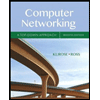
Create a MATLAB function that can select the closest site from a list of other sites and also return the distance to that site. The function should report the distance to that site as well (L).
function [selected_site, L] = select_nearest_site(present_site, list_of_sites, x, y)
Example:
we have a list of four sites given to us:
- x = [2, 4, 1, 5]
- y = [3, 6, 5, 8]
present_site = 4
list_of_sites = [1, 2, 3] (Note: this essentially means, find me which of the 1st, 2nd, and 3rd site is closest to the present_site (4) where you use information about their positions stored in x and y)
L = distance
Example of use:
- x = [2, 4, 1, 5]
- y = [3, 6, 5, 8]
- present_site = 4
- list_of_sites = [1, 2, 3]
[selected_site, L] = select_nearest_site(4, [1 2 3], x, y)
ans:
selected_site
= 1
L
= 1.732
I've just included down below the function that I used to calculate the distances, that should be used to find L. This function calculates the distances from the point (x_c, y_c) - are the chosen points; to all the points provided by the
x_c and y_c is the chosen point.
- given location (2, -1) - this is x_c and y_c
- x = [0, 2, 3]
- y = [3, 2, 4]

Step by stepSolved in 3 steps with 2 images

- Write a MATLAB function should take as parameters an unsorted list of non-negative integers, and an integer value, and returns the index where that value is first located in the list. (No argument testing is required.) Your MATLAB function should return the value -1 if the value is not found.arrow_forwardIn Python Each image-processing function that modifies its image argument has the same loop pattern for traversing the image. The only thing that varies is the code used to change each pixel within the loop. The topic of higher-order functions, discussed in chapter 6, suggests a simpler design pattern for such code. Design a single function, named transform, which expects an image and a function as arguments. When this function is called, it should be passed by another function that expects a tuple of integers and returns a tuple of integers. This is the function that transforms the information for an individual pixel (such as converting it to black and white or gray-scale) The transform function contains the loop logic for traversing its image argument. In the body of the loop, the transformfunction accesses the pixel at the current position, passes it as an argument to the other function, and resets the pixel in the image to the function’s value. Write and test a script that…arrow_forwardWhich of the following is the definition of a higher-order function? func xs = sum (map (^2) xs) func x = x * 2 func (x,y) = (y,x) func (x:y:zs) = y func f [x,y] = [f x, f y]arrow_forward
- Finally, we will do some simple analysis - ranking of words. Write a function word_ranking(corpus, n, sort_index) which takes input parameters corpus (type list), n (type int), and sort_index (type int) and returns a list containing tuples of words and their frequencies in the top n of words. Here, corpus is a list of sentences. n is the number of ranked words to return. The resulting list should then be sorted based on the sort_index; sort_index = should sort the list of tuples in ascending alphabetical order, while sort_index = 1 should sort the list of tuples by the frequencies in descending order. All other numeric values for sort_index should return an empty list (don't need to consider non- integer inputs). For example, we have: corpus = ['hi hi hi hi', 'hello hello say bye', 'bye bye'] n = 3 sort_index = 1 The function should return: [('hi', 4), ('bye', 3), ('hello', 2)] While sort_index = 0 should return: [('bye', 3), ('hello', 2), ('hi', 4)] You can assume n would be selected…arrow_forwardFinally, we will do some simple analysis – ranking of words. Write a function word_ranking(corpus, n, sort_index) which takes input parameters corpus (type list), n (type int), and sort_index (type int) and returns a list containing tuples of words and their frequencies in the top n of words. Here, corpus is a list of sentences. n is the number of ranked words to return. The resulting list should then be sorted based on the sort_index; sort_index = 0 should sort the list of tuples in ascending alphabetical order, while sort_index = 1 should sort the list of tuples by the frequencies in descending order. All other numeric values for sort_index should return an empty list (don't need to consider non-integer inputs). For example, we have: corpus = ['hi hi hi hi', 'hello hello say bye', 'bye bye']n = 3sort_index = 1 The function should return: [('hi', 4), ('bye', 3), ('hello', 2)] While sort_index = 0 should return: [('bye', 3), ('hello', 2), ('hi', 4)] You can assume n would…arrow_forwardAnswer the following questin in datail in JS and with opps conecptsarrow_forward
- Write the MATLAB commands that assign 33 and 4 to the variables a and b, respectively. Then, create a column vector W with the elements а! In(ab) Vb - a V(a + b)² – (b – a)? -a b where y! is the factorial of y, for which you should use a built-in MATLAB function, and In(x) is the natural logarithm of x. Finally, compute the average of the elements in W.arrow_forwardComputer Science Use the trees dataset (which is pre-loaded in R, under the name trees). This data set provides measurements of the girth (inches), height (feet) and volume (cubic feet) of timber in 31 felled black cherry trees. # Use R to work out the predicted height (in feet) for a tree of girth 30 inches.arrow_forwardIn mathematics, the triangle inequality states that for any triangle, the sum of the lengths of any two sides must be greater than or equal to the length of the remaining side. Write a function named, prg_question_1 to check whether three sides of given lengths would form a triangle or not. For example, for sides 12.5, 20.8, 15.0 your function must return true whereas for 4.8, 3.0, 12.0 it must return false as 4.8 + 3.0 is < 12. Do the following: 1. Follow UMPIRE process to get the algorithm. Write only the algorithm as code comments 2. Implement your function 3. Test your function for the sets of side lengths of units 10, 20, 15 and 4, 4, 10. Please follow the test criteria given in the code templatearrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
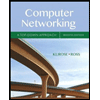
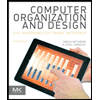
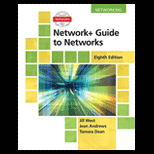
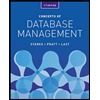
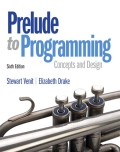
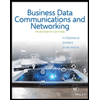