"The variable userNumber should be used for storing the input the user gives you. The variable maxVal keeps track of the largest integer found so far. In the loop, prompt and store the user's number. Then check, if userNumber is positive and userNumber is greater than maxVal. If it is, then update maxVal to equal userNumber. Repeat this process until userNumber is equal to the SENTINEL variable."
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
I am not sure entirely How to store max value. These are my instructions:
"The variable userNumber should be used for storing the input the user gives you. The variable maxVal keeps track of the largest integer found so far.
In the loop, prompt and store the user's number. Then check, if userNumber is positive and userNumber is greater than maxVal. If it is, then update maxVal to equal userNumber. Repeat this process until userNumber is equal to the SENTINEL variable."
This is what I have thus far:
#include <iostream>
using namespace std;
int main()
{
// variables
int maxVal = 0, userNumber = 0;
const int SENTINEL = -1;
// TODO: create a `while` loop version of finding the maximum number
cout << "Enter a number: ";
cin >> userNumber;
while (userNumber != SENTINEL)
{
userNumber = ++maxVal;
cout << userNumber << endl;
}
// display results
cout << "\nMaximum number entered: " << maxVal << endl;
// terminate
return 0;
}
What am I missing?

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

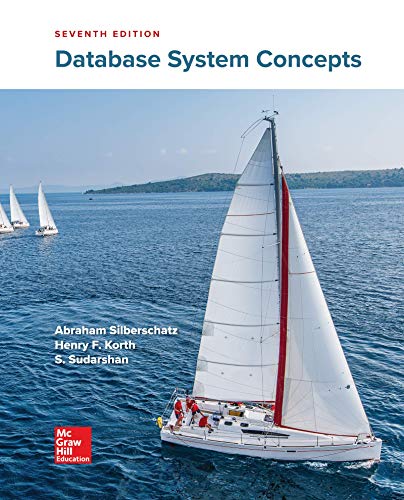
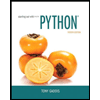
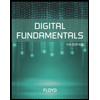
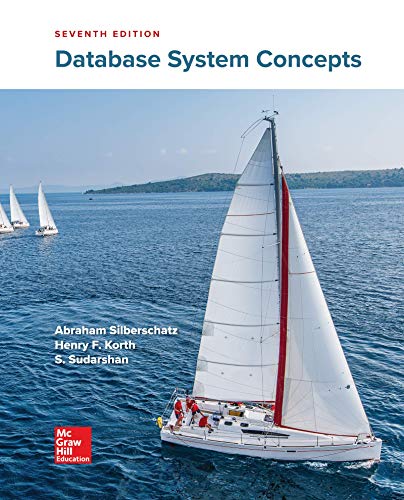
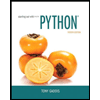
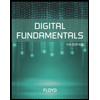
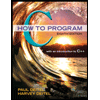
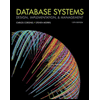
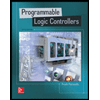