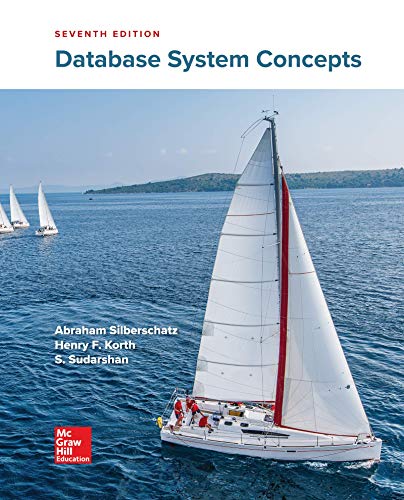
def level_up(leveling_factor, *args, **kwargs):
"""
Returns a dictionary with number of levels leveled up for each caster,
factoring in additional experience (if any).
Args:
leveling_factor: integer to divide total experience
*args: tuples containing name (str) and current experience (int)
**kwargs: additional experience for each spellcaster
Returns:
dictionary of each person's levels gained
>>> factor = 10
>>> level_up(factor, ("merlin", 350), ("hermione", 500), merlin = 100, \
hermione = 50)
{'merlin': 45, 'hermione': 55}
>>> factor = 50
>>> level_up(factor, ("merlin", 350), ("gandalf", 500), ("hermione", 250),\
("dumbledore", 700), merlin = 100, hermione = 50)
{'merlin': 9, 'gandalf': 10, 'hermione': 6, 'dumbledore': 14}
>>> factor = 30
>>> level_up(factor, ("harry", 200), ("ron", 150), ("hermione", 250), \
("neville", 175), harry = 50, neville = 30)
{'harry': 8, 'ron': 5, 'hermione': 8, 'neville': 6}
"""
spellcaster = {k:v for k,v in args}
experience = kwargs
return {k: (spellcaster.get(k, 0) + experience.get(k, 0)) for k in spellcaster.keys() | experience.keys()}
factor = 10
print(level_up(factor, ("merlin", 350), ("hermione", 500), merlin = 100, \
hermione = 50))

Step by stepSolved in 2 steps with 1 images

- Assuming that we have defined the Card class as above, fill in the blank in the code below such that the code segment prints out "Yes" without causing any errors. if > Card( 'Queen', 'Spades'): print("Yes")arrow_forwardPython code please help, indentation would be greatly appreciatedarrow_forwardCode the following directions in python. 1.Assume the variable dct references a dictionary. Write an if statement that determines whether the key 'Jim' exists in the dictionary. If so, delete 'Jim' and its associated value. 2.Write code to create a set with the following integers as members: 10, 20, 30, and 40. 3.Assume each of the variables set1 and set2 references a set. Write code that creates another set containing all the elements of set1 and set2, and assigns the resulting set to the variable set3.arrow_forward
- JS Write a function named number_of_pairs whose parameter is an object/dictionary with strings as keys and integers as values. Your function should returns the number of key-value pairs in the parameter.arrow_forwardProgram - Python Problem below the code This is my code for a dictionary of student's test scores. def main():#Create a dictionary with name as key#Marks as the list of valuesstudent_dictionary=\{'Brian': [94, 89, 92],'Rachel': [100,90,65],'Jon': [67.5,95,100],'Brit': [0,78,80] ,'Gret': [65,100,78],'Andrea': [55.5,67,79]}#Loop that print name and list of marksfor key, values in student_dictionary.items():print('Name: ', key, " , Marks:", values)#Call main functionif __name__ == '__main__':main() Problem: print the dictionary data using a loop that starts with for key inarrow_forwardThis code is a part of a C dictionary. Please write the code for the below requirements. dict_get Next, you will implement: char* dict_get (const dict_t* dict, const char* key); This function goes through the list given by dict. If you use the above structure, this means starting at el = dict->head and checking each time whether the key at el is key; if it is not, we set el = el->next, until either key is found, or we reach el == NULL. To compare key with el->key, we need to compare one by one each character in key with those in el->key. Remember that strings in C are just pointers (memory addresses) to the first character in the string, so comparing el->key == key will not do what you want. So how do you even get the length of a string s? You would start at memory location s and advance as long as the byte at s (i.e. *s) is not the end-of-string marker (\0, the NULL character). This can get a bit messy, so luckily, you are allowed to use the string comparison…arrow_forward
- Write code that iterates through a dictionary represented by the variable my_dict and prints any values (not keys!) associated with keys beginning with the letter "k" (lowercase).arrow_forwardMy code from milestone 1 # Define the quiz_type dictionary quiz_type = { 1: "BabyAnimals", 2: "Brooklyn99", 3: "Disney", 4: "Hogwarts", 5: "MyersBriggs", 6: "SesameStreet", 7: "StarWars", 8: "Vegetables" } # Print the welcome message print("Welcome to the Personality Quiz!") print() print("What type of Personality Quiz do you want to run?") print() for number, quiz_name in quiz_type.items(): print(f"{number} - {quiz_name}") print() test_number = int(input("Choose test number (1-8): ")) # Check if the test_number is valid if test_number in quiz_type: quiz_name = quiz_type[test_number] print() print(f"Great! Let's begin the {quiz_name} Personality Quiz...") else: print("Invalid test number. Please choose a number between 1 and 8.") ------------------------------------------------------------------- Milestone #3 code : # Ending statements with the period to easily split them as mentioned in the question. questions = [ "I…arrow_forwarddef city_dict(adict): """ Question 6 -Given a dictionary that maps a person to a list of countries they want to travel to, return a dictionary with the value being the list sorted by the last letter in each countries name. If two countries have the same last letter, sort by the first letter. -For an extra challenge, try doing this in one line (Optional). Args: adict (dict) Returns: dict >>> city_dict({"Pablo": ["Belgium", "Canada", "Germany"], "Athena": ["Italy", "France", "Egypt"], "Liv": ["Japan", "Bolivia", "Greece"]}) {'Pablo': ['Canada', 'Belgium', 'Germany'], 'Athena': ['France', 'Egypt', 'Italy'], 'Liv': ['Bolivia', 'Greece', 'Japan']} >>> city_dict({"Jacob": ["Bahamas", "Brazil", "Chile"], "Lexi": ["Colombia", "Finland", "Panama"], "Emily": ["Ireland", "Russia", "Kenya", "Jordan"]}) {'Jacob': ['Chile', 'Brazil', 'Bahamas'], 'Lexi': ['Colombia', 'Panama', 'Finland'], 'Emily': ['Kenya',…arrow_forward
- This section has been set as optional by your instructor. OBJECTIVE: Write a program in Python that prints the middle value of a dictionary's values, using test_dict from the test_data.py module. NOTE: test_dict is created & imported from the module test_data.py into main.py Example 1: test dict = {'a':1,'b':9,'c':2,'d':5} prints 5 Example 2: test_dict = {'b':9,'d':5} prints 5 Hint: You may(or may not) find the following function useful: list.sort() which sorts the elements in a list. Note: the result is automatically stored in the original list, and return value from the function is None Туре list = [1, 2,3,2,1] After calling: list = [1,1,2,2,3] list.sort(), 339336.2266020 x3zgy7 LAB 13.11.1: Middlest Value (Structured Types) АCTIVITY 0/11 File is marked as read only Current file: test_data.py 1 #This module creates the test_dict to be used in main.py 2 #Reads input values from the user & places them into test_dict 3 dict_size = int(input()) #Stores the desired size of test_dict. 4…arrow_forwardNeed help answering this python question Create a dictionary of your friends that you found in 2017 and 2018. So, the keys are '2017' and '2018. For example: friend_solar = {2022: ["James", "Linda"] , 2023: ["Joshua", "Zoey"]} Print the friend's name Ask the user to enter a name of their friends. Then show the friend you found in 2022 or 2023. Ask the user to add a new friend name to the 2022 or 2023 lists (You need also to ask the user what year they want to add the friend name). Then add the entered friend name to the dictionary(2022 or 2023 value) Print the friends' dictionary on the screen.arrow_forwardPythonarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
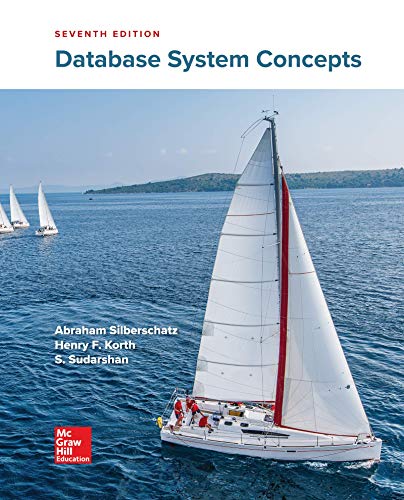
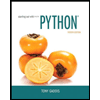
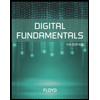
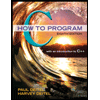
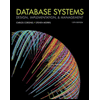
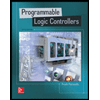