Circle two. The two Circles are equal if they have the same radius. Circle one is larger than Circle two if its radius is larger. If circle One.compareTo circle Two <> 0 The Test Class displays a menu that allows the user to: Enter a Circle (the user only needs to enter the radius). Print all Circles (print the toString for each Circle in the ArrayList). Quit To adda a circle Cases: The ArrayList is empty The new circle is less than the first circle, add it at the beginning. The circle is greater than the last circle, add it at the end The new circle belongs somewhere in the middle. import java.lang.Math;public class Circle implements Comparable<Circle> {private double radius;public Circle(double radius) {this.radius = radius;}public double findArea()…arrow_forwardJAVA PPROGRAM ASAP Please Modify this program ASAP BECAUSE IT IS MY LAB ASSIGNMENT so it passes all the test cases. It does not pass the test cases when I upload it to Hypergrade. Because RIGHT NOW IT PASSES 0 OUT OF 1 TEST CASES. I have provided the failed the test cases and the inputs as a screenshot. The program must pass the test case when uploaded to Hypergrade. import java.util.Scanner;// creating class WordCounterpublic class WordCounter{ public static void main(String[] args) { // creating a scanner object Scanner sc = new Scanner(System.in); // if while loop is true while (true) { // then enter a string System.out.print("Please enter a string or type QUIT to exit:\n"); // Taking string as user input String str = sc.nextLine(); // if user enters quit then ignore the case if (str.equalsIgnoreCase("QUIT")) { break; } // Counting the…arrow_forwardWrite all the code within the main method in the TestTruck class below. Implement the following functionality. Construct two Truck objects: one with any make and model you choose and the second object with gas tank capacity 10. If an exception occurs, print the stack trace. Print both Truck objects that were constructed. import java.lang.IllegalArgumentException ; public class TestTruck { public static void main( String[] args ) { // write your code herearrow_forward
- Using javascript getElementByClass- write a simple programe whereby each time a player gets shot by a bullet, the player loses a life and a 'live' is remove from the three 'lives'.If the player loses all three lives, display and print 'game over'.Display 'play again' message to restart th game. <ul class = "lives"> <li></li> <li></li> <li></li> </ul>arrow_forward1. Goals and Overview The goal of this assignment is to practice creating a hash table. This assignment also has an alternative, described in Section 5. A hash table stores values into a table (meaning an array) at a position determined by a hash function. 2. Hash Function and Values Every Java object has a method int hashCode() which returns an int suitable for use in a hash table. Most classes use the hashCode method they inherit from object, but classes such as string define their own hashCode method, overriding the one in object. For this assignments, your hash table stores values of type public class HashKeyValue { K key; V value; } 3. Hash Table using Chained Hashing Create a hash table class (called HashTable211.java) satisfying the following interface: public interface HashInterface { void add(K key, V value); // always succeeds as long as there is enough memory V find (K key); // returns null if there is no such key } Internally, your hash table is defined as a private…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
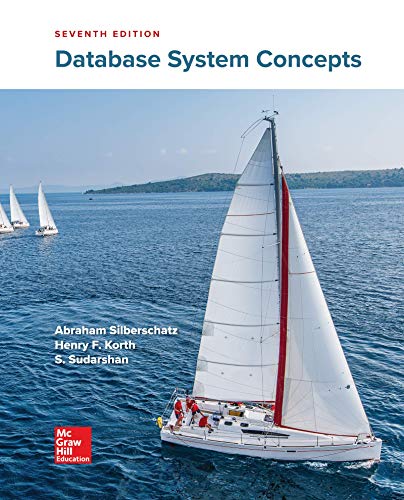
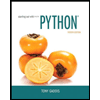
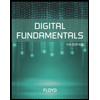
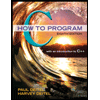
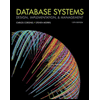
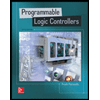