This may make more sense if I show you a sample run of a program that implements the wizard shown above: Do you want to buy a snowboard? no Do you want to buy downhill skis? yes Have you gone skiing before? yes Are you an expert? no Buy the ZR200 model. Notice how a series of questions is asked by the wizard and answered by the shopper. When there are no more questions to be asked, the conclusion is printed by the wizard and the program exits. The series of questions asked by the wizard closely follows the rules in the flow chart. A Decision object represents a triangle in the flow cart. The Decision object asks a question and then returns either the next decision in the flow chart, or a message that should be printed before the program terminates. This is an example of the Composite design pattern because a Decision object contains other Decision objects. Here is a description of the methods in the IDecision interface: public void setYes(IDecision yes): Specifies the IDecision that should follow this one if the user answers yes to the question. public void setNo(IDecision no): Specifies the IDecision that should follow this one if the user answers no to the question. public void setYesTerminal(String terminal): Specifies the conclusion that will be printed if the user answers yes to the question. public void setNoTerminal(String terminal): Specifies the conclusion that will be printed if the user answers no to the question. public IDecision ask(): This method asks the user the question that was specified when the Decision object was created (using a Scanner object). The return value specifies how we should proceed. If a Decision object is returned then it should be the next question asked. If null is returned, then a conclusion has been printed to the screen, and the line of questioning should stop. The Builder class in the UML diagram is responsible for building the composite of Decision objects. This class should be a singleton. The buildWizard method should construct a collection of interconnected decision objects that represent the flow chart. The first decision in the flow chart should be returned. Now, here are two sample runs that help illustrates how the wizard works: Input entered in the input box: yes yes no Expected output: Do you want to buy a snowboard? Have you snowboarded before? Are you an expert? Buy the XG200 model. Input entered in the input box: no yes yes no Expected output: Do you want to buy a snowboard? Do you want to buy downhill skis? Have you gone skiing before? Are you an expert? Buy the ZR200 model. public class Main { public static void main(String[] args) { IDecision next = Builder.getInstance().buildWizard(); do { next = next.ask(); } while (next != null); } } public interface IDecision { /** * Specifies the IDecision that should follow this one if the user answers yes * to the question. */ public void setYes(IDecision yes); /** * Specifies the IDecision that should follow this one if the user answers no * to the question. */ public void setNo(IDecision no); /** * Specifies the conclusion that will be printed if the user answers yes * to the question. */ public void setYesTerminal(String terminal); /** * Specifies the conclusion that will be printed if the user answers no * to the question. */ public void setNoTerminal(String terminal); /** * Asks the user the question that was specified when the Decision object * was created. The return value specifies how we should proceed. If a * Decision object is returned then it should be the next question asked. If * null is returned then a conclusion has been printed to the screen, and the * line of questioning should stop. */ public IDecision ask();
This may make more sense if I show you a sample run of a program that implements the wizard shown above:
Do you want to buy a snowboard?
no
Do you want to buy downhill skis?
yes
Have you gone skiing before?
yes
Are you an expert?
no
Buy the ZR200 model.
Notice how a series of questions is asked by the wizard and answered by the shopper. When there are no more questions to be asked, the conclusion is printed by the wizard and the program exits. The series of questions asked by the wizard closely follows the rules in the flow chart.
A Decision object represents a triangle in the flow cart. The Decision object asks a question and then returns either the next decision in the flow chart, or a message that should be printed before the program terminates. This is an example of the Composite design pattern because a Decision object contains other Decision objects. Here is a description of the methods in the IDecision interface:
- public void setYes(IDecision yes): Specifies the IDecision that should follow this one if the user answers yes to the question.
- public void setNo(IDecision no): Specifies the IDecision that should follow this one if the user answers no to the question.
- public void setYesTerminal(String terminal): Specifies the conclusion that will be printed if the user answers yes to the question.
- public void setNoTerminal(String terminal): Specifies the conclusion that will be printed if the user answers no to the question.
- public IDecision ask(): This method asks the user the question that was specified when the Decision object was created (using a Scanner object). The return value specifies how we should proceed. If a Decision object is returned then it should be the next question asked. If null is returned, then a conclusion has been printed to the screen, and the line of questioning should stop.
The Builder class in the UML diagram is responsible for building the composite of Decision objects. This class should be a singleton. The buildWizard method should construct a collection of interconnected decision objects that represent the flow chart. The first decision in the flow chart should be returned.
Now, here are two sample runs that help illustrates how the wizard works:
Input entered in the input box:
yes
yes
no
Expected output:
Do you want to buy a snowboard?
Have you snowboarded before?
Are you an expert?
Buy the XG200 model.
Input entered in the input box:
no
yes
yes
no
Expected output:
Do you want to buy a snowboard?
Do you want to buy downhill skis?
Have you gone skiing before?
Are you an expert?
Buy the ZR200 model.
public class Main
{
public static void main(String[] args)
{
IDecision next = Builder.getInstance().buildWizard();
do
{
next = next.ask();
} while (next != null);
}
}
public interface IDecision
{
/**
* Specifies the IDecision that should follow this one if the user answers yes
* to the question.
*/
public void setYes(IDecision yes);
/**
* Specifies the IDecision that should follow this one if the user answers no
* to the question.
*/
public void setNo(IDecision no);
/**
* Specifies the conclusion that will be printed if the user answers yes
* to the question.
*/
public void setYesTerminal(String terminal);
/**
* Specifies the conclusion that will be printed if the user answers no
* to the question.
*/
public void setNoTerminal(String terminal);
/**
* Asks the user the question that was specified when the Decision object
* was created. The return value specifies how we should proceed. If a
* Decision object is returned then it should be the next question asked. If
* null is returned then a conclusion has been printed to the screen, and the
* line of questioning should stop.
*/
public IDecision ask();
}



Step by step
Solved in 2 steps

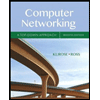
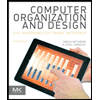
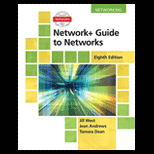
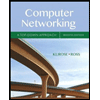
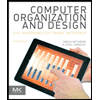
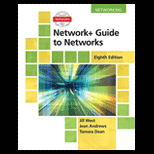
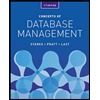
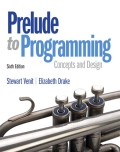
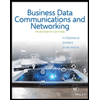