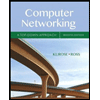
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Can I please get help with this
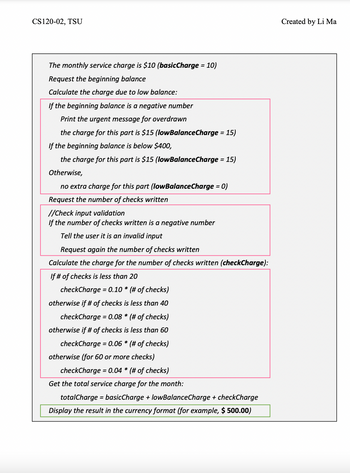
Transcribed Image Text:**Overview of Bank Service Charge Calculation**
This guide aims to assist users in understanding how to calculate monthly service charges for a bank account based on the beginning balance and the number of checks written.
1. **Basic Monthly Service Charge:**
- The monthly service charge is set at $10. This is referred to as `basicCharge`.
2. **Beginning Balance Inquiry:**
- Users are prompted to enter their beginning balance.
3. **Low Balance Charge Calculation:**
- If the beginning balance is a negative number:
- Print an urgent message indicating the account is overdrawn.
- A charge of $15 (denoted as `lowBalanceCharge`) applies.
- If the beginning balance is below $400:
- A charge of $15 applies.
- Otherwise:
- No extra charge is applied for this section.
4. **Number of Checks Written:**
- Users are requested to input the number of checks they have written.
5. **Input Validation for Checks:**
- If the number of checks is negative:
- Inform the user that the input is invalid.
- Prompt the user to enter the number again.
6. **Check Charge Calculation:**
- Based on the number of checks written, calculate `checkCharge` as follows:
- If less than 20 checks: `checkCharge = 0.10 * (number of checks)`
- If less than 40 checks: `checkCharge = 0.08 * (number of checks)`
- If less than 60 checks: `checkCharge = 0.06 * (number of checks)`
- If 60 or more checks: `checkCharge = 0.04 * (number of checks)`
7. **Total Monthly Service Charge Calculation:**
- Compute the total service charge using the formula:
`totalCharge = basicCharge + lowBalanceCharge + checkCharge`
8. **Display of Result:**
- Present the total service charge to the user in a currency format (e.g., $500.00).
This flowchart provides a structured methodology for users to accurately determine the bank service charges applicable for a given month based on specific criteria.
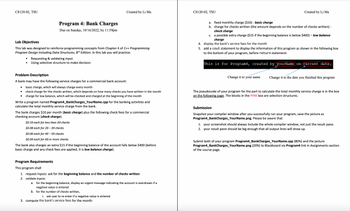
Transcribed Image Text:# Program 4: Bank Charges
**Due on Sunday, 10/16/2022, by 11:59pm**
## Lab Objectives
This lab aims to reinforce programming concepts from Chapter 4 of *C++ Programming: Program Design Including Data Structures, 8th Edition*. In this lab you will practice:
- Requesting & validating input
- Using selective structure to make decisions
## Problem Description
A bank may have the following service charges for a commercial bank account:
- Basic charge, which will always charge every month
- Check charge for the checks written, which depends on the number of checks you have written in the month
- Charge for low balance, which will be checked and charged at the beginning of the month
Write a program named `Program4_BankCharges_YourName.cpp` for the banking activities and calculate the total monthly service charge from the bank.
The bank charges $10 per month (*basic charge*) plus the following check fees for a commercial checking account (*check charge*):
- $0.10 each for less than 20 checks
- $0.08 each for 20 – 39 checks
- $0.06 each for 40 – 59 checks
- $0.04 each for 60 or more checks
The bank also charges an extra $15 if the beginning balance of the account falls below $400 (before basic charge and any check fees are applied, it is *low balance charge*).
## Program Requirements
This program shall:
1. Request inputs: ask for the **beginning balance** and the **number of checks written**
2. Validate inputs:
- For the beginning balance, display an urgent message indicating the account is overdrawn if a negative value is entered.
- For the number of checks written, ask the user to re-enter if a negative value is entered.
3. Compute the bank’s service fees for the month:
- Fixed monthly charge ($10) - *basic charge*
- Charge for checks written (the amount depends on the number of checks written) - *check charge*
- A possible extra charge ($15 if the beginning balance is below $400) - *low balance charge*
4. Display the bank’s service fees for the month.
5. Add a `cout` statement to display the information of this program as shown in the following box at the bottom of your program, before `return` statement:
```
Expert Solution

arrow_forward
Step 1
Answer:
We have done in C++ programming language and also attached the code and code screenshot and output
Algorithms:
Step1: we have asked the user input for the beginning balance
Step2: we check beginning balance is negative then message urgent message for withdrawn
Step3: then we have ask the user to give the input for check
Step4: if beginning balance is less than the 400$ then calculate the charge charge based on check and calculate the charge with the basic charge +low balance charge
Step5: if beginning balance is greater than the 400$ then calculate the charge charge based on check +basic charge
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Similar questions
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
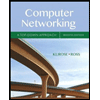
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
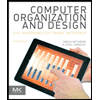
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
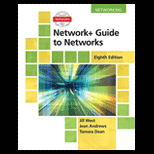
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
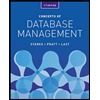
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
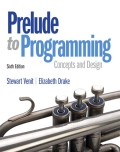
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
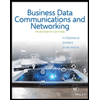
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY