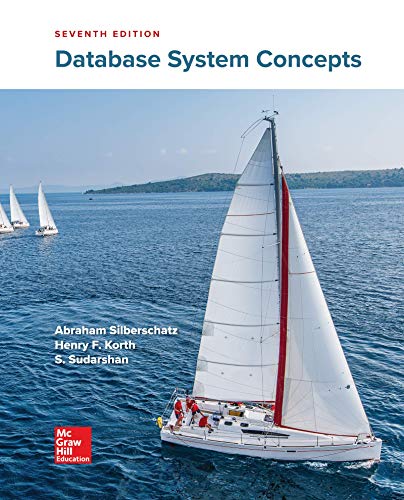
Please provide proper comments.
Define the following code.
#include <stdio.h>
2 #include <string.h>
3
4 #define STRING_LEN 20
5 #define SONG_BANK 5
6 #define SONGS 3
7
8 struct Song
9 {
10 char title[STRING_LEN + 1];
11 int seconds;
12 };
13
14 void calculateTimeFormat(int tSeconds, int* minutes, int* seconds);
15 void createPlaylist(struct Song playlist[], const struct Song bank[], int offset, int seed, int order[]);
16 void playSongs(const struct Song songs[], const int order[], int crossFade);
17
18 int main(void)
19 {
20 //
21 struct Song songBank[SONG_BANK] = { {"Song1", 546},
22 {"Song2", 295},
23 {"Song3", 399},
24 {"Song4", 234},
25 {"Song5", 111} },
26 playlist[SONGS] = { {0} };
27
28 //
29 //
30 //
31 int shuffled[SONGS] = { 0 }, config1 = 1, config2 = 26, config3 = 9;
32
33 createPlaylist(playlist, songBank, config1, config2, shuffled);
34 playSongs(playlist, shuffled, config3);
35
36 return 0;
37 }
38
39 void calculateTimeFormat(const int tSeconds, int* minutes, int* seconds)
40 {
41 *minutes = tSeconds / 60;
42 *seconds = tSeconds % 60;
43 }
44
45 void createPlaylist(struct Song playlist[], const struct Song bank[], int offset, int seed, int order[])
46 {
47 int i = 0, idx = seed % SONGS;
48
49 do
50 {
51 order[i] = idx + 2 < SONGS ? idx + 2 : idx + 2 - SONGS;
52
53 if (offset < 1 || offset > SONG_BANK)
54 {
55 offset = SONG_BANK;
56 }
57
58 playlist[i++] = bank[--offset];
59 idx = idx + 1 < SONGS ? idx + 1 : idx + 1 - SONGS;
60
61 } while (i < SONGS);
62 }
63
64 void playSongs(const struct Song songs[], const int order[], int crossFade)
65 {
66 int i, min, sec, total = 0;
67
68 for (i = 0; i < SONGS; i++)
69 {
70 total += songs[order[i]].seconds;
71
72 if (crossFade && (i + 1 < SONGS))
73 {
74 total -= crossFade;
75 calculateTimeFormat(songs[order[i]].seconds - crossFade, &min, &sec);
76 }
77 else
78 {
79 calculateTimeFormat(songs[order[i]].seconds, &min, &sec);
80 }
81 printf("%-20s %2d:%02d\n", songs[order[i]].title, min, sec);
82 }
83
84 calculateTimeFormat(total, &min, &sec);
85 printf("Total playing time: %3d:%02d\n", min, sec);
86 }
87

Step by stepSolved in 2 steps with 3 images

- 4. An array named nums contains the following numbers: 5, 10, 15. It is declared as follows: box const nums = [5, 10, 15]; Write the necessary code to display each item in the array in an alert using a for loop?arrow_forwardfood_wastage_record.hpp class FoodWastageRecord {public:void SetDate(const std::string &date);void SetMeal(const std::string &meal);void SetFoodName(const std::string &food_name);void SetQuantityInOz(double qty_in_oz);void SetWastageReason(const std::string &wastage_reason);void SetDisposalMechanism(const std::string &disposal_mechanism);void SetCost(double cost); std::string Date() const;std::string Meal() const;std::string FoodName() const;double QuantityInOz() const;std::string WastageReason() const;std::string DisposalMechanism() const;double Cost() const; private:std::string date_;std::string meal_;std::string food_name_;double qty_in_oz_;std::string wastage_reason_;std::string disposal_mechanism_;double cost_;}; food_wastage_record.cpp #include "food_wastage_record.h" void FoodWastageRecord::SetDate(const std::string &date) { date_ = date; }void FoodWastageRecord::SetMeal(const std::string &meal) { meal_ = meal; }void…arrow_forwardWrite a function getNeighbors which will accept an integer array, size of the array and an index as parameters. This function will return a new array of size 2 which stores the neighbors of the value at index in the original array. If this function would result in returning garbage values the new array should be set to values {0,0} instead of values from the array.arrow_forward
- please go in to the detail and explain the purpose and function to each line & function of code listed below. Dsecribe each line. #include <stdio.h>//including headers#include <string.h>#include <stdlib.h> struct node{//structure intialization int data; struct node *next; }; #include <assert.h> typedef struct Info_ { char name[100]; } Info; typedef struct Compar_ { Info info; struct Compar_* next; } Compar; void print_comparisons(Compar* CP)//comparison method { assert(CP); Compar* cur = CP; Compar* next = cur->next; for (; next; cur = next, next = next->next) { if (strcmp(cur->info.name, next->info.name) == 0) printf("Same name\n"); else printf("Diff name\n"); } } struct node *head, *tail = NULL; void addNode(int data) {//adding node method struct node *newNode = (struct node*)malloc(sizeof(struct node)); newNode->data = data; newNode->next = NULL; if(head == NULL) { head = newNode; tail = newNode; } else { tail->next =…arrow_forwardDice_Game.cpp #include <iostream>#include "Die.h" using namespace std; // a struct for game variablesstruct GameState { int turn = 1; int score = 0; int score_this_turn = 0; bool turn_over = false; bool game_over = false; Die die;}; // declare functionsvoid display_rules();void play_game(GameState&);void take_turn(GameState&);void roll_die(GameState&);void hold_turn(GameState&); int main() { display_rules(); GameState game; play_game(game);} // define functionsvoid display_rules() { cout << "Dice Game Rules:\n" << "\n" << "* See how many turns it takes you to get to 20.\n" << "* Turn ends when you hold or roll a 1.\n" << "* If you roll a 1, you lose all points for the turn.\n" << "* If you hold, you save all points for the turn.\n\n";} void play_game(GameState& game) { while (!game.game_over) { take_turn(game); } cout << "Game…arrow_forward6. sum_highest_five This function takes a list of numbers, finds the five largest numbers in the list, and returns their sum. If the list of numbers contains fewer than five elements, raise a ValueError with whatever error message you like. Sample calls should look like: >>> sum_highest_five([10, 10, 10, 10, 10, 5, -17, 2, 3.1])50>>> sum_highest_five([5])Traceback (most recent call last): File "/usr/lib/python3.8/idlelib/run.py", line 559, in runcode exec(code, self.locals) File "<pyshell#44>", line 1, in <module> File "/homework/final.py", line 102, in sum_highest_five raise ValueError("need at least 5 numbers")ValueError: need at least 5 numbersarrow_forward
- main.cc file #include <iostream>#include <memory> #include "train.h" int main() { // Creates a train, with the locomotive at the front of the train. // LinkedList diagram: // Locomotive -> First Class -> Business Class -> Cafe Car -> Carriage 1 -> // Carriage 2 std::shared_ptr<Train> carriage2 = std::make_shared<Train>(100, 100, nullptr); std::shared_ptr<Train> carriage1 = std::make_shared<Train>(220, 220, carriage2); std::shared_ptr<Train> cafe_car = std::make_shared<Train>(250, 250, carriage1); std::shared_ptr<Train> business_class = std::make_shared<Train>(50, 50, cafe_car); std::shared_ptr<Train> first_class = std::make_shared<Train>(20, 20, business_class); std::shared_ptr<Train> locomotive = std::make_shared<Train>(1, 1, first_class); std::cout << "Total passengers in the train: "; // =================== YOUR CODE HERE…arrow_forwardCreate pseudocode for the following #include <iostream> #include <string> #include <cstdlib> #include <ctime> using namespace std; char water[10][10] = {{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'}}; void createBoard(int &numShip); void promptCoords(int& userX, int &userY); void shipGen(int shipX[] ,int shipY[], int &numShip); void testCoords(int &userX, int &userY, int shipX[], int shipY[], int &numShip, int& victory); void updateBoard();arrow_forwardvrite a each eler the original assigns the element with 0. Ex: lowerScores = {5, 0, 2, -3} becomes {4, 0, 1, 0}. 406554.2871636.qx3zqy7 1 #include 2 #include 3 using namespace std; 4 5 int main() { 6 7 9 10 11 12 13 14 15 16 17 Run const int SCORES_SIZE = 4; vector lowerScores (SCORES_SIZE); unsigned int i; for (i 0; i > lowerScores.at(i); } /* Your solution goes here */ for (i = 0; i < lowerScores.size(); ++i) { cout << lowerScores.at (i) << " "; nent was greater than 0, and oth wise.arrow_forward
- please use DEQUE #include <iostream>#include <string>#include <deque> using namespace std; const int AIRPORT_COUNT = 12;string airports[AIRPORT_COUNT] = {"DAL","ABQ","DEN","MSY","HOU","SAT","CRP","MID","OKC","OMA","MDW","TUL"}; int main(){// define stack (or queue ) herestring origin;string dest;string citypair;cout << "Loading the CONTAINER ..." << endl;// LOAD THE STACK ( or queue) HERE// Create all the possible Airport combinations that could exist from the list provided.// i.e DALABQ, DALDEN, ...., ABQDAL, ABQDEN ...// DO NOT Load SameSame - DALDAL, ABQABQ, etc .. cout << "Getting data from the CONTAINER ..." << endl;// Retrieve data from the STACK/QUEUE here } Using the attached shell program (AirportCombos.cpp), create a list of strings to process and place on a STL DEQUE container. Using the provided 3 char airport codes, create a 6 character string that is the origin & destination city pair. Create all the possible…arrow_forwardC++arrow_forward#include <iostream>#include <iomanip>#include <string>using namespace std;struct menuItemType{string menuItem;double menuPrice;};void getData(menuItemType menuList[]);void showMenu(menuItemType menuList[] , int n);void printCheck(menuItemType menuList[], int menu_order[], int n);int main(){const int item = 8;menuItemType menuList[item];int menu_order[item] ={0};int choice =0;int count =0;bool order = true;getData(menuList);showMenu(menuList,item);while(order){cout<<"Enter the choice for the order or press 0 to exit"<<endl;cin>>choice;if(choice>0 && choice<=item){menu_order[choice-1]+=1;}else{order=false;}}printCheck(menuList,menu_order,item);return 0;}void getData(menuItemType menuList[]){menuItemType plainEgg;menuItemType baconEgg;menuItemType muffin;menuItemType frenchToast;menuItemType fruitBasket;menuItemType cereal;menuItemType coffee;menuItemType tea;plainEgg.menuItem = "Plain Egg";plainEgg.menuPrice = 1.45;baconEgg.menuItem =…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
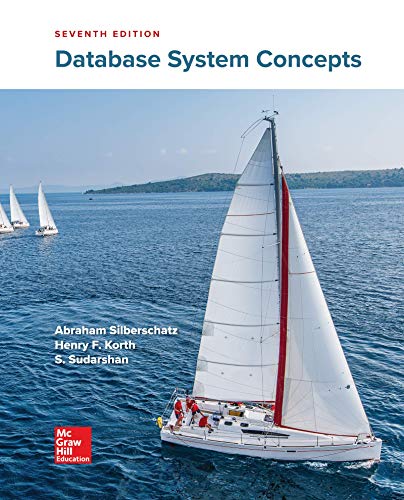
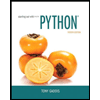
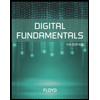
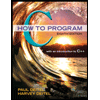
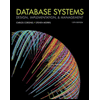
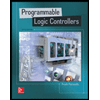