This exercise uses your programming environment to enhance the Web site you created last week with additional functionality to include images, tables and a Form using Python flask. Specifically, you will add two (2) additional routes allowing a user to register and login to a web site. Additional security considerations include other routes (beyond the register route) will not be accessible until a successful login
This exercise uses your programming environment to enhance the Web site you created last week with additional functionality to include images, tables and a Form using Python flask. Specifically, you will add two (2) additional routes allowing a user to register and login to a web site. Additional security considerations include other routes (beyond the register route) will not be accessible until a successful login has occurred
In addition to the requirements list above the following functionality should be found within your web site on one or more web pages.
A user registration form
A user login form
A password complexity should be enforced to include at least 12 characters in length, and include at least 1 uppercase character, 1 lowercase character, 1 number and 1 special character.
Below I have attached my python code as well as my home page html code. I need assistance creating the registration form and login form and implementing in both an html form and putting into my current python code. Thanks in advance.
app.py
"""Program to generate a simple website using Python flask.
Includes at least 3 routes using render_template() functionality."""
from datetime import date
from datetime import datetime
from flask import Flask, render_template
app = Flask(__name__,template_folder='templates')
now = datetime.now()
current_time = now.strftime("%H:%M")
@app.route('/')
@app.route('/home_page.html')
def home():
"""Render the home page for the website"""
return render_template('home_page.html', date = date.today(), time = current_time )
@app.route('/ariana_grande.html')
def page1():
"""Render the Ariana Grande page for the website"""
return render_template('ariana_grande.html', date = date.today(), time = current_time )
@app.route('/ava_max.html')
def page2():
"""Render the Ava Max page for the website"""
return render_template('ava_max.html', date = date.today(), time = current_time )
@app.route('/dua_lipa.html')
def page3():
""""Render the Dua Lipa page for the website"""
return render_template('dua_lipa.html', date = date.today(), time = current_time )
if __name__=='__main__':
app.run(debug=True)
home_page.html
<html>
<head>
<title>Home Page</title>
<link rel= "stylesheet" type= "text/css" href= "{{ url_for('static',filename='styles/style.css') }}">
</head>
<body>
<h1 style="color:red;">Home Page</h1>
<h2>Our Favorite Artists</h2>
<div class="separate">
<!--Ordered list-->
<ol>
<li>Ariana Grande</li>
<li>Ava Max</li>
<li>Dua Lipa</li>
</ol>
</div>
<h2>What you'll find on their page:</h2>
<div class="separate">
<!--Unordered list-->
<ul>
<li>Artist names and age</li>
<li>Fun facts about the group/artist</li>
<li>Links to their official page, Wikipedia, and social media page</li>
</ul>
</div>
<!--Display the date and time passed from python-->
<p>You visited this page on {{date}} at {{time}}</p>
<br>
<h3>Menu</h3>
<!--Menu to navigate to different pages-->
<a href="/ariana_grande.html">Ariana Grande</a>
<br>
<a href="/ava_max.html">Ava Max</a>
<br>
<a href="/dua_lipa.html">Dua Lipa</a>
</body>
</html>

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

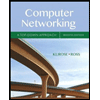
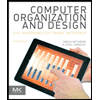
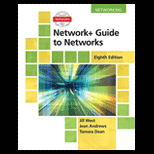
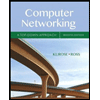
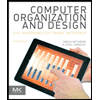
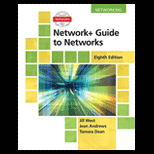
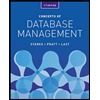
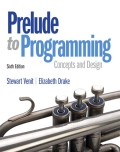
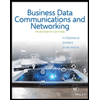