The following code is used to derive Fibonacci algorithm with this sequence: (0, 1, 1, 2, 3, 5, 8, 13, 21, …) where each number is “add” of two previous ones. int fib(int n){ if (n==0) return 0; else if (n == 1) return 1; else return fib(n−1) + fib(n−2); } Explain why the following RISC-V assembly code works, you need to explain all the details using the comments as hints? # IMPORTANT! Stack pointer must remain a multiple of 16!!!! 1. addi x10,x10,8 # fib(8), you can change it 2. fib: 3. beq x10, x0, done # If n==0, return 0 4. addi x5, x0, 1 5. beq x10, x5, done # If n==1, return 1 6. addi x2, x2, -16 # Allocate 2 words of stack space 7. sd x1, 0(x2) # Save the return address 8. sd x10, 8(x2) # Save the current n 9. addi x10, x10, -1 # x10 = n-1 10. jal x1, fib # fib(n-1) 11. ld x5, 8(x2) # Load old n from the stack 12. sd x10, 8(x2) # Push fib(n-1) onto the stack 13. addi x10, x5, -2 # x10 = n-2 14. jal x1, fib # Call fib(n-2) 15. ld x5, 8(x2) # x5 = fib(n-1) 16. add x10, x10, x5 # x10 = fib(n-1)+fib(n-2) 17. # Clean up: 18. ld x1, 0(x2) # Load saved return address 19. addi x2, x2, 16 # Pop two words from the stack 20. done: 21. jalr x0, 0(x1)
The following code is used to derive Fibonacci
(0, 1, 1, 2, 3, 5, 8, 13, 21, …) where each number is “add” of two previous ones.
int fib(int n){
if (n==0)
return 0;
else if (n == 1)
return 1;
else
return fib(n−1) + fib(n−2);
}
Explain why the following RISC-V assembly code works, you need to explain all the
details using the comments as hints?
# IMPORTANT! Stack pointer must remain a multiple of 16!!!!
1. addi x10,x10,8 # fib(8), you can change it
2. fib:
3. beq x10, x0, done # If n==0, return 0
4. addi x5, x0, 1
5. beq x10, x5, done # If n==1, return 1
6. addi x2, x2, -16 # Allocate 2 words of stack space
7. sd x1, 0(x2) # Save the return address
8. sd x10, 8(x2) # Save the current n
9. addi x10, x10, -1 # x10 = n-1
10. jal x1, fib # fib(n-1)
11. ld x5, 8(x2) # Load old n from the stack
12. sd x10, 8(x2) # Push fib(n-1) onto the stack
13. addi x10, x5, -2 # x10 = n-2
14. jal x1, fib # Call fib(n-2)
15. ld x5, 8(x2) # x5 = fib(n-1)
16. add x10, x10, x5 # x10 = fib(n-1)+fib(n-2)
17. # Clean up:
18. ld x1, 0(x2) # Load saved return address
19. addi x2, x2, 16 # Pop two words from the stack
20. done:
21. jalr x0, 0(x1)
Unlock instant AI solutions
Tap the button
to generate a solution
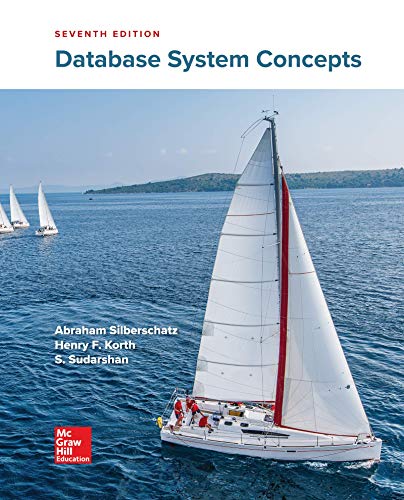
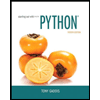
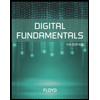
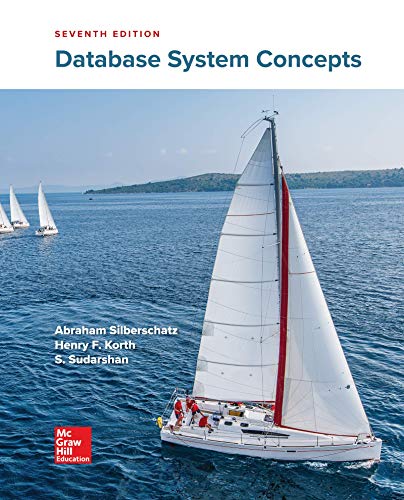
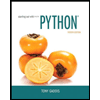
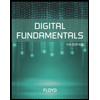
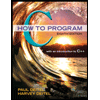
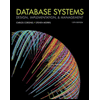
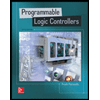