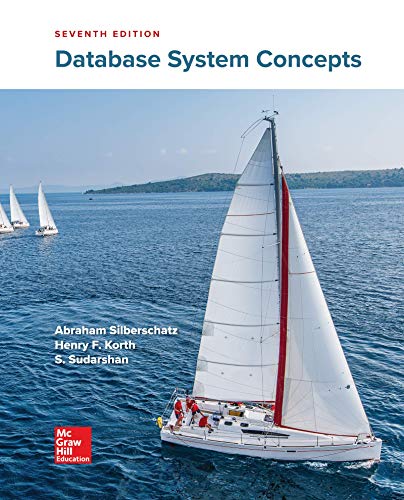
Concept explainers
The program below simulates tossing a fair coin N times. The program computes the longest sequence of consecutive heads. Or it should compute the longest sequence of consecutive heads. It does not compute the longest sequence of consecutive because one statement is missing and one statement is in the wrong place in the program. Supply the missing statement and move the out of place statement to the right position in the program.
If you toss the coin a hundred times the longest sequence of consecutive heads is usually 5 or 6.
#include <ctime>
#include <iostream>
#include <random>
#include <string>
using namespace std;
int main()
{
const int HEADS = 0;
const int TAILS = 1;
int seed = (int)time(nullptr);
default_random_engine e(seed);
uniform_int_distribution<int> u(HEADS, TAILS);
int prevCoin = -1;
int N = 100;
int maxlength = 0;
int length = 0;
for (int n = 1; n <= N; ++n)
{
if (coin == HEADS)
{
if (prevCoin == coin)
length++;
else
length = 1;
}
else
{
if (length > maxlength)
maxlength = length;
prevCoin = coin;
}
}
if (length > maxlength)
maxlength = length;
cout << "Maxlength = " << maxlength << endl;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

- Create a program that allows the user to enter a person's age (in years) and current salary. Both Next, you plan the algorithm and then desk-check it. Figure 8-15 shows the completed IPO algorithm contains two loops. The outer loop keeps track of the three annual raise rates (3%, 4%, chart and desk-check table, which (for simplicity) uses an age of 62 and a salary of $25000. The before retirement at age 65, using annual raise rates of 3%, 4%, and 5%. To calculate then for the input. In this case, the program should display a person's total earnings in Figure 8-15. You begin by analyzing the problem, looking first for the output and In this lab, you will plan and create an algorithm for the problem specification shown Frepure 8-15 Problem specification, IPO chart, and desk-check table for the retirement algorithm (continues) and 5%). The nested loop keeps track of the number of years until retirement. the amounts, the program will need to know the person's age and current salary. The…arrow_forwardTransient PopulationPopulations are affected by the birth and death rate, as well as the number of people who move in and out each year. The birth rate is the percentage increase of the population due to births and the death rate is the percentage decrease of the population due to deaths. Write a program that displays the size of a population for any number of years. The program should ask for the following data: The starting size of a population P The annual birth rate (as a percentage of the population expressed as a fraction in decimal form)B The annual death rate (as a percentage of the population expressed as a fraction in decimal form)D The average annual number of people who have arrived A The average annual number of people who have moved away M The number of years to display nYears Write a function that calculates the size of the population after a year. To calculate the new population after one year, this function should use the formulaN = P + BP - DP + A - Mwhere N is the…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
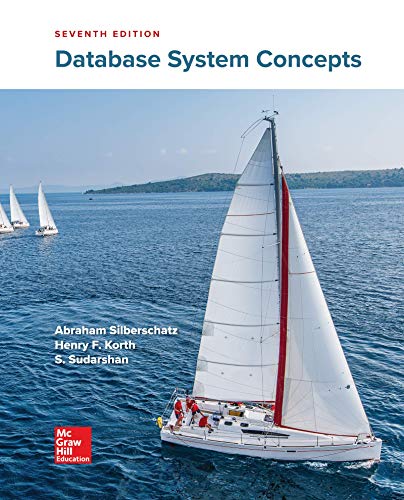
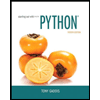
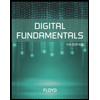
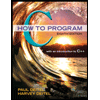
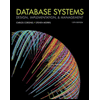
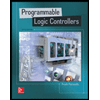