This exercise requires to ensure that the input values representing fractions are stored with denominators that are positive integers. You cannot require the user to only enter a positive denominator value; the user should not be inconvenienced by such a restriction. For example, whilst values of 1 / -2 are acceptable inputs for a fraction, the output representation should be -1 / 2. Your solution should check the denominator input; if it is negative, swap the sign of both numerator and denominator instance variables. Test this functionality with TestFraction.java class from the following program
This exercise requires to ensure that the input values representing
fractions are stored with denominators that are positive integers. You cannot
require the user to only enter a positive denominator value; the user should not
be inconvenienced by such a restriction. For example, whilst values of 1 / -2
are acceptable inputs for a fraction, the output representation should be -1 / 2.
Your solution should check the denominator input; if it is negative, swap the
sign of both numerator and denominator instance variables. Test this
functionality with TestFraction.java class from the following program
//Fraction class
class Fraction {
//Fraction numerator
int numerator;
//Fraction denominator
int denominator;
//method to initialize numerator and denominator
public void input(int num, int den)
{
numerator = num;
//check if denominator is not zero
if (den != 0) {
denominator = den;
}
else {
System.out.println("Invalid denominator!!");
System.exit(0);
}
}
//method to display fraction
public void display()
{
System.out.println(numerator + "/" + denominator);
}
}
import java.util.Scanner;
//test program
public class TestFraction
{
public static void main(String[] args) {
//create a Scanner object
Scanner in = new Scanner(System.in);
//create new fraction
Fraction frac = new Fraction();
//declare numerator and denominator
int n=1,d;
System.out.print("Enter Fraction's Numerator: ");
//input numerator
n = in.nextInt();
System.out.print("Enter Fraction's Denominator: ");
//input denominator
d = in.nextInt();
//loop execute until the numerator is negative
while(n>=0)
{
//call input with n and d
frac.input(n, d);
//call display
frac.display();
System.out.print("Enter Fraction's Numerator: ");
//input numerator
n = in.nextInt();
System.out.print("Enter Fraction's Denominator: ");
//input denominator
d = in.nextInt();
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

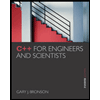
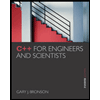