The problem I have is, say I am storing the phone number and don't want their phone number to contain any characters, have a length of 10 numbers and start with 1 and return "Not Valid" if one of these occur. I have tried to create if statements in the setter but it is not returning the "Not Valid". This is what I have so far import java.util.Scanner; class Clint { private String id; private String name; private long prson; private Address home; public Clint() { prson = 0; } public Clint(String acc, String nameGiven, long bal, Address residence) { id = acc; name = nameGiven; prson = bal; home = residence; } Scanner sc = new Scanner(System.in); // deposit money into the bank account by given amount amt public void deposit(long amt) { prson += amt; } // if amount is not given ask the user for the amount public void deposit() { System.out.print("Enter Amount(to deposit): $"); long amt = sc.nextLong(); prson += amt; } // withdraw money from the bank account public void withdraw(long amt) { if (prson >= amt) { prson -= amt; } else { System.out.println("Insufficient funds!"); } } // if amt is not given ask the user for the amount public void withdraw() { System.out.print("Enter Amount(to withdraw): $"); long amt = sc.nextLong(); if (prson >= amt) { prson -= amt; } else { System.out.println("Insufficient funds!"); } } // getters and setters for all the private members public String getAccNumber() { return id; } public String getHolderName() { return name; } public long getBalance() { return prson; } public void setBalance(long amt) { prson = amt; } public void setHolderName(String n) { name = n; } public void setAccNumber(String acc) { id = acc; } public void setPhoneNumber(String phoneNumber) { if (phoneNumber.matches("[a-zA-z]+)")) { phoneNumber = "Not Valid"; } else if (phoneNumber.length() > 10) { phoneNumber = "Not Valid"; } else if (phoneNumber.startsWith("1")){ phoneNumber = "Not Valid"; } } // method to display account details public void printDetails() { System.out.println("[" + id + "-" + name + ": $" + prson +home.toString()+ "]"); } } public class Address { private String phoneNumber; // The city in which the address is located private String city; // The state in which the address is located private String street; public Address(String number,String road, String town ) { phoneNumber= number; city = town; street = road; } public String getPhoneNumber() { return phoneNumber; } // public void setPhoneNumber(String phoneNumber) { // if (phoneNumber.matches("[a-zA-z]+)")) { // phoneNumber = "Not Valid"; // } // else if (phoneNumber.length() > 10) { // phoneNumber = "Not Valid"; // } // // else if (phoneNumber.startsWith("1")){ // phoneNumber = "Not Valid"; // } // else { // this.phoneNumber = phoneNumber; // } // } public String getCity() { return city; } public void setCity(String name) { this.city = city; } public String getstreet() { return street; } public void setStreet(String Street) { this.street = Street; } /** The toString method @return Information about the address. */ public String toString() { return " Name: " + phoneNumber + city + "\n treet " + phoneNumber; } }
The problem I have is, say I am storing the phone number and don't want their phone number to contain any characters, have a length of 10 numbers and start with 1 and return "Not Valid" if one of these occur.
I have tried to create if statements in the setter but it is not returning the "Not Valid". This is what I have so far
import java.util.Scanner;
class Clint {
private String id;
private String name;
private long prson;
private Address home;
public Clint() {
prson = 0;
}
public Clint(String acc, String nameGiven, long bal, Address residence) {
id = acc;
name = nameGiven;
prson = bal;
home = residence;
}
Scanner sc = new Scanner(System.in);
// deposit money into the bank account by given amount amt
public void deposit(long amt) {
prson += amt;
}
// if amount is not given ask the user for the amount
public void deposit() {
System.out.print("Enter Amount(to deposit): $");
long amt = sc.nextLong();
prson += amt;
}
// withdraw money from the bank account
public void withdraw(long amt) {
if (prson >= amt) {
prson -= amt;
} else {
System.out.println("Insufficient funds!");
}
}
// if amt is not given ask the user for the amount
public void withdraw() {
System.out.print("Enter Amount(to withdraw): $");
long amt = sc.nextLong();
if (prson >= amt) {
prson -= amt;
} else {
System.out.println("Insufficient funds!");
}
}
// getters and setters for all the private members
public String getAccNumber() {
return id;
}
public String getHolderName() {
return name;
}
public long getBalance() {
return prson;
}
public void setBalance(long amt) {
prson = amt;
}
public void setHolderName(String n) {
name = n;
}
public void setAccNumber(String acc) {
id = acc;
}
public void setPhoneNumber(String phoneNumber) {
if (phoneNumber.matches("[a-zA-z]+)")) {
phoneNumber = "Not Valid";
}
else if (phoneNumber.length() > 10) {
phoneNumber = "Not Valid";
}
else if (phoneNumber.startsWith("1")){
phoneNumber = "Not Valid";
}
}
// method to display account details
public void printDetails() {
System.out.println("[" + id + "-" + name + ": $" + prson +home.toString()+ "]");
}
}
public class Address {
private String phoneNumber;
// The city in which the address is located
private String city;
// The state in which the address is located
private String street;
public Address(String number,String road, String town )
{
phoneNumber= number;
city = town;
street = road;
}
public String getPhoneNumber() {
return phoneNumber;
}
// public void setPhoneNumber(String phoneNumber) {
// if (phoneNumber.matches("[a-zA-z]+)")) {
// phoneNumber = "Not Valid";
// }
// else if (phoneNumber.length() > 10) {
// phoneNumber = "Not Valid";
// }
//
// else if (phoneNumber.startsWith("1")){
// phoneNumber = "Not Valid";
// }
// else {
// this.phoneNumber = phoneNumber;
// }
// }
public String getCity() {
return city;
}
public void setCity(String name) {
this.city = city;
}
public String getstreet() {
return street;
}
public void setStreet(String Street) {
this.street = Street;
}
/**
The toString method
@return Information about the address.
*/
public String toString() {
return " Name: " + phoneNumber + city + "\n treet " + phoneNumber;
}
}

Step by step
Solved in 3 steps with 4 images

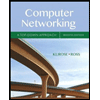
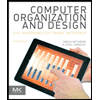
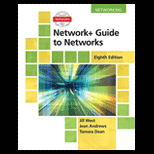
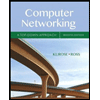
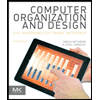
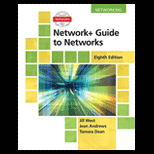
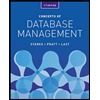
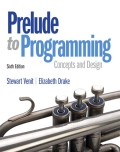
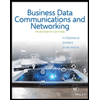