ADD 2 STORE 0 LOAD 3 SUB 1 STORE 3 SKIP 0 JUMP 12 LOAD 0 OUT 7 END Output Specifications The virtual machine(VM) you are implementing should provide output according to the input file. Along with this output your program should provide status messages identifying details on the workings of your simulator. Output text does not have to reflect my example word-for-word, but please provide detail on the program as it runs in a readable format that does not conflict with the actual output of your VM. After each instruction print the current state of the Program Counter, Accumulator, and Data Memory. The INPUT instruction is the only one that should prompt an interaction from the user. Example: Reading Program... Program Loaded. Run. PC 10|A NULL | DM = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0] /* input value */ X PC 12|A=X|DM = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0] /* outputting accumulator to screen */ X PC 14|A=X | DM = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0] /* storing accumulator to memory location 0 */ PC 16|A X | DM = [X, 0, 0, 0, 0, 0, 0, 0, 0, 0] ... etc Program complete. Your program should compile and run from the command line with one input file parameter. For instance, to implement FETCH and instruction LOAD you must implement each step: FETCH MAR1 <-PC PC <- PC+2 MDR1 <- IM [MAR1] // IM stands for Instruction Memory (program memory) IR <-MDR1 Case IR.OP = 1 Load is executed. //LOAD (Execute cycle) MAR2 <-IR.ADDR MDR2 <- DM [MAR2] //DM stands for Data Memory A <-MDR2 Note: you can use MAR or MAR1 for IM and MAR2 for DM. Tiny Machine ISA: FETCH MARI <- PC PC <-PC+2 MDR1 <- IM [MAR] // IM stands for Instruction Memory (program memory) IR <-MDR1 Depending on IR.OP one of the following instructions will be executed: (Execute cycle) LOAD MAR2 <- IR.ADDR MDR2 <-DM[MAR2] A <- MDR2 ADD MAR2 <- IR.ADDR MDR2 <- DM[MAR2] A <-A+MDR2 STORE MAR2 <- IR.ADDR MDR2 <- A DM[MAR2] <- MDR2 SUB MAR2 <-IR.ADDR MDR2 <- DM[MAR2] A <- A - MDR2 IN A <- Input value from keyboard (emit a message to the user: "Input data:”) OUT Screen <-A (emit message to the user: "The result is:" END Run <- 0 // In your program Run must be initialized to 1 to control the instruction cycle. JMP PC <- IR.ADDR SKIP IF (A=0) PC PC + 1| THE CODE HAS TO MULTIPLY 2 NUMBERS TOGETHER. IF YOU DON'T KNOW HOE TO DO THIS PART PLEASE GIVE THE QUESTION TO SOMEONE ELSE. Using C programming language write a program that simulates a variant of the Tiny Harvard Architecture. In this implementation memory (RAM) is split into Instruction Memory (IM) and Data Memory (DM). Your code must implement the basic instruction set architecture (ISA) of the TinyMachine Architecture: 1 -> LOAD 2->ADD 3 ->STORE 4-> SUB 5->IN 6-> OUT 7 -> END 8 -> JMP 9 -> SKIPZ Each piece of the architecture must be accurately represented in your code (Instruction Register, Program Counter, Instruction Memory (IM), MAR1, MDR-1(MAR-1 and MDR-1 are connected to the IM). Data Memory, MAR-2, MDR2 (MAR-2 and MDR-2 are connected to the DM), and Accumulator. Instruction Memory will be represented by an integer array and each instruction will use 2 elements of the array(one for OP and the other one for address) Data Memory will be represented by an integer array and each data value uses an element of the DM array. Your Program Counter will begin pointing to the first instruction of the program (PC-0). For the sake of simplicity Instruction Memory (IM) and Data Memory (DM) may be implemented as separate integer arrays. IM size 250 DM size 10 Hint: All CPU registers and Data Memory (DM) are of type int. Input Specifications Your simulator must run from the command line with a single input file as a parameter to main. This file will contain a sequence of instructions for your simulator to store in "Instruction Memory" and then run via the fetch/execute cycle. In the input file each instruction is represented with two integers: the first one represents the opcode and the second one a memory address or a device number depending on the instruction. YOU MUST MAKE SURE THAT THE PROGRAM BEING RAN MULTIPLIES TWO NUMBERS. YOU CAN USE THE OBJECT FILE BELOW TO HELP WITH THIS. The numbers will not be validated. you can use two integers as inputs 3 and 4. The program must accept the input values in this order input1=0 (to accumulate the result here) input2=1 (top decrement variable that control the loop). input3: first number to be multiplied (for example, 5) input4: second number to be multiplied (for example, 3). Example of reading the object file and printing the Assembly language(this is what should be in the input file): Read Object File 55 67 30 55 67 31 55 67 32 55 67 33 10 22 30 13 41 33 90 812 10 67 70 What the object file above prints: Print it IN 5 OUT 7 STORE 0 IN 5 OUT 7 STORE 1 IN 5 OUT 7 STORE 2 IN 5 OUT 7 STORE 3 LOAD 0
THIS IS MY CODE SO FAR:
// header
#include <stdio.h>
// define the IM and DM size
#define IM_SIZE 250
#define DM_SIZE 10
// Define the ISA of the Tiny Machine Architecture
#define LOAD 1
#define ADD 2
#define STORE 3
#define SUB 4
#define IN 5
#define OUT 6
#define END 7
#define JMP 8
#define SKIPZ 9
// Accurately represented architecture
int PC = 0; // PC =
int IR; // IR = Instruction Register
int MAR1, MAR2; // MAR1,MAR2 = Memory Address Registers 1 and 2
int MDR1, MDR2; // MDR1,MDR2 = Memory Data Registers 1 and 2
int A = 0; // A = Accumulator
int IM[IM_SIZE]; // IM = Instruction Memory
int DM[DM_SIZE]; // DM = Data Memory
// opening the file
void openProgram(const char* filename) {
FILE* file = fopen(filename, "r");
if (file == NULL) {
printf("Error opening file.\n");
return;
}
int opcode, address;
int i = 0;
while (fscanf(file, "%d %d", &opcode, &address) == 2 && i < IM_SIZE) {
IM[i++] = opcode;
IM[i++] = address;
}
fclose(file);
}
// execute the instructions
void execute() {
int run = 1;
while (run) {
// Fetch
MAR1 = PC;
PC = PC + 2;
MDR1 = IM[MAR1];
IR = MDR1;
// use a switch to help carry out the instructions
switch (MDR1) {
case LOAD:
// LOAD
MAR2 = IR;
MDR2 = DM[MAR2];
A = MDR2;
break;
case ADD:
// ADD
MAR2 = IR;
MDR2 = DM[MAR2];
A = A + MDR2;
break;
case STORE:
// STORE
MAR2 = IR;
MDR2 = A;
DM[MAR2] = MDR2;
break;
case SUB:
// SUB
MAR2 = IR;
MDR2 = DM[MAR2];
A = A - MDR2;
break;
case IN:
// IN
printf("Input data: ");
scanf("%d", &A);
break;
case OUT:
// OUT
printf("The result is: %d\n", A);
break;
case END:
// END
run = 0;
break;
case JMP:
// JMP
PC = IR;
break;
case SKIPZ:
// SKIPZ
if (A == 0)
PC++;
break;
default:
printf("Invalid opcode encountered.\n");
run = 0;
}
// Print the current state after each instruction
printf("PC = %d | A = %d | DM = [", PC, A);
for (int i = 0; i < DM_SIZE; i++) {
printf("%d", DM[i]);
if (i < DM_SIZE - 1)
printf(", ");
}
printf("]\n");
}
printf("Program complete.\n");
}
// main method
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: %s <filename>\n", argv[0]);
return 1;
}
openProgram(argv[1]);
printf("Reading Program... Program Loaded. Run.\n");
execute();
return 0;
}
the elf file:
5 5
6 7
3 0
5 5
6 7
3 1
5 5
6 7
3 2
5 5
6 7
3 3
1 0
2 2
3 0
1 3
4 1
3 3
9 0
8 12
1 0
6 7
7 0
I JUST NEED HELP FIGURING OUT HOW TO MULTIPLY THE TWO INPUT VALUES PLEASE HELP.
![ADD 2
STORE 0
LOAD 3
SUB 1
STORE 3
SKIP 0
JUMP 12
LOAD 0
OUT 7
END
Output Specifications
The virtual machine(VM) you are implementing should provide output according to the input file. Along with this output your program should provide status messages identifying details on the workings of your simulator. Output text does not have to reflect my example word-for-word, but please provide detail on the program
as it runs in a readable format that does not conflict with the actual output of your VM. After each instruction print the current state of the Program Counter, Accumulator, and Data Memory. The INPUT instruction is the only one that should prompt an interaction from the user.
Example:
Reading Program...
Program Loaded.
Run.
PC 10|A NULL | DM = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
/* input value */
X
PC
12|A=X|DM = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
/* outputting accumulator to screen */
X
PC
14|A=X | DM = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
/* storing accumulator to memory location 0 */
PC 16|A X | DM = [X, 0, 0, 0, 0, 0, 0, 0, 0, 0]
... etc
Program complete.
Your program should compile and run from the command line with one input file parameter.
For instance, to implement FETCH and instruction LOAD you must implement each step:
FETCH
MAR1 <-PC
PC <- PC+2
MDR1 <- IM [MAR1] // IM stands for Instruction Memory (program memory)
IR <-MDR1
Case IR.OP = 1 Load is executed.
//LOAD (Execute cycle)
MAR2 <-IR.ADDR
MDR2 <- DM [MAR2] //DM stands for Data Memory
A <-MDR2
Note: you can use MAR or MAR1 for IM and MAR2 for DM.
Tiny Machine ISA:
FETCH
MARI <- PC
PC <-PC+2
MDR1 <- IM [MAR] // IM stands for Instruction Memory (program memory)
IR <-MDR1
Depending on IR.OP one of the following instructions will be executed:
(Execute cycle)
LOAD
MAR2 <- IR.ADDR
MDR2 <-DM[MAR2]
A <- MDR2
ADD
MAR2 <- IR.ADDR
MDR2 <- DM[MAR2]
A <-A+MDR2
STORE
MAR2 <- IR.ADDR
MDR2 <- A
DM[MAR2] <- MDR2
SUB
MAR2 <-IR.ADDR
MDR2 <- DM[MAR2]
A <- A - MDR2
IN
A <- Input value from keyboard (emit a message to the user: "Input data:”)
OUT
Screen <-A (emit message to the user: "The result is:"
END
Run <- 0 // In your program Run must be initialized to 1 to control the instruction cycle.
JMP
PC <- IR.ADDR
SKIP
IF (A=0) PC PC + 1|](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F44870f31-83c6-452e-ba54-77702704a3ca%2Febaccba4-44b0-4645-a139-dcd87b942a76%2Fx2rdq0c_processed.png&w=3840&q=75)
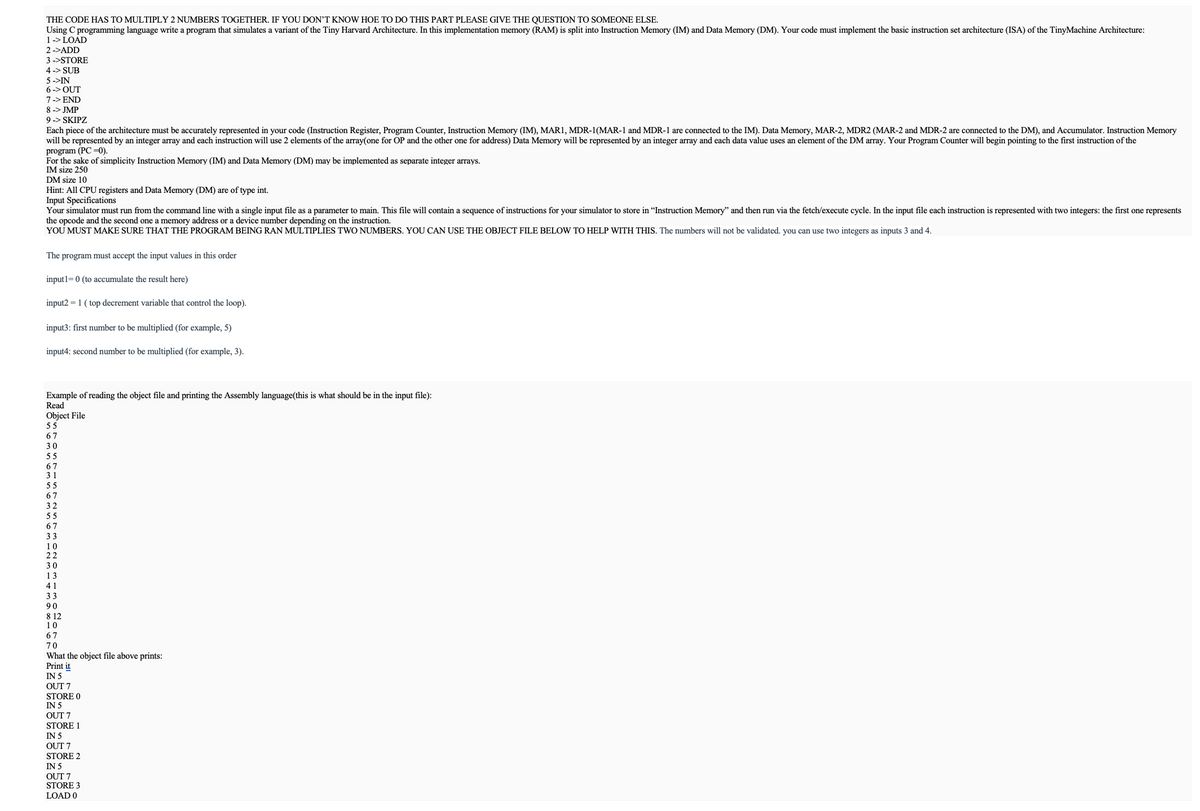

Step by step
Solved in 2 steps

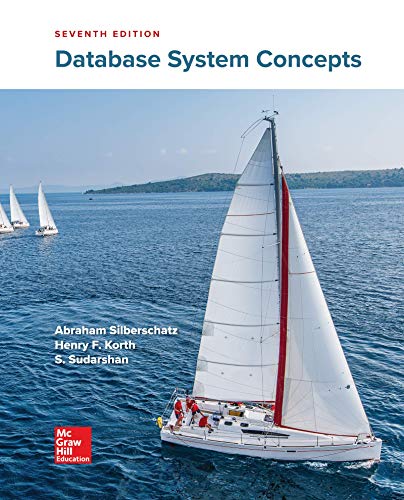
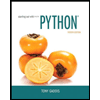
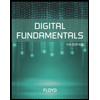
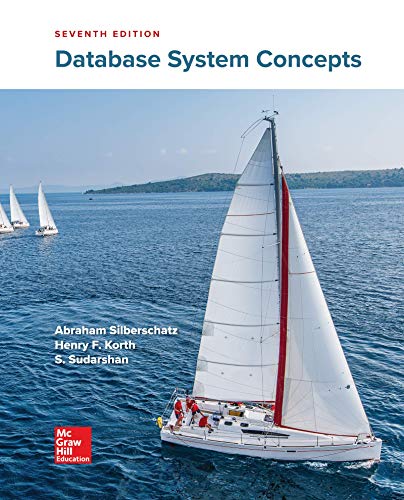
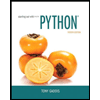
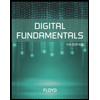
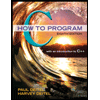
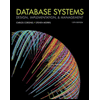
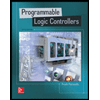