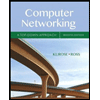
This code is in Ada programming language
Please type your solution out in working code don't just give me an example they may just confuse me more
This code is what I have so far what is in the image is what I'm currently trying to do And asking help with
Below is Number_Theory.adb
with Ada.Numerics.Generic_Elementary_Functions;
package body Number_Theory is
-- Instantiate the library for floating point math using Floating_Type.
package Floating_Functions is new Ada.Numerics.Generic_Elementary_Functions(Floating_Type);
use Floating_Functions;
function Factorial(N : in Factorial_Argument_Type) return Positive is
begin
-- TODO: Finish me!
--
-- 0! is 1
-- N! is N * (N-1) * (N-2) * ... * 1
return 1;
end Factorial;
function Is_Prime(N : in Prime_Argument_Type) return Boolean is
Upper_Bound : Positive;
Current_Divisor : Prime_Argument_Type;
begin
-- Handle 2 as a special case.
if N = 2 then
return True;
end if;
Upper_Bound := N - 1;
Current_Divisor := 2;
while Current_Divisor < Upper_Bound loop
if N rem Current_Divisor = 0 then
return False;
end if;
Upper_Bound := N / Current_Divisor;
Current_Divisor := Current_Divisor + 1;
end loop;
return True;
end Is_Prime;
function Prime_Counting(N : in Prime_Argument_Type) return Natural is
begin
-- TODO: Finish me!
--
-- See the page for more information.
return 0;
end Prime_Counting;
function Logarithmic_Integral(N : in Prime_Argument_Type) return Floating_Type is
begin
-- TODO: Finish me!
--
-- See the page for more information.
return 1.0;
end Logarithmic_Integral;
end Number_Theory;
Below is Number_Theory.ads
-- This package contains subprograms for doing number theoretic computations.
package Number_Theory is
-- Constant Definitions
-----------------------
Gamma : constant := 0.57721_56649_01532_86060_65120_90082_40243_10421_59335_93992;
-- Type Definitions
-------------------
-- The range of values for which N! can be computed without overflow.
subtype Factorial_Argument_Type is Integer range 0 .. 12;
-- The range of values that might meaningfully be asked: are you prime?
subtype Prime_Argument_Type is Integer range 2 .. Integer'Last;
-- A floating point type with at least 15 significant decimal digits.
type Floating_Type is digits 15;
-- Subprogram Declarations
--------------------------
-- Returns N!
function Factorial(N : Factorial_Argument_Type) return Positive;
-- Returns True if N is prime; False otherwise.
function Is_Prime(N : in Prime_Argument_Type) return Boolean;
-- Returns the number of prime numbers less than or equal to N.
function Prime_Counting(N : in Prime_Argument_Type) return Natural;
-- The logarithmic integral function, which is an approximation of the prime counting function.
function Logarithmic_Integral(N : in Prime_Argument_Type) return Floating_Type;
end Number_Theory;
Below in main.adb
-- Some packages that we will need.
with Ada.Text_IO;
with Ada.Integer_Text_IO;
with Number_Theory;
-- Make the contents of the standard library packages "directly visible."
use Ada.Text_IO;
use Ada.Integer_Text_IO;
procedure Main is
-- We need to print values of type Number_Theory.Floating_Type, so generate a package for that.
package Floating_IO is new Ada.Text_IO.Float_IO(Number_Theory.Floating_Type);
use Floating_IO;
N : Number_Theory.Prime_Argument_Type;
-- Add any other local variables you might need here.
begin
Put_Line("Counting Primes!");
-- TODO: Finish me!
end Main;
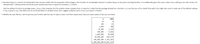

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps

- This code is in Ada code in Ada Please Not in C code Please type your solution out in working code don't just give me an example they may just confuse me more Below is Number_Theory.adb with Ada.Numerics.Generic_Elementary_Functions; package body Number_Theory is -- Instantiate the library for floating point math using Floating_Type. package Floating_Functions is new Ada.Numerics.Generic_Elementary_Functions(Floating_Type); use Floating_Functions; function Factorial(N : in Factorial_Argument_Type) return Positive is begin -- TODO: Finish me! -- -- 0! is 1 -- N! is N * (N-1) * (N-2) * ... * 1 return 1; end Factorial; function Is_Prime(N : in Prime_Argument_Type) return Boolean is Upper_Bound : Prime_Argument_Type; Current_Divisor : Prime_Argument_Type; begin -- Handle 2 as a special case. if N = 2 then return True; end if; Upper_Bound := N - 1; Current_Divisor…arrow_forwardcomputer graphics A Simple Utility for Drawing Function Curves i need write code c#(.net) and send the design please with outputlike this https://www.codeproject.com/Articles/5267642/A-Simple-Utility-for-Drawing-Function-Curvesthe design in picture form1button name button1 picturebox name picturebox1when i click the button draw cerve , static function and the curve like picturearrow_forwardPlease write the code in C language thank youarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
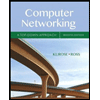
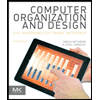
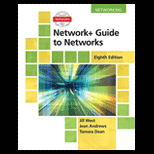
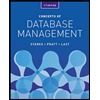
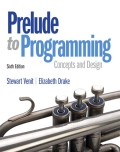
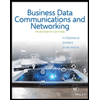