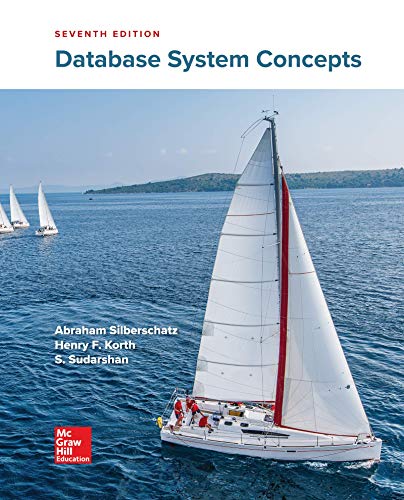
This exercise is about the bit-wise operators in C. Complete each function
skeleton using only straight-line code (i.e., no loops, conditionals, or function
calls) and limited of C arithmetic and logical C operators. Specifically, you
are only allowed to use the following eight operators: ! ∼, &,ˆ|, + <<>>.
For more details on the Bit-Level Integer Coding Rules on p. 128/129 of the
text. A specific problem may restrict the list further: For example, to write
a function to compute the bitwise xor of x and y, only using & |, ∼
int bitXor(int x, int y)
{ return ((x&∼y) | (∼x & y));}
(a) /*
copyLSbit:Set all bits of result to least significant bit of x
* Example: copyLSB(5) = 0xFFFFFFFF, copyLSB(6) = 0x00000000
* Legal ops: ! ∼ & ˆ| + <<>>
*/
int copyLSbit(int x) {
return 2;}
(b) /* negate - return -x
* Example: negate(1) = -1.
* Legal ops:! ∼ & ˆ| + <<>>
*/ int negate(int x) { return 2; }
(c) /* isEqual - return 1 if x == y, and 0 otherwise
* Examples: isEqual(5,5) = 1, isEqual(4,5) = 0
* Legal ops: ! ∼ & ˆ| + <<>>
*/ int isEqual(int x, int y) { return 2; }
(d) /* twoCmax: return maximum two’s complement integer
Legal ops: ! ∼ & ˆ| + <<>>int twoCmax(void)
{ return 2; }
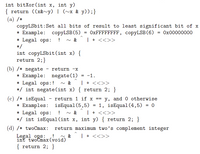

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- In c++, please. Thank you! Given a main() that reads user IDs (until -1), complete the BubbleSort() functions to sort the IDs in ascending order using the Bubblesort algorithm, and output the sorted IDs one per line. You may assume there will be no more than 100 user IDs. Ex. If the input is: kaylasimms julia myron1994 kaylajones -1 the output is: julia kaylajones kaylasimms myron1994 The following code is given: #include <string>#include <iostream> using namespace std; // TODO: Write the Bubblesort algorithm that sorts the array of string, with k elementsvoid Bubblesort(string userIDs [ ], int k) { } int main() { string userIDList[100]; string userID; cin >> userID; while (userID != "-1") { //put userID in the array cin >> userID; } // Initial call to quicksort Bubblesort(userIDList, /* ?? */ ); //make this output only the userIDs that were entered, not garbage for (int i = 0; i < 100; ++i) { cout <<…arrow_forwardDefine a function named get_encrypted_list (word) which takes a word as a parameter. The function returns a list of characters. The first element is the first letter from the parameter word and the rest is as a sequence of '*', each '*' representing a letter in the parameter word. Note: you can assume that the parameter word is not empty. For example: Test Result ['h', '*', **1 guess = get_encrypted_list('hello') print(guess) print (type (guess)) guess = get_encrypted_list('succeed') ['s', '*', print (guess) **¹, ¹*¹] **']arrow_forwardI need this code in C programing(Not C++) There are several test cases. Each test case begins with a line containing a single integer n (1≤n≤1000). Each of the next n lines is either a type-1 command, or an integer 2 followed by an integer x. This means that executing the type-2 command returned the element x. The value of x is always a positive integer not larger than 100. Given a sequence of operations with return values, you’re going to guess the data structure. It is a stack (Last-In, First-Out), a queue (First-In, First-Out), a priority-queue (Always take out larger elements first), not sure(It can be more than one of the three data structures mentioned above) or impossible(It can’t be a stack, a queue or a priority queue). Sample Input Sample output 6 1 1 1 2 1 3 2 1 2 2 2 3 6 1 1 1 2 1 3 2 3 2 2 2 1 2 1 1 2 2 4 1 2 1 1 2 1 2 2 7 1 2 1 5 1 1 1 3 2 5 1 4 2 4 1 2 1 queue not sure impossible stack priority queue impossiblearrow_forward
- Define a function named get_encrypted_list (word) which takes a word as a parameter. The function returns a list of characters. The first element is the first letter from the parameter word and the rest is as a sequence of '*', each '*' representing a letter in the parameter word. Note: you can assume that the parameter word is not empty. For example: Test Result ['h', ¹*¹ **', '*'] *** guess = get_encrypted_list('hello') print (guess) print (type (guess)) guess = get_encrypted_list('succeed') ['s', '*', print (guess) **']arrow_forwardPython Programmingarrow_forwardImplement the following C function: repeatChars take in a null-terminated string as an argument and returns a newly- allocated null-terminated string that repeats every character in the input string. For example, repeatChars(''abcd'' )=''aabbccdd'' . You may use the strlen function to determine the length of the string your implementation does not need to check whether malloc returns NULL C Your implementation should not modify the input stringarrow_forward
- Computer Science code in c++ please .Do NOT use ANY string/character manipulation or arithmetic functions to implement your solution. You may assume that the input to this encryption function is always exactly 16 bits long. Test that input 0xADE1 yields ciphertext (188, 153). You may hard-code these values for testing.arrow_forwardplease code in python Implement an encryption function that tests that input 0xADE1 yields ciphertext (188, 153). You may hard-code these values for testing. Do NOT use ANY string/character manipulation or arithmetic functions to implement your solution.arrow_forwardConsider the following two sentences: S1:I like yellow roses better than red ones. S2:Looks like John is bettering the working conditions at his organization Create a Python function that encodes two sentences using the custom BPE tokenizer and identifies common subword tokens (tokens that appear in both encodings). Return a list of these common subword tokens. Is/Are there any interesting observations when you compare the tokens between the two encodings? What do you think is causing what you observe as part of your comparison?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
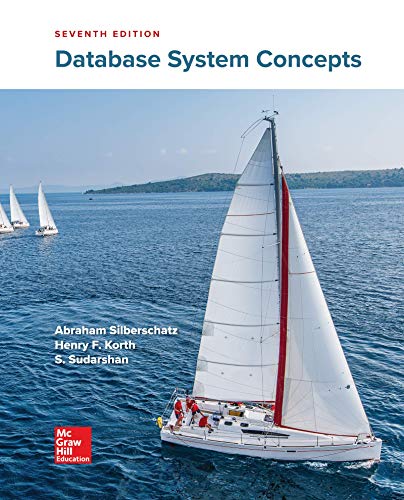
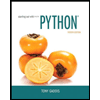
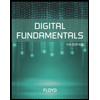
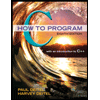
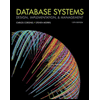
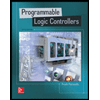