This assignment relates to content from Chapter 13 and Exercise #11 of the eText. The objective is to have the program know when numbers out of the array boundaries are received and mitigate those problems. The given code intentionally induces numbers out of bounds and the student's portion must correct for this. As always use a header file and implementation file to do those calculations. Remember not to change the given code below in any way. Instructors are encouraged to paste this default code over your source and see if your program still runs. INSTRUCTIONS You will not complete this assignment in Mindtap. This assignment will be completed in Visual Studio and submitted to the associated Assignment Folder. To complete your assignment, ZIP and submit the entire project folder with *.sln project file inside. 1. Download the supplied code. Do not change anything in the supplied code which is the Ch13_Ex11_Main Program.cpp (except to add documentation and your name). Please use the file names listed below since your file will have the same components in the supplied code. 2. Recall that in C++, there is no check on an array index out of bounds. However, during program execution, an array index out of bounds can cause serious problems. Also, in C++, the array index starts at 0. 3. Design and implement the class myArray that solves the array index out of bounds problem and also allows the user to begin the array index starting at any integer, positive or negative. • Every object of type myArray is an array of type int. During execution, when accessing an array component, if the index is out of bounds, the program must terminate with an appropriate error message. Consider the following statements: myArray list(5); //Line 1 myArray myList(2, 13); //Line 2 myArray yourList(-5, 9); //Line 3 The statement in Line 1 declares list to be an array of 5 components, the component type is int, and the components are:list[0], list[1], ..., list[4]; The statement in Line 2 declares myList to be an array of 11 components, the component type is int, and the components are: myList[2], myList[3],..., myList[12]; The statement in Line 3 declares yourList to be an array of 14 components, the component type is int, and the components are: yourList[-5], yourList[-4], ..., yourList[0], ..., yourList[8].
Supplied code Document
/ Ch13_Ex11_MainProgram.cpp - given code below
// Ch13_Ex11_MainProgram.cpp - given code below // #include "stdafx.h" may uncomment this if Visual Studios requires
#include <iostream>
#include "myArray.h"
using namespace std;
int main()
{
// Creates list1 and list2
myArray list1(5);
myArray list2(5);
// Zeroing (initializing) first array
int i;
cout << "list1 : ";
for (i = 0; i < 5; i++)
cout << list1[i] << " ";
cout << endl;
// Prompt to enter five numbers into list1
cout << "Enter 5 integers: ";
for (i = 0; i < 5; i++)
cin >> list1[i];
cout << endl;
// show contents list1
cout << "After filling list1: ";
for (i = 0; i < 5; i++)
cout << list1[i] << " ";
cout << endl;
// Transfer five elements from list1 to list2, Print list2
list2 = list1;
cout << "list2 : ";
for (i = 0; i < 5; i++)
cout << list2[i] << " ";
cout << endl;
// Write three elements replacing first 3 of list1
cout << "Enter 3 elements: ";
for (i = 0; i < 3; i++)
cin >> list1[i];
cout << endl;
// Prints three elements of list1 just entered
cout << "First three elements of list1: " << endl;
for (i = 0; i < 3; i++)
cout << list1[i] << " ";
cout << endl;
// Create list3 for first time
// Induced Chaos by
// cramming -2 to 6 into array list3
myArray list3(-2, 6);
// Print 8 array location numbers from -2 through 5
// Should print zero eight times if memory is initialized
cout << "list3: ";
for (i = -2; i < 6; i++) cout << list3[i] << " ";
cout << endl;
list3[-2] = 7;
list3[4] = 8;
list3[0] = 54;
list3[2] = list3[4] + list3[-2];
// Print results to screen
cout << "list3: ";
for (i = -2; i < 6; i++)
cout << list3[i] << " ";
cout << endl;
system("pause");
return 0;
}
.** Do not change anything in the supplied code which is the Ch13_Ex11_MainProgram.cpp (except to add documentation and your name). Please use the file names listed below since your file will have the same components in the supplied code.
2. **Recall that in C++, there is no check on an array index out of bounds.** However, during program execution, an array index out of bounds can cause serious problems. Also, in C++, the array index starts at 0.
3. **Design and implement the class `myArray`** that solves the array index out of bounds problem and also allows the user to begin the array index starting at any integer, positive or negative.
- Every object of type `myArray` is an array of type `int`. During execution, when accessing an array component, if the index is out of bounds, the program must terminate with an appropriate error message. Consider the following statements:
```cpp
myArray list(5);
//Line 1 myArray
myList(2, 13);
//Line 2
myArray yourList(-5, 9);
//Line 3
```
The statement in Line 1 declares list to be an array of 5 components, the component type is `int`, and the components are: `list[0]`, `list[1]`, ..., `list[4]`. The statement in Line 2 declares `myList` to be an array](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F69463cef-e943-4ccb-87fa-6ffaeb77fa1b%2Fa70ab880-8af1-452b-8709-1f67dae00897%2Fm11ly0t_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Could you please look at step 3, for some reasons I am getting errors?
![myArray.cpp
++Array Out_of_Bounds Instructions
#pragma once
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
myArray.h* X Ch13_Ex11_MainProgram.cpp
class myArray
{
public:
myArray (int indexs, int indexE);
myArray (int arrayLength);
int& operator[] (const int index);
private:
8:
int* list;
int length;
int indexStart;
int indexEnd;
};
Bint& myArray::operator[](int index) //overloading array index
{
if (index >= indexStart && index <= indexEnd) //check to see if its within bounds
{
}
else
{
}
int& indexLoc = *(list + index); //set memeory location to store actual value of the list index.
return indexLoc;
cout << "Index is out of range.\n";
exit(0);
→↓↓ myArray
}
✓ No issues found
100%
Error List
Entire Solution
Description
Code
XC2065
X C2065
'cout': undeclared identifier
'cout': undeclared identifier
X C3861 'exit': identifier not found
9
X 3 Errors A 0 Warnings ✪ 0 Messages Build+ IntelliSense
operator[](int index)
Project
Array Out_of_Bounds Instructions
Array Out of Bounds Instructions
Array Out_of_Bounds Instructions
File
myArray.h
myArray.h
myArray.h
Ln: 27
Ch: 36
Col: 42
Search Error List
Line
27
27
28](https://content.bartleby.com/qna-images/question/69463cef-e943-4ccb-87fa-6ffaeb77fa1b/8867ed70-23e2-4f57-80ae-ce5e45a38f48/m4k5qfs_thumbnail.png)
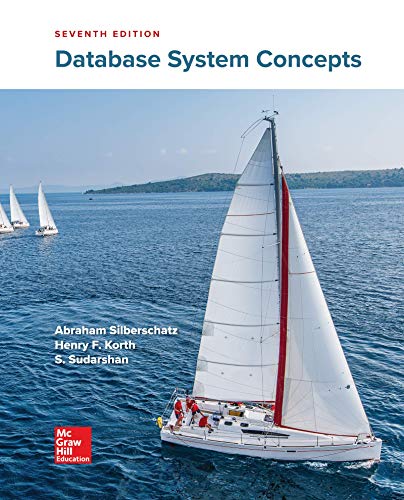
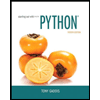
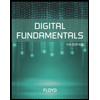
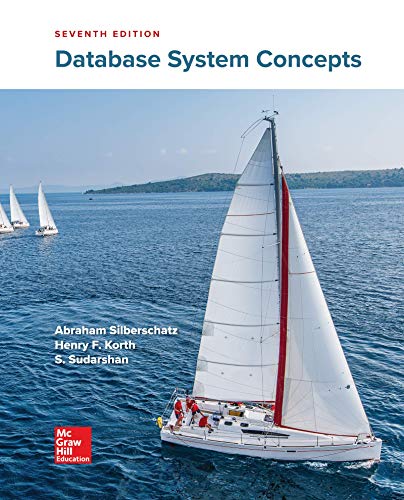
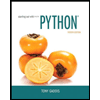
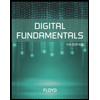
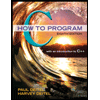
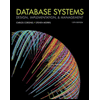
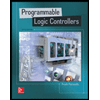