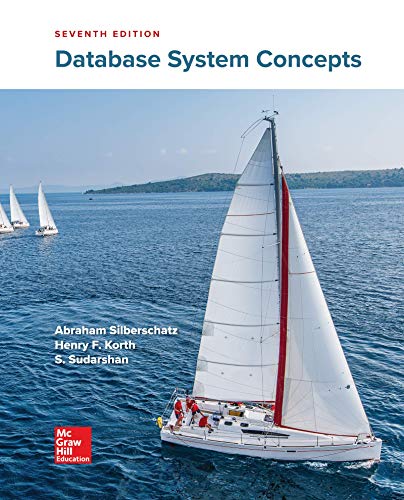
The following program rotates a cube with mouse clicks. In the display callback, the Lookat function is used to point the viewer, whose location can be altered by the x, X, y, Y, z and Z keys. The perspective view is set in the reshape callback. Create the code
/* cubeview.cpp */
#include <stdlib.h>
#include <GL/glut.h>
GLfloat vertices[][3] = {{-1.0,-1.0,-1.0},{1.0,-1.0,-1.0},
{1.0,1.0,-1.0}, {-1.0,1.0,-1.0}, {-1.0,-1.0,1.0},
{1.0,-1.0,1.0}, {1.0,1.0,1.0}, {-1.0,1.0,1.0}};
GLfloat normals[][3] = {{-1.0,-1.0,-1.0},{1.0,-1.0,-1.0},
{1.0,1.0,-1.0}, {-1.0,1.0,-1.0}, {-1.0,-1.0,1.0},
{1.0,-1.0,1.0}, {1.0,1.0,1.0}, {-1.0,1.0,1.0}};
GLfloat colors[][3] = {{0.0,0.0,0.0}, {0.0,0.0,1.0},
{0.0,1.0,0.0}, {0.0,1.0,1.0}, {1.0,0.0,0.0},
{1.0,0.0,1.0}, {1.0,1.0,0.0}, {1.0,1.0,1.0}};
void polygon(int a, int b, int c , int d)
{
glBegin(GL_POLYGON);
glColor3fv(colors[a]);
glNormal3fv(normals[a]);
glVertex3fv(vertices[a]);
glColor3fv(colors[b]);
glNormal3fv(normals[b]);
glVertex3fv(vertices[b]);
glColor3fv(colors[c]);
glNormal3fv(normals[c]);
glVertex3fv(vertices[c]);
glColor3fv(colors[d]);
glNormal3fv(normals[d]);
glVertex3fv(vertices[d]);
glEnd();
}
void colorcube()
{
polygon(0,3,2,1);
polygon(2,3,7,6);
polygon(0,4,7,3);
polygon(1,2,6,5);
polygon(4,5,6,7);
polygon(0,1,5,4);
}
static GLfloat theta[] = {0.0,0.0,0.0};
static GLint axis = 2;
static GLdouble viewer[]= {0.0, 0.0, 5.0}; /* initial viewer location */
void display()
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
/* Update viewer position in modelview matrix */
glLoadIdentity();
gluLookAt(viewer[0],viewer[1],viewer[2], 0.0, 0.0, 0.0, 0.0, 1.0, 0.0);
/* rotate cube */
glRotatef(theta[0], 1.0, 0.0, 0.0);
glRotatef(theta[1], 0.0, 1.0, 0.0);
glRotatef(theta[2], 0.0, 0.0, 1.0);
colorcube();
glFlush();
glutSwapBuffers();
}
void mouse(int btn, int state, int x, int y)
{
if(btn==GLUT_LEFT_BUTTON && state == GLUT_DOWN) axis = 0;
if(btn==GLUT_MIDDLE_BUTTON && state == GLUT_DOWN) axis = 1;
if(btn==GLUT_RIGHT_BUTTON && state == GLUT_DOWN) axis = 2;
theta[axis] += 2.0;
if( theta[axis] > 360.0 ) theta[axis] -= 360.0;
display();
}
void keys(unsigned char key, int x, int y)
{
/* Use x, X, y, Y, z, and Z keys to move viewer */
if(key == 'x') viewer[0]-= 1.0;
if(key == 'X') viewer[0]+= 1.0;
if(key == 'y') viewer[1]-= 1.0;
if(key == 'Y') viewer[1]+=1.0;
if(key == 'z') viewer[2]-= 1.0;
if(key == 'Z') viewer[2]+= 1.0;
display();
}
void myReshape(int w, int h)
{
glViewport(0, 0, w, h);
/* Use a perspective view */
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
if(w<=h)
glFrustum(-2.0, 2.0, -2.0 * (GLfloat) h/ (GLfloat) w,
2.0* (GLfloat) h / (GLfloat) w, 2.0, 20.0);
else
glFrustum(-2.0, 2.0, -2.0 * (GLfloat) w/ (GLfloat) h,
2.0* (GLfloat) w / (GLfloat) h, 2.0, 20.0);
glMatrixMode(GL_MODELVIEW);
}
int main(int argc, char **argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH);
glutInitWindowSize(500, 500);
glutCreateWindow("colorcube");
glutReshapeFunc(myReshape);
glutDisplayFunc(display);
glutMouseFunc(mouse);
glutKeyboardFunc(keys);
glEnable(GL_DEPTH_TEST);
glutMainLoop();
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- question in the image: let timerId = null; window.addEventListener("DOMContentLoaded", function() { document.addEventListener("click", startAnimation);}); function startAnimation(e) { // Get mouse coordinates let clickX = e.clientX; let clickY = e.clientY; // TODO: Modify the code below moveImage(clickX, clickY); } function moveImage(x, y) { const img = document.querySelector("img"); // Determine location of image let imgX = parseInt(img.style.left); let imgY = parseInt(img.style.top); // Determine (x,y) coordinates that center the image // around the clicked (x, y) coords const centerX = Math.round(x - (img.width / 2)); const centerY = Math.round(y - (img.height / 2)); // TODO: Add code here // Move 1 pixel in both directions toward the click if (imgX < centerX) { imgX++; } else if (imgX > centerX) { imgX--; } if (imgY < centerY) { imgY++; } else if (imgY > centerY) { imgY--;…arrow_forwardConnectedCircles.java, allows the userto create circles and determine whether they are connected. Rewrite the programfor rectangles. The program lets the user create a rectangle by clicking amouse in a blank area that is not currently covered by a rectangle. As the rectanglesare added, the rectangles are repainted as filled if they are connected orare unfilled otherwise, as shown in Figure b–c.arrow_forwardIn java plsarrow_forward
- Write a code to the following image using Console.WriteLine.arrow_forwardChapter 18 Problem 38.PE Textbook: Introduction to Java programming and datastructure. 11th Edition Y. Daniel Liang Publisher: PEARSON ISBN: 9780134670942 This code is taken from your website does not work. package e38; import javafx.application.Application;import javafx.event.ActionEvent;import javafx.event.EventHandler;import javafx.scene.Scene;import javafx.scene.control.Button; import javafx.stage.Stage;import javafx.geometry.Pos;import javafx.scene.control.Label;import javafx.scene.control.TextField;import javafx.scene.control.Textfield;import javafx.scene.layout.BorderPane;import javafx.scene.layout.HBox;import javafx.scene.layout.Pane;import javafx.scene.shape.Line;import javafx.stage.Stage; public class E38 extends Application { @Override public void start(Stage primaryStage) { // Create a pane TreePane tp = new TreePane(); // Create a textfield TextField tfOrder = new TextField(); // Set the Depth…arrow_forward21. Which properties will extend a grid item so that it covers multiple rows and columns, specifically the area from row gridlines 3 to 5 and from column gridlines 1 to 3? Group of answer choices a. grid-row: 3/5; grid-column: 1/3; b. grid-row: 2/4; grid-column: 4; c. grid-column-start: 3; grid-column-end: 5; d. grid-column-start-end: 1/3; grid-row-start-end: 3/5;arrow_forward
- Fix any error in this code import org.jfree.chart.ChartFactory;import org.jfree.chart.ChartPanel;import org.jfree.chart.JFreeChart;import org.jfree.data.category.DefaultCategoryDataset;import org.jfree.ui.ApplicationFrame;import org.jfree.ui.RefineryUtilities;public class BarChartExample extends ApplicationFrame {public BarChartExample(String title) {super(title);Object ChartFactory;JFreeChart barChart = ChartFactory.createBarChart("Referral Sources Count","Referral Source","Count",createDataset(),org.jfree.chart.plot.PlotOrientation.VERTICAL,true, true, false);ChartPanel chartPanel = new ChartPanel(barChart);chartPanel.setPreferredSize(new java.awt.Dimension(560, 367));setContentPane(chartPanel);}private DefaultCategoryDataset createDataset() {DefaultCategoryDataset dataset = new DefaultCategoryDataset();String series1 = "Referral Sources";dataset.addValue(250, series1, "Website");dataset.addValue(400, series1, "Word of Mouth");dataset.addValue(300, series1,…arrow_forwardIn the langauage R: Which of the following statements is NOT correct about themes in R? Select one: Once set, a theme applies to all subsequent plots and remains active until it is replaced by a different theme The theme_set() function takes the name of a theme as an argument If we want to change the overall look of the figure all at once, we can use ggplot’s theme engine The theme_minimal() function can make ggplot output look like it has been featured in the Wall Street Journalarrow_forwardCreate three students with the following details: Snow White, student ID: A00234,credits: 24 Lisa Simpson, student ID: C22044,credits: 56 Charlie Brown, student ID: A12003,credits: 6 Then enter all three into a lab and print a list to the screen.arrow_forward
- burses.projectstem.org/courses/64525/assignments/9460863?module_item_id=18079077 Maps HH 50 Follow these steps to create your Warhol Grid: 1. Find or create an image (for this activity, a smaller starting image will produce higher quality results in a shorter amount of time). 2. Using the Python documentation as a guide, create a program that loads the image, filters three copies of it, and saves the result. Note: You will need to create the three filters using the filter() method. • Include multiple filters on at least two of your variants. One of your variants must apply a single filter multiple times with the use of a loop. For example, the top right image above uses a loop to blur the image 2 times (what if we did it 100 times?). U ▪ One of your images must apply at least two different filters to the same image. In the example image, the bottom right image includes a filter to smooth the image and then edge enhance them. L • Create the Warhol Grid using Python with the three images…arrow_forwardUsing the following Triangle structure, declare a structure variable and initialize its vertices to (0,0), (5, 0), and (7,6): Triangle STRUCT Vertex1 COORD Vertex2 COORD Vertex3 COORD Triangle ENDS Declare an array of Triangle structures. Write a loop that initializes Vertex1 of each triangle to random coordinates in the range (0...10, 0...10).arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
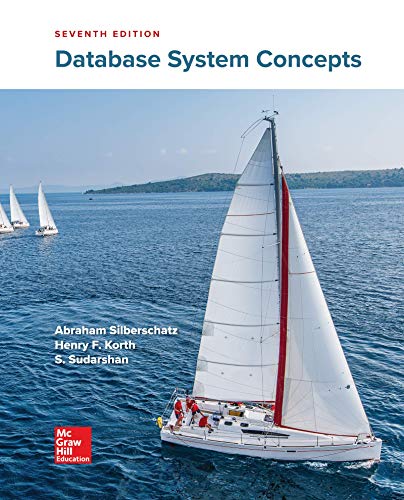
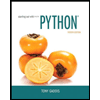
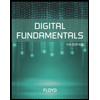
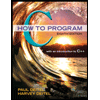
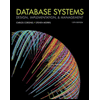
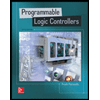