The owners of the Annan Supermarket would like to have a program that computes the weekly gross pay of their employees. The user will enter an employee’s first name, last name, the hourly rate of pay, and the number of hours worked for the week. In addition, Annan Supermarkets would like the program to compute the employee’s net pay and overtime pay. Overtime hours, any hours over 40, are paid at 1.5 the regular hourly rate. Net pay is gross pay minus taxes (Refer to the tax table on the second page). Define a class called Employee. The class must have private attributes to store the employee's name, hourly rate, and regular (≤ 40) and overtime hours worked. The class must also have methods to perform the following tasks: • A constructor to initialize the hourly rate to the minimum wage of $7.25 per hour and the hours worked (regular and overtime) to 0.0. • A method to get (a setter/mutator method) ⬧ the employee's name ⬧ the hourly rate ⬧ the hours work for the month (by the week – assume 4 weeks in a month). Do not write separate setters for regular and overtime hours. • A method to return (a getter/accessor method) ⬧ the employee's name ⬧ the hourly rate ⬧ the total regular hours work for the month ⬧ the total overtime hours for the month • A method to return (a getter/accessor method) ⬧ the monthly regular pay • A method to return (a getter/accessor method) ⬧ the monthly overtime pay • A __str__ method to display the output which must include the following information: ⬧ Employee's name ⬧ Total regular hours worked ⬧ Total overtime hours worked ⬧ Total hours worked ⬧ Pay rate ⬧ Monthly Regular Pay ⬧ Monthly overtime pay ⬧ Monthly gross pay ⬧ Monthly taxes ⬧ Monthly net pay Write a main function that declares an object for the class defined and test the functionsand methods written for the class. Allow the user to run the program as many times as possible. No input, processing, or output should happen in the main function. All work should be delegated to other functions. Include the recommended minimum documentation for each function.
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
The owners of the Annan Supermarket would like to have a program that computes the weekly gross pay of their employees. The user will enter an employee’s first name, last name, the hourly rate of pay, and the number of hours worked for the week. In addition, Annan Supermarkets would like the program to compute the employee’s net pay and overtime pay. Overtime hours, any hours over 40, are paid at 1.5 the regular hourly
rate. Net pay is gross pay minus taxes (Refer to the tax table on the second page).
Define a class called Employee. The class must have private attributes to store the employee's name, hourly rate, and regular (≤ 40) and overtime hours worked. The class must also have methods to perform the following tasks:
• A constructor to initialize the hourly rate to the minimum wage of $7.25 per hour
and the hours worked (regular and overtime) to 0.0.
• A method to get (a setter/mutator method)
⬧ the employee's name
⬧ the hourly rate
⬧ the hours work for the month (by the week – assume 4 weeks in a month).
Do not write separate setters for regular and overtime hours.
• A method to return (a getter/accessor method)
⬧ the employee's name
⬧ the hourly rate
⬧ the total regular hours work for the month
⬧ the total overtime hours for the month
• A method to return (a getter/accessor method)
⬧ the monthly regular pay
• A method to return (a getter/accessor method)
⬧ the monthly overtime pay
• A __str__ method to display the output which must include the following
information:
⬧ Employee's name
⬧ Total regular hours worked
⬧ Total overtime hours worked
⬧ Total hours worked
⬧ Pay rate
⬧ Monthly Regular Pay
⬧ Monthly overtime pay
⬧ Monthly gross pay
⬧ Monthly taxes
⬧ Monthly net pay
Write a main function that declares an object for the class defined and test the functionsand methods written for the class. Allow the user to run the program as many times as possible. No input, processing, or output should happen in the main function. All work should be delegated to other functions. Include the recommended minimum documentation for each function.
This is the question and the remaining part is in the picture that i have attached . Use python to solve this program. Thank you.


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

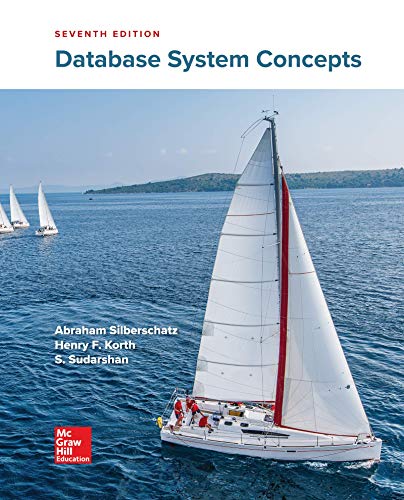
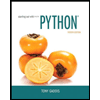
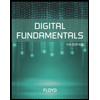
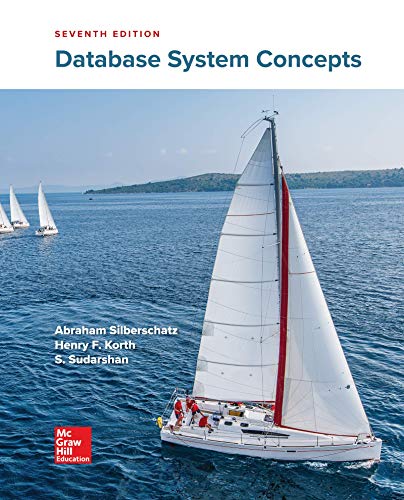
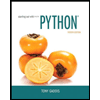
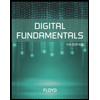
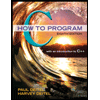
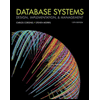
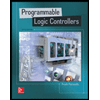