In c++, please. Thank you! Given a main() that reads user IDs (until -1), complete the BubbleSort() functions to sort the IDs in ascending order using the Bubblesort algorithm, and output the sorted IDs one per line. You may assume there will be no more than 100 user IDs. Ex. If the input is: kaylasimms julia myron1994 kaylajones -1 the output is: julia kaylajones kaylasimms myron1994 The following code is given: #include #include using namespace std; // TODO: Write the Bubblesort algorithm that sorts the array of string, with k elements void Bubblesort(string userIDs [ ], int k) { } int main() { string userIDList[100]; string userID; cin >> userID; while (userID != "-1") { //put userID in the array cin >> userID; } // Initial call to quicksort Bubblesort(userIDList, /* ?? */ ); //make this output only the userIDs that were entered, not garbage for (int i = 0; i < 100; ++i) { cout << userIDList[i] << endl;; } return 0; }
In c++, please. Thank you!
Given a main() that reads user IDs (until -1), complete the BubbleSort() functions to sort the IDs in ascending order using the Bubblesort
Ex. If the input is:
kaylasimms julia myron1994 kaylajones -1
the output is:
julia kaylajones kaylasimms myron1994
The following code is given:
#include <string>
#include <iostream>
using namespace std;
// TODO: Write the Bubblesort algorithm that sorts the array of string, with k elements
void Bubblesort(string userIDs [ ], int k) {
}
int main() {
string userIDList[100];
string userID;
cin >> userID;
while (userID != "-1") {
//put userID in the array
cin >> userID;
}
// Initial call to quicksort
Bubblesort(userIDList, /* ?? */ );
//make this output only the userIDs that were entered, not garbage
for (int i = 0; i < 100; ++i) {
cout << userIDList[i] << endl;;
}
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

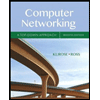
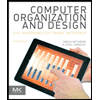
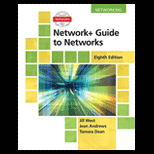
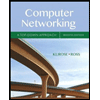
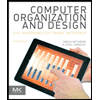
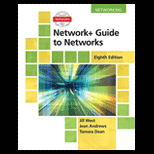
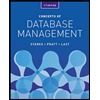
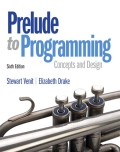
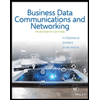