The 'checkout' method prints a receipt for the cart contents. It prints a title line showing the customer name and cart number, then iterates through the cart dictionary and prints a line for each item (name, quantity, and extended price), and a total line at the bottom showing the total cost for all items in the cart. Here's an example: Checkout for Mary Smith Cart# 1 $ $ 3.98 $ 9.50 milk 1 3.99 2 eggs salmon 1 Total $ 17.47
The 'checkout' method prints a receipt for the cart contents. It prints a title line showing the customer name and cart number, then iterates through the cart dictionary and prints a line for each item (name, quantity, and extended price), and a total line at the bottom showing the total cost for all items in the cart. Here's an example: Checkout for Mary Smith Cart# 1 $ $ 3.98 $ 9.50 milk 1 3.99 2 eggs salmon 1 Total $ 17.47
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
follow the instructions and this is the test script to follow:

Transcribed Image Text:In this assignment you are to create a class called Cart that will model the operation of an on-line
store shopping cart. A Cart object, once created, will hold items selected by an on-line shopper.
When the shopper is finished, the Cart object will be used to perform a checkout for the shopper.
The Cart class is to be created with the following methods and attributes:
Method
Purpose
Input
Output/returns
Name
Constructor - sets the customer name for
the cart and maintains a cart sequence
number. The first cart is #1, the second is
#2, etc.
If the object passed is not type 'Item',
return False and 'not an Item type'
One Cart class
object
init
The name of the
shopper or
customer
(type string)
One item object
addltem
True plus 'added'
or
False plus 'not an
Item type'
If the item is not in the Cart, add the object
and the quantity of 1, return True and
'added'.
If the item is already in the Cart, add 1 to
the quantity, return True and 'added'
checkout
Print a heading line for the receipt,
including the customer name and cart
None
Prints a checkout
receipt for the
Cart, including
customer name,
number.
Iterate through the Cart contents, print
each item, the quantity, and extended
price (qty x unit price).
Print a total line at the end with the total
cart number, each
item qty, and
price, and total
price
price for all items in the cart.
Attribute
Туре
Definition (note: double underscore protection is not needed)
The name of the customer shopper, passed to the Cart constructor
when the Cart object is created
Sequence number for the Cart object – starts as 1 for cart #1, then 2,
3, etc. This is not an input, but is maintained by the Cart class.
Holds the contents of the cart. The key is the name of the Item
object added, which will be supplied by the Item object (i.e.
item_object.name). The corresponding value is a list with two things:
(1) the quantity of that particular item in the cart (integer), and (2) the
object id of the Item being added.
custName
str
seqNo
int
contents
dict
A simple Item class definition is provided with the assignment for you to use to hold items. It only has
a constructor method and two attributes: name (str) and price (float). 'name' is used as the dictionary
key inside cart. 'price' is the unit price for one of the items (i.e. 1.99 is the cost of one carton of eggs).
The most difficult part of this Lab is creating and using the self.contents dictionary inside the Cart
![class. The constructor only creates a blank dictionary when the Cart object is created. The 'addltem'
and 'checkout' methods need to maintain and use it.
As an example, when item objects i1 ('milk', 3.99), i2 ('eggs', 1.99), and i3 ('wine', 9.50) are added,
the dictionary contents will be:
self.contents = { 'milk': [1, <i1 object id>], 'eggs': [1, <i2 object id>], 'wine': [1, <i3 object id>] }
The 'addltem' method checked to see if the item name is in the dictionary. If not, it adds each of the
above to the dictionary.
If Item object i4 ('eggs', 1.99) is then added, the dictionary becomes:
self.contents = { 'milk': [1, <i1 object id>], 'eggs': [2, <i2 object id>], 'wine': [1, <i3 object id>] }
In this case the 'addltem' method found that 'eggs' was already a key in the dictionary and only
updated the quantity by adding one. The 'object id' did not need to be updated since it is a duplicate
of the one previously added. Note that items can only be added to the Cart one at a time. There is
no provision for adding 2, 3, or more of a given item at once.
The 'checkout' method prints a receipt for the cart contents. It prints a title line showing the customer
name and cart number, then iterates through the cart dictionary and prints a line for each item (name,
quantity, and extended price), and a total line at the bottom showing the total cost for all items in the
cart. Here's an example:
Checkout for Mary Smith
Cart# 1
milk
$
$
$ 9.50
1
3.99
2
3.98
eggs
salmon
1
Total
$ 17.47](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9eb2eb60-aea6-4c2b-bdb3-b0b3ca45de12%2F35a39c69-1120-4460-ad53-88eebffe4b4f%2Fr5qr60c_processed.png&w=3840&q=75)
Transcribed Image Text:class. The constructor only creates a blank dictionary when the Cart object is created. The 'addltem'
and 'checkout' methods need to maintain and use it.
As an example, when item objects i1 ('milk', 3.99), i2 ('eggs', 1.99), and i3 ('wine', 9.50) are added,
the dictionary contents will be:
self.contents = { 'milk': [1, <i1 object id>], 'eggs': [1, <i2 object id>], 'wine': [1, <i3 object id>] }
The 'addltem' method checked to see if the item name is in the dictionary. If not, it adds each of the
above to the dictionary.
If Item object i4 ('eggs', 1.99) is then added, the dictionary becomes:
self.contents = { 'milk': [1, <i1 object id>], 'eggs': [2, <i2 object id>], 'wine': [1, <i3 object id>] }
In this case the 'addltem' method found that 'eggs' was already a key in the dictionary and only
updated the quantity by adding one. The 'object id' did not need to be updated since it is a duplicate
of the one previously added. Note that items can only be added to the Cart one at a time. There is
no provision for adding 2, 3, or more of a given item at once.
The 'checkout' method prints a receipt for the cart contents. It prints a title line showing the customer
name and cart number, then iterates through the cart dictionary and prints a line for each item (name,
quantity, and extended price), and a total line at the bottom showing the total cost for all items in the
cart. Here's an example:
Checkout for Mary Smith
Cart# 1
milk
$
$
$ 9.50
1
3.99
2
3.98
eggs
salmon
1
Total
$ 17.47
Expert Solution

Step 1
Answers
The Python Code for the given problem is:
# class Item
class Item:
def __init__(self, name, price) -> None:
# constructor with item name and price
self.name = name
self.price = price
class Cart:
# static variable to store count of carts
counter = 1
# constructor of cart, takes customer name and initialize members
def __init__(self, customer_name) -> None:
self.custName = customer_name
self.seqNo = '# ' + str(Cart.counter)
self.contents = {}
Cart.counter = Cart.counter + 1
# add anitem to contents dictionary
def addItem(self, anItem):
# check if anItem is of type Item, else return false
if not isinstance(anItem, Item):
return False, 'not an item type'
# if item is not in dictionary already, add it with quantity 1
# else increase the quantity of item by 1
if anItem.name not in self.contents.keys():
self.contents[anItem.name] = [1, anItem]
else:
self.contents[anItem.name][0] = self.contents[anItem.name][0] + 1
return True, 'added'
# prints the reciept of cart
def checkout(self):
total = 0
print('Checkout for %s \tCart %s\n'%(self.custName, self.seqNo))
for key, value in self.contents.items():
print("%s \t\t\t%d\t$\t%.2f\n"%(key, value[0], value[1].price * value[0]))
total = total + (value[0] * value[1].price)
print("Total \t\t\t\t$\t%.2f\n"%(total))
# test data
item1 = Item('milk', 3.99)
item2 = Item('eggs', 1.99)
item3 = Item('salmon', 9.50)
aCart = Cart('Marry Smith')
aCart.addItem(item1)
aCart.addItem(item2)
aCart.addItem(item2)
aCart.addItem(item3)
aCart.checkout()
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
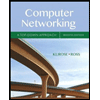
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
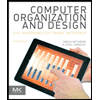
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
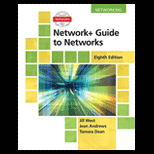
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
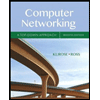
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
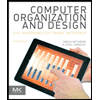
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
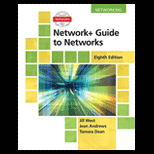
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
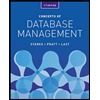
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
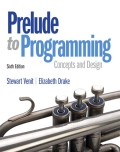
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
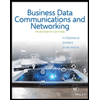
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY