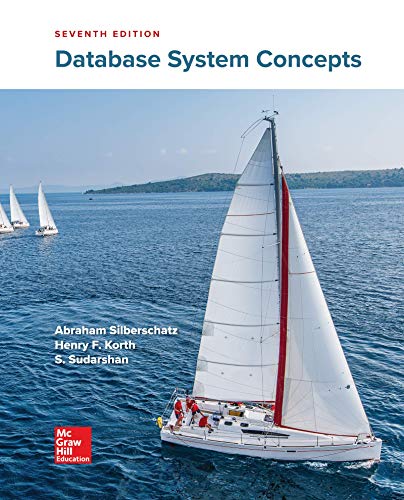
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
This is my code for a dictionary of student's test scores.
def main():
#Create a dictionary with name as key
#Marks as the list of values
student_dictionary = \
{
'Brian': [94, 89, 92],
'Rachel': [100,90,65],
'Jon': [67.5,95,100],
'Brit': [0,78,80] ,
'Gret': [65,100,78],
'Andrea': [55.5,67,79]
}
for key in student_dictionary.keys():
print('Name: ', key, " , Marks:", student_dictionary.get(key))
if __name__ == '__main__':
main()
Problem:
Find and print out Rachels second score (should be a 90)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Create a dictionary (order_dict) that the key is the burger number, and the value is a list of the quantities. For example: order_dict which is {'1': [10, 3, 78], '2': [35, 0, 65], '3': [9, 23, 0], '4': [0, 19, 43], '5': [43, 0, 21]} Create a dictionary (total_order_dict) that the key is the burger number, and the value is the total of the quantities for that burger. For example: total_order_dict which is {'1': 91, '2': 100, '3': 32, '4': 62, '5': 64} Use "assert to test the values of the total_order_dict For example: expected_result_ototal_order_dict = {'1': 91, '2': 100, '3': 32, '4': 62, '5': 64} assert actual_result_ototal_order_dict['1'] == expected_result_ototal_order_dict['1'] , "The actual result is not the \same as expected result for the order number 1!" (and test the other elements) The function can be like: def test_sum(): #Testing assert actual_result_ototal_order_dict['1'] == 91, "The actual result is not the \ same as expected result for the…arrow_forwardhello, this problem is give some trouble about it? my code # Dictionary to store course prerequisites course_prerequisites = { "CS111": "None", "CS141": "None", "CS151": "None", "CS211": "CS141, CS151", "CS251": "CS211", "CS261": "CS211", "CS277": "None", "CS377": "CS277", "CS341": "CS211, CS251, CS261", } # Print the header print("--------------------------------") print(" UIC CS Track") print("--------------------------------\n") # Ask the user for a course name course_name = input("Enter a subject abbreviation and course number (CS111 for example): ") # Check if the course name is in the dictionary if course_name in course_prerequisites: prerequisites = course_prerequisites[course_name] print(f"Prerequisites for {course_name}: {prerequisites}") else: print(f"Course {course_name} not found in the list of CS courses.") # Goodbye message print("\nThank you for using App!") print("Closing app...")arrow_forwardYou can create an empty dictionary with dictionary() [ ] ( ) { } This data structure stores a collection of objects in an unordered manner where each object must be unique dictionary set tuple listarrow_forward
- This program will store roster and rating information for a football team. Coaches rate players during tryouts to ensure a balanced team. (1) Prompt the user to input five pairs of numbers: A player's jersey number (0 - 99) and the player's rating (1 - 9). Store the jersey numbers and the ratings in a dictionary. Output the dictionary's elements with the jersey numbers in ascending order (i.e., output the roster from smallest to largest jersey number). Hint: Dictionary keys can be stored in a sorted list. (3 pts)Ex: Enter player 1's jersey number: 84 Enter player 1's rating: 7 Enter player 2's jersey number: 23 Enter player 2's rating: 4 Enter player 3's jersey number: 4 Enter player 3's rating: 5 Enter player 4's jersey number: 30 Enter player 4's rating: 2 Enter player 5's jersey number: 66 Enter player 5's rating: 9 ROSTER Jersey number: 4, Rating: 5 Jersey number: 23, Rating: 4 Jersey number 30, Rating: 2 ... (2) Implement a menu of options for a user to modify the roster. Each…arrow_forwardWrite code that prints out the keys that have a value less than 3 in the following dictionary: d= {'a':5, 'is':3, 'this':2, 'program':1}arrow_forwardCreate a flowchart for the following code. namesList=['Juan','Rae','Ivanna','Lilly','Robert']; #list to store names wagesHoursDict={7.50:35, 11.00:41, 18.25:26, 9.25:35, 11.10:45}; #dictionary to store wages and hours grossPay=0; #variable to store gross pay wagesList=list(wagesHoursDict.keys()); #convert all the keys of dictionary to listhoursList=list(wagesHoursDict.values()); #convert all the values of dictionary to list print("Name\tWages\tHours\tGross Pay"); #print header for i in range(0,5): if(hoursList[i]>40): #if hours more than 40 #calculate basic for 40 hours and 1.5 times basic for remaining hours grossPay=(wagesList[i]*40)+(1.5*wagesList[i]*(hoursList[i]-40)); else: grossPay=wagesList[i]*hoursList[i]; #calculate hours*wage print(namesList[i],"\t",wagesList[i],"\t",hoursList[i],"\t",grossPay); #printing all the valuesarrow_forward
- The get(key) function for a dictionary will return _____ if the key does not exist. In python. A. “ B. {} C. “Null” D. None arrow_forwardA date-to-event dictionary is a dictionary in which the keys are dates (as strings in the format 'YYYY-MM-DD'), and the values are lists of events that take place on that day. Complete the following function according to its docstring. Your code must not mutate the parameter! def group_by_year (events: Dict[str, List[str]]) -> Dict [str, Dict [str, List[str]]]: """Return a dictionary in which in the keys are the years that appear in the dates in events, and the values are the date-to-event dictionaries for all dates in that year. >>> date_to_event = {'2018-01-17': ['meeting', 'lunch'], '2018-03-15': ['lecture'], '2017-05-04': ['gym', 'dinner']} >>> group_by_year (date_to_event) {'2018': {'2018-01-17': ['meeting', 'lunch'], '2018-03-15': ['lecture']}, '2017': {'2017-05-04': ['gym', 'dinner']} } || || ||arrow_forwardBubble Sort HouseholdSize.py 2 HouseholdSize.py - This program uses a bubble sort to arrange household sizes Summary in descending order and then prints the mean and median 3 4 household size. In this lab, you will complete a Python program that uses a list to store data for the village of 5 Input: Interactive. Marengo. 6 Output: Mean and median household size. 8 The village of Marengo conducted a census and collected records that contain household 9 # Initialize variables. 10 householdSizes = [] # Array used to store household sizes. 11 numSizes = 0 data, including the number of occupants in each household. The exact number of household records has not yet been determined, but you know that Marengo has fewer than 300 households. 12 total = 0.0 13 mean = 0.0 The program is described in Chapter 8, Exercise 5, in Programming Logic and Design. The 14 median = 0.0 15 program should allow the user to enter each household size and determine the mean and 16 # Input household size 17…arrow_forward
- In pyhton Using the students dictionary provided below # This dictionary provided for youstudents = {'S1' : True,'S2' : False,'S3' : True,'S4' : False,} write a for loop that loops across the dictionary and collects all subject numbers (ex. 'S2') where the dictionary value is False. Imagine, for example, the dictionary indicates whether a student has completed an assignment, and we wanted to get a list of the students who had not yet completed the assignment. To answer this question, use a for loop across the students dictionary. You then need to get the associated value in each iteration, and check if it is True. If it is True, you can use continue to skip ahead to the next iteration. Otherwise, append the subject number (i.e. 'S2') to a list called incomplete.arrow_forward3. form_letter This function takes a list of dictionaries, where each dictionary has an entry of key "name" and entry of key "date". It returns a list of strings, where each output string has replaced the name and the date into the following template. Use the format method on strings -- see Lecture 8! The template should be exactly like this -- just copy-paste this into your code. TEMPLATE = """Dear {name},Your appointment is at {time}.Thanks very much.-- bimmy""" It can be either local to your function, or global in your Python file. Your choice! Sample calls should look like this. >>> form_letter([{"name":"Alex", "time":"three o'clock"},{"name":"Laura", "time":"the stroke of midnight"}])["Dear Alex,\nYour appointment is at three o'clock.\nThanks very much.\n-- bimmy", 'Dear Laura,\nYour appointment is at the stroke of midnight.\nThanks very much.\n-- bimmy']arrow_forwardA Dictionary object’s Remove method returns an integer indicating the number of elements that were removed. Question 48 options: True Falsearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
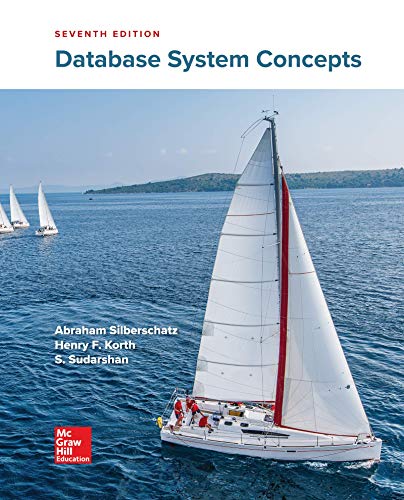
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
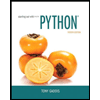
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
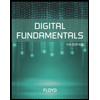
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
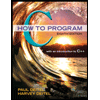
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
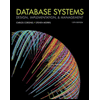
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
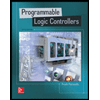
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education