The three java files (source code) pasted below associated with this question. Any help will be greatly appreciated!! QUESTION (using Eclipse and default Junit package that came with it): Create a Junit 4 test case for the Hourly class. Your test should use these methods from class org.junit.Assert: assertEquals to test the getJob() method assertEquals to test the getPayRate() method assertTrue to test the hours attribute Admin.java package ch44extra; import java.time.LocalDate; public class Admin extends Person { private String dept; private String office; private double salary; //private double budget;removed to simplify class public Admin(String name, LocalDate date, int id, String dept, String office, double salary) { super(name, date, id); this.dept = dept; this.office = office; this.salary = salary; } public Admin() { super(); // TODO Auto-generated constructor stub } public String getDept() { return dept; } public void setDept(String dept) { this.dept = dept; } public String getOffice() { return office; } public void setOffice(String office) { this.office = office; } public double getSalary() { return salary; } public void setSalary(double salary) { this.salary = salary; } @Override public void pay() { double pay = salary / 12; System.out.printf("Annual Salary pays $%,.2f monthly\n",pay); } @Override public String toString() { Strings = super.toString(); s += "\nAdmin dept=" + dept + ", office=" + office + ", salary=" + String.format("$%,.0f",salary); returns; } } Hourly.java package ch44extra; import java.time.LocalDate; public class Hourly extends Person { private double payRate; private double hours; private String job; public Hourly(String name, LocalDate date, int id, double payRate, double hours, String job) { super(name, date, id); this.payRate = payRate; this.hours = hours; this.job = job; } public double getPayRate() { return payRate; } public void setPayRate(double payRate) { this.payRate = payRate; } public double getHours() { return hours; } public void setHours(double hours) { this.hours = hours; } public String getJob() { return job; } public void setJob(String job) { this.job = job; } @Override// implements abstract method pay inherited from Person public void pay() { double pay = payRate * hours; System.out.printf("%.2f hours @ $%.2f = $%.2f weekly\n",hours,payRate,pay); } @Override public String toString() { return super.toString() + "\n" + "payRate=" + String.format("$%.2f",payRate) + ", hours=" + hours + ", job=" + job; } } Person.java package ch44extra; import java.time.LocalDate; // abstract because it contains the abstract method pay public abstract class Person implements Comparable { protected String name; protected LocalDate date; protected int id; // deleted salary field, added id public Person(String name, LocalDate date, int id) { super(); this.name = name; this.date = date; this.id = id; } public Person() { } // getters not needed since attributes are now protected // abstract method, so Person class must be abstract public abstract void pay(); // no braces, no body @Override public int compareTo(Person arg0) { return date.compareTo(arg0.date); } @Override public String toString() { return "Name=" + name + ", hired=" + date + ", id number=" + id ; } }
The three java files (source code) pasted below associated with this question.
Any help will be greatly appreciated!!
QUESTION (using Eclipse and default Junit package that came with it):
Create a Junit 4 test case for the Hourly class. Your test should use these methods from class org.junit.Assert:
- assertEquals to test the getJob() method
- assertEquals to test the getPayRate() method
- assertTrue to test the hours attribute
Admin.java
package ch44extra;
import java.time.LocalDate;
public class Admin extends Person {
private String dept;
private String office;
private double salary;
//private double budget;removed to simplify class
public Admin(String name, LocalDate date, int id, String dept, String office, double salary) {
super(name, date, id);
this.dept = dept;
this.office = office;
this.salary = salary;
}
public Admin() {
super();
// TODO Auto-generated constructor stub
}
public String getDept() {
return dept;
}
public void setDept(String dept) {
this.dept = dept;
}
public String getOffice() {
return office;
}
public void setOffice(String office) {
this.office = office;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
@Override
public void pay() {
double pay = salary / 12;
System.out.printf("Annual Salary pays $%,.2f monthly\n",pay);
}
@Override
public String toString() {
Strings = super.toString();
s += "\nAdmin dept=" + dept + ", office=" + office + ", salary=" + String.format("$%,.0f",salary);
returns;
}
}
Hourly.java
package ch44extra;
import java.time.LocalDate;
public class Hourly extends Person {
private double payRate;
private double hours;
private String job;
public Hourly(String name, LocalDate date, int id, double payRate, double hours, String job) {
super(name, date, id);
this.payRate = payRate;
this.hours = hours;
this.job = job;
}
public double getPayRate() {
return payRate;
}
public void setPayRate(double payRate) {
this.payRate = payRate;
}
public double getHours() {
return hours;
}
public void setHours(double hours) {
this.hours = hours;
}
public String getJob() {
return job;
}
public void setJob(String job) {
this.job = job;
}
@Override// implements abstract method pay inherited from Person
public void pay() {
double pay = payRate * hours;
System.out.printf("%.2f hours @ $%.2f = $%.2f weekly\n",hours,payRate,pay);
}
@Override
public String toString() {
return super.toString() + "\n" + "payRate=" + String.format("$%.2f",payRate) + ", hours=" + hours + ", job=" + job;
}
}
Person.java
package ch44extra;
import java.time.LocalDate;
// abstract because it contains the abstract method pay
public abstract class Person implements Comparable<Person> {
protected String name;
protected LocalDate date;
protected int id; // deleted salary field, added id
public Person(String name, LocalDate date, int id) {
super();
this.name = name;
this.date = date;
this.id = id;
}
public Person() {
}
// getters not needed since attributes are now protected
// abstract method, so Person class must be abstract
public abstract void pay(); // no braces, no body
@Override
public int compareTo(Person arg0) {
return date.compareTo(arg0.date);
}
@Override
public String toString() {
return "Name=" + name + ", hired=" + date + ", id number=" + id ;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

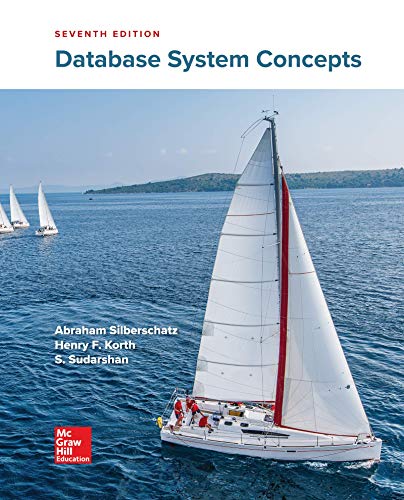
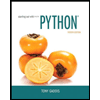
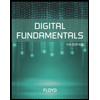
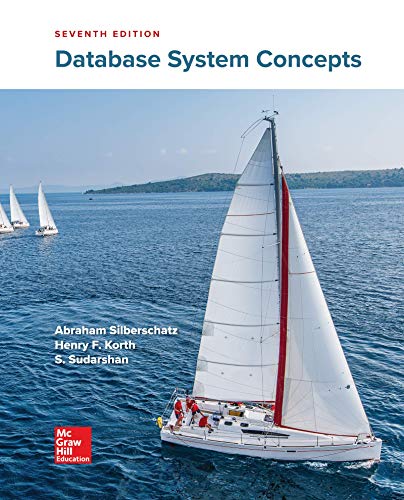
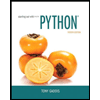
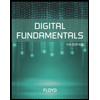
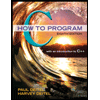
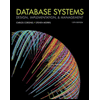
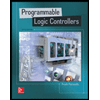