The following are the specifications of the member methods: 1. The print() method is used to print last name, first name and the computed wages with currency sign with the following sample output display: PART-TIME EMPLOYEE DETAILS Name: DELA CRUZ, JOHN S. Gross Pay Before Tax: $ $$$,$$$.$$ (Gross Pay Rank: 2 Step: 1) Gross Pay After Tax (Rated @ 11%): $ $$$,s$S.$$ ==== ====== Name: SANTOS, MARIA L. Gross Pay Before Tax: $ $$$,$$$.$$ (Gross Pay Rank:4 Step: 2) Gross Pay After Tax (Rated @ 11%): $ $$$,$$S.$$ ====== ======== (and so on ...) *** END-OF-PROGRAM RUN *** 2. The calculatePay) computes and returms the wages based on a Pay Scale Rate (See Table 1 below) 3. The setNameRateHour() with input parameters first, last, rate, and hours sets the first name, last name, payRate and hoursWorked properties. 4. The constructor for this class partTimeEmployees sets the first name, last name, payRate and hoursWorked according to the parameters when instantiated. When no value is specified, the default values are assumed. 5. Due to the Pay Scale schedule of Table 1, add two class properties: payStep and PayRank to be reflected in the computation of wages. 6. Wages are computed using Table 1 given the gross pay scale rank and step increment of any employee. Include also a corporate tax deduction rated at 11 %.
The following are the specifications of the member methods: 1. The print() method is used to print last name, first name and the computed wages with currency sign with the following sample output display: PART-TIME EMPLOYEE DETAILS Name: DELA CRUZ, JOHN S. Gross Pay Before Tax: $ $$$,$$$.$$ (Gross Pay Rank: 2 Step: 1) Gross Pay After Tax (Rated @ 11%): $ $$$,s$S.$$ ==== ====== Name: SANTOS, MARIA L. Gross Pay Before Tax: $ $$$,$$$.$$ (Gross Pay Rank:4 Step: 2) Gross Pay After Tax (Rated @ 11%): $ $$$,$$S.$$ ====== ======== (and so on ...) *** END-OF-PROGRAM RUN *** 2. The calculatePay) computes and returms the wages based on a Pay Scale Rate (See Table 1 below) 3. The setNameRateHour() with input parameters first, last, rate, and hours sets the first name, last name, payRate and hoursWorked properties. 4. The constructor for this class partTimeEmployees sets the first name, last name, payRate and hoursWorked according to the parameters when instantiated. When no value is specified, the default values are assumed. 5. Due to the Pay Scale schedule of Table 1, add two class properties: payStep and PayRank to be reflected in the computation of wages. 6. Wages are computed using Table 1 given the gross pay scale rank and step increment of any employee. Include also a corporate tax deduction rated at 11 %.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
c#

Transcribed Image Text:The following are the specifications of the member methods:
1. The print() method is used to print last name, first name and the computed wages with
currency sign with the following sample output display:
PART-TIME EMPLOYEE DETAILS
**
Name: DELA CRUZ, JOHN S.
Gross Pay Before Tax: $ $$$,$$$.$$
(Gross Pay Rank: 2 Step: 1)
Gross Pay After Tax (Rated @ 11%): $ $$$,s$S.$$
======
Name: SANTOS, MARIA L.
Gross Pay Before Tax: $ $$$,$$$.$$
(Gross Pay Rank: 4 Step: 2)
Gross Pay After Tax (Rated @ 11%): $ $$$,S$$.$$
===
====
(and so on...)
*** END-OF-PROGRAM RUN ***
2. The calculatePay() computes and returns the wages based on a Pay Scale Rate (See Table
1 below)
3. The setNameRateHour() with input parameters first, last, rate, and hours sets the first name,
last name, payRate and hoursWorked properties.
4. The constructor for this class partTimeEmployees sets the first name, last name, payRate and
hoursWorked according to the parameters when instantiated. When no value is specified, the
default values are assumed.
5. Due to the Pay Scale schedule of Table 1, add two class properties: payStep and PayRank
to be reflected in the computation of wages.
6. Wages are computed using Table 1 given the gross pay scale rank and step increment of any
employee. Include also a corporate tax deduction rated at 11 %.
Gross Pay By Rank ($)
4
3
2
1 115.00 215.00 342.50 510.00
2
125.00 232.50 360.00 620.00
3 145.00 245.00 380.00 740.00
4 167.50 267.05 405.00 850.00
190.00 290.00 440.00 990.00
Table 1. Schedule of wages without tax deduction.
Step

Transcribed Image Text:Suppose that you want to define a class to group the attributes of an employee. There are both
full-time and part-time employees. Part-time employees are paid based on the number of hours
worked and an hourly rate. Suppose that you want to define a class to keep track of a part-time
employee's information, such as name, pay rate, and hours worked. You can then print the
employee's name together with his or her wages. Because every employee is a person and Figure
10-11 defined the class personType to store the first name and the last name together with the
necessary operations on name, we can define a class partTimeEmployee (sub-class) based on
the class personType (base class). You can also redefine the print function to print the appropriate
information. Figure 11-5 shows the UML class diagram of the class partTimeEmployee and the
inheritance hierarchy.
partTimeEmployee
-payRate: double
-hoursWorked: double
+print () const: void
+calculatePay () const: double
+setNameRateHours (string, string,
personType
double, double): void
+partTimeEmployee (string = "", string = "",
double = 0)
double = 0,
partTimeEmployee
FIGURE 11-5 UML class diagram of the class partTimeEmployee and inheritance hierarchy
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
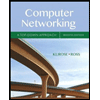
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
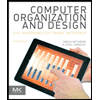
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
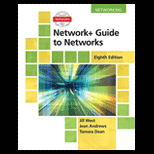
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
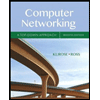
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
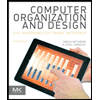
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
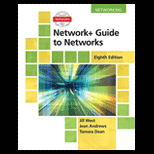
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
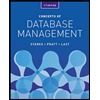
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
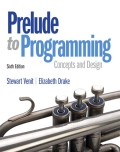
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
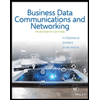
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY