Write a for loop to print all NUM_VALS elements of vector courseGrades, following each with a space (including the last). Print forwards, then backwards. End with newline. Ex: If courseGrades = {7, 9, 11, 10}, print: 7 9 11 10 10 11 9 7 Hint: Use two for loops. Second loop starts with i = courseGrades.size() - 1 (Notes) C++ without using std::
Write a for loop to print all NUM_VALS elements of
7 9 11 10 10 11 9 7 Hint: Use two for loops. Second loop starts with i = courseGrades.size() - 1 (Notes)
C++
without using std::
Note: These activities may test code with different test values. This activity will perform two tests, both with a 4-element vector (vector<int> courseGrades(4)). See "How to Use zyBooks".
Also note: If the submitted code has errors, the test may generate strange results. Or the test may crash and report "Program end never reached", in which case the system doesn't print the test case that caused the reported message.
#include <iostream>
#include <vector>
using namespace std;
int main() {
const int NUM_VALS = 4;
vector<int> courseGrades(NUM_VALS);
int i;
for (i = 0; i < courseGrades.size(); ++i) {
cin >> courseGrades.at(i);
}
if (courseGrades.size()
for (i = 0; i < courseGrades.size() -1; --i) {
cin >> courseGrades.at(i);
cout << NUM_VALS << " ";
}
cout << endl;
return 0;
}
It's a mess and it only produces 4 in the output

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

this C++ code is pre-existing and cannot be changed or modified. for it to work, the program has to use this information.
#include <iostream>
#include <
using namespace std;
int main() {
const int NUM_VALS = 4;
vector<int> courseGrades(NUM_VALS);
int i;
for (i = 0; i < courseGrades.size(); ++i) {
cin >> courseGrades.at(i);
}
//enter code here//
return 0;
}
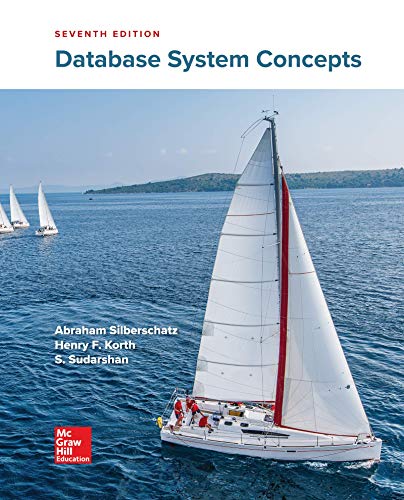
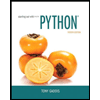
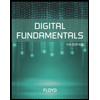
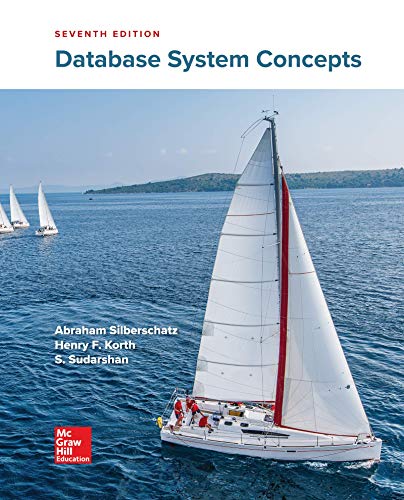
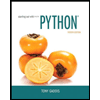
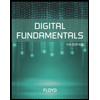
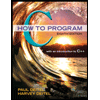
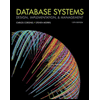
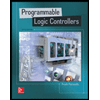