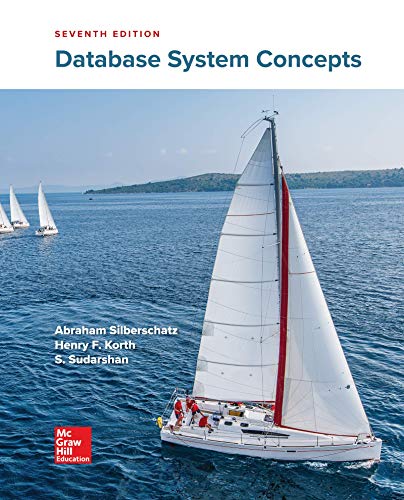
Concept explainers
The following recursive method is causing an exception because the base case is missing.
This method is suppose to display the content of an array backward. For example If the array num contains 1 2 3 4 5 6 then the call print(num, 5) should display: 6 5 4 3 2 1
public static void print(int[] a, int index)
{
System.out.println(a[index]);
print(a, index -1);
}
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index -1 out of bounds for length 5
fill in the blank so that the exception does not happen, in order of the given numbers
public static void print(int[] a, int index)
{
if( __1___ ___2__ ___3__) <----- base case
__4____
System.out.println(a[index]);
print(a, index -1); <---- recursive case
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- 1. Write a recursive method expFive(n) to compute y=5^n. For instance, if n is 0, y is 1. If n is 3, then y is 125. If n is 4, then y is 625. The recursive method cannot have loops. Then write a testing program to call the recursive method. If you run your program, the results should look like this: > run RecExpTest Enter a number: 3 125 >run RecExpTest Enter a number: 3125 2. For two integers m and n, their GCD(Greatest Common Divisor) can be computed by a recursive function. Write a recursive method gcd(m,n) to find their Greatest Common Divisor. Once m is 0, the function returns n. Once n is 0, the function returns m. If neither is 0, the function can recursively calculate the Greatest Common Divisor with two smaller parameters: One is n, the second one is m mod n. Although there are other approaches to calculate Greatest Common Divisor, please follow the instructions in this question, otherwise you will not get the credit. Meaning your code needs to follow the given algorithm. Then…arrow_forward1. The sorted values array contains 16 integers 5, 7, 10, 13, 13, 20, 21, 25, 30,32, 40, 45, 50, 52, 57, 60. Indicate the sequence of recursive calls that are made tobinaraySearch, given an initial invocation of binarySearch(32, 0, 15).show only the recursive calls. For example, initial invocation is binarySearch(45,0,15)where the target is 45, first is 0 and last is 15.arrow_forwardThe following recursive method get Number Equal searches the array x of 'n integers for occurrences of the integer val. It returns the number of integers in x that are equal to val. For example, if x contains the 9 integers 1, 2, 4, 4, 5, 6, 7, 8, and 9, then getNumberEqual(x, 9, 4) returns the value 2 because 4 occurs twice in x. public static int getNumberEqual(int x[], int n, int val) { if (n< 0) ( return 0; } else { if (x[n-1) == val) { return getNumberEqual(x, n-1, val) +1; } else { return getNumber Equal(x, n-1, val); } // end if ) // end if } // end get Number Equal Demonstrate that this method is recursive by listing the criteria of a recursive solution and stating how the method meets each criterion.arrow_forward
- Refer to the following method that finds the smallest value in an array. /** Precondition: arr is initialized with int values. * Oparam arr the array to be processed Greturn the smallest value in arr public static int findMin(int[] arr) { int min = /* some value */; int index = 0; while (index < arr.length) { if (arr [index] < min) min = arr [index]; index++; } return min; } Which replacement(s) for /* some value */ will always result in correct execu- tion of the findMin method? I Integer.MIN_VALUE II Integer.MAX_VALUE III arr [0] (A) I only (B) II only (C) III only (D) I and III only (E) II and III onlyarrow_forwardProblem 11 of an Create a class ArraysAndMethodsGames, Write a method that returns the average array with the following header: public static double average(double[] array); Write a method to initialize the array with the following header: public static double[] readArray(): The readArray method prompts the user to enter ten double values and initializes a newly array with these values. It returns the array to main. The method main calls the method Method main prints the array as well. created average and prints the average. 8 Of 9 – SLCC, ASDV 1220, Lab17 Problem 12 Modify class ArraysAndMethodsGames by adding a method that finds the smallest element in an array of double values using the following header: public static double min(double[] array); Call it from main to test it with an array you create in main.arrow_forwardJava Netbeansarrow_forward
- Paragraph Styles Editing 5. Given the following series of n integers:1+2+3++n-2+n-1+nWrite a Java method that returns the sum of the series. 6. Given the following function:f(x)-2xFor, determine 7. Consider an array that stores the following sequence of integers:10, 15, 20, 25, 30Write a Java method that returns the sum of the integers stored in the array. The array must be passed to the method as an argument. I OFocus Earrow_forwardPYTHON: This exercise is a variation on "instrumenting" the recursive Fibonacci program to better understand its behavior. Write a supporting method that counts how many times the fib function is called to compute fib (n) where n is a user input. Hint: To solve this problem, you need an accumulator variable whose value "persists" between calls to fib. You can do this by making the count an instance variable of an object. Create a FibCounter class with the following methods: _init_(self) Creates a new FibCounter, setting its count instance variable to 0. getCount(self) Returns the value of count. fib(self, n) Recursive function to compute the nth Fibonacci number. It increments the count each time it is called. resetCount(self) Sets the count back to 0.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
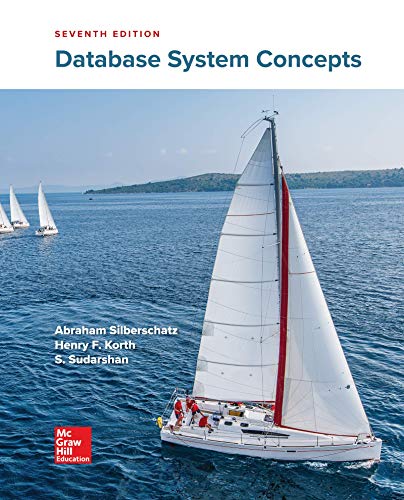
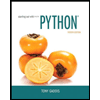
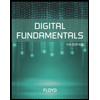
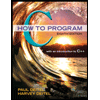
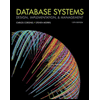
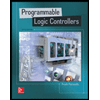