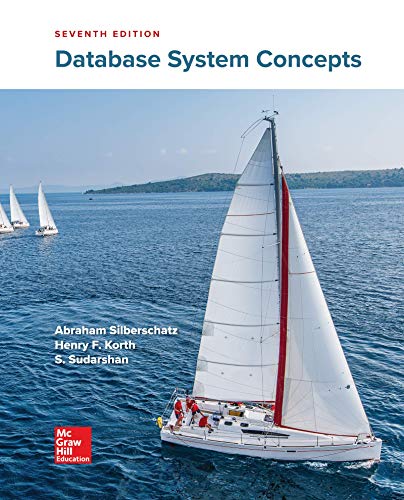
Concept explainers
Suppose you are given a `Employee` class with instance variables `name`, `age`,
`department, and salary. You are also given a static variable "total Employees and a static variable `totalSalary`, which respectively keep track of the total number of employees and the total salary of all employees.
Using this class, write a Java program that does the following:
1. Create an array of size 3 to store Employee objects.
2. Initialize the array with the following Employee` objects:
• Name: Alice, Age: 25, Department: HR, Salary: 50,000
Name: Bob, Age: 35, Department: Marketing, Salary: 60,000
Name: Charlie, Age: 30, Department: Sales, Salary: 55,000
3. Modify the age of the first employee in the array to 26.
4. Modify the salary of the second employee in the array to 65,000.
5. Print out the total number of employees and the average salary of all employees.
6. Use the static method `findEmployeeByName to find the `Employee` object with the name "Alice", and print out the `toString()` representation of this object.
7. Use the instance method `isolderThan` to determine if the first employee in the array is older than 30. Print out the result of this determination.
8. Use the instance method `promote` to give a raise of 10,000 to the first employee in
the array, and print out the new salary of this employee.
Your program should output the following:
csharp
Copy code
Total number of employees: 31
Average salary: 55000.0
Employee
found by
Employee 1 is
Employee [name='Alice',
age=26,
department='HR',
sala
Employee
older than
30: false
60000.0
salary:
1 new
name:

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Rename the show method to people Override the show method in student and in the other class you created (this will be the polymorphic method) In main program, create an array of 3 objects of class people Instantiate each array position with a different object (people, student, other) With a loop, display all the objects in the array.arrow_forwardChapter 7. PC #1. Rainfall Class Write a RainFall class that stores the total rainfall for each of 12 months into an array of doubles. The program should have methods that return the following: • the total rainfall for the year • the average monthly rainfall • the month with the most rain • the month with the least rain Demonstrate the class in a complete program. Main class name: RainFall (no package name) PLEASE MODIFY THIS CODE SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES THE TEST CASES. IT HAS TO PASS THE TEST CASES BECAUSE WHEN I UPLOAD IT TO HYPERGRADE IT DOES NOT PASS THE TEST CASES. I HAVE PROVIDED PROOF OF THE PART OF THE TEST CASE THAT FAILED. import java.util.Scanner;public class Rainfall{ double rain[]; public Rainfall() { rain = new double[12]; } public double averageRainfall() { return getTotalRain()/12.0; } public double getTotalRain() { double total = 0.0; for(int i=0;i<12;i++)…arrow_forwardWrite a Java program (Hangman.java) to play the classic word game Hangman. In the game, a word is randomly selected from an array of possible words. Then, the user is prompted to guess letters in the word one at a time. When the user makes a correct guess, all instances of that letter in the word are shown. When all letters in the word have been guessed, the game ends and the score (number of missed guesses) is displayed. A user can play the game multiple times if they choose. Here is a sample run of a correct program (user input indicated by orange text):Enter a letter in word ******** > cEnter a letter in word c******* > rEnter a letter in word c******r > s s is not in the wordEnter a letter in word c******r > tEnter a letter in word c****t*r > mEnter a letter in word c*m**t*r > t t is already in the wordEnter a letter in word c*m**t*r > pEnter a letter in word c*mp*t*r > oEnter a letter in word comp*t*r > eEnter a letter in word comp*ter >…arrow_forward
- This is in Java. The assignement is to create a class. I am confused on how to write de code for an array that is up to 5. Also I am confused regarding how to meet the requirements of the constructor. This is what I have. public class InventoryOnShelf { //fields private int[] itemOnShelfList []; private int size; public InventoryOnShelf() { }arrow_forwardWrite an application (including algorithm) that uses a class called “Book” (seedetails below). The application must perform the following tasks. Create an array of “Book” objects (i.e., array must be able to store 2books). Create 2 “Book” objects initialized as follows.o title = “C++ Textbook”; Author = “Pete Parker”; Publisher =“Cengage”, ISBN = “0123456789”; subject = “Computer Programming”;o title = “Java Textbook”; Author = “Bruce Wayne”; Publisher = “McGrawHill”, ISBN = “9876543210”; subject = “Computer Programming”; Add the two books to the array.o Assume the two publishers have merged into a new company called“BookConglomerate”. Use a loop to create statements to modify thepublisher field with the new publisher name. Display the following information for both bookso Book Title (i.e., “C++ Textbook” or “Java Textbook”)o Authoro Publishero ISBNo SubjectThe Book class must contain the following. Constructor with parameters that initialize all instance variables title, author,…arrow_forwardThis question is in javaYou are walking along a hiking trail. On this hiking trail, there is elevation marker at every kilometer. The elevation information is represented in an array of integers. For example, if the elevation array is [100, 50, 20, 30, 50, 40], that means at kilometer 0, the elevation is 100 meters; at kilometer 1, the elevation is 50 meters; at kilometer 2, the elevation is 20 meters; at kilometer 3, the elevation is 30 meters; at kilometer 4, the elevation is 50 meters; at kilometer 5, the elevation is 40 meters. a) Write a method called dangerousDescent that determines whether there is a downhill section in the hiking trail with a slope of more than -0.05. The slope is calculated by the rise in elevation over the run in distance. For example, the last kilometer section has a gradient of -0.01 because the rise in elevation is 40 - 50 = -10 meters. The distance covered in 1000 meters. -10 / 1000 = 0.01. Your method should return true if there exists a dangerous…arrow_forward
- Write a program that uses two classes. The first class is called “dAta” and holds x and y coordinates of a point in 2-d space called p1. The second class is called “cOmpute” and holds an array of two pointers to the “dAta” class. The “cOmpute” class has a function, lOad(float x, float y, int n), which loads x and y coordinate data into the array at index n. It also has a function, sLope(), which computes the slope of the line connecting the two array data coordiantes. It also has a function, pRint(), which prints the slope result to the screen. Implement a divide by zero exception using throw in the sLope() nd/or pRint() function as appropriate. The exception should, when caught, print “Slope calculation error…” to the screen and exit the function. The program should load the array with some example points and print the slope to the screen using the class functions.arrow_forwardIn C# Use a one-dimensional array to solve the following problem. Write a class called ScoreFinder. This class receives a single dimensional integer array representing passer ratings for NFL players as an argument for its constructor. The test class will provides this argument. The test class then calls upon a method from the ScoreFinder class to start the process of finding a specific rating, which will be provided by the user. The method will iterate through the array and find any matching values. For all the matches that are found, the method will note the index of the cell along with its value. At completion, the method will print a list of all the indices and the associated scores along with a count of all the matching values.arrow_forwardIn Java.arrow_forward
- Write a class AnalyzeNumbers which asks the user to enter the number of inputs andstores them in an Array, then prints the Array that was inputted, calculates the sum ofthe numbers entered and prints the total numbers greater than the first inputtednumber: Two Sample RunsEnter the number of items: 5Enter the numbers: 3.4 5 6 7.1 9You inputted: 3.4 5.0 6.0 7.1 9.0Sum is: 30.5Number of elements greater than the first element: 4 Enter the number of items: 3Enter the numbers: 6.7 1.5 3.2You inputted: 6.7 1.5 3.2Sum is: 11.4Number of elements greater than the first element: 0arrow_forwardIn C++ Create a class called Student. Each student should have a name and ID number (an int). They should also have a ptr to a dynamic array called Grades. The public methods for Student should be: getName, setName, getID, setID, setGrades, getGrades. In the constructor you should initialize Grades to be an array of size 4 with the values 0 for each position. Make sure that you use THIS properly. Create 2 students, assign one object to another and show that when you assign grades to one it effects the other equally.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
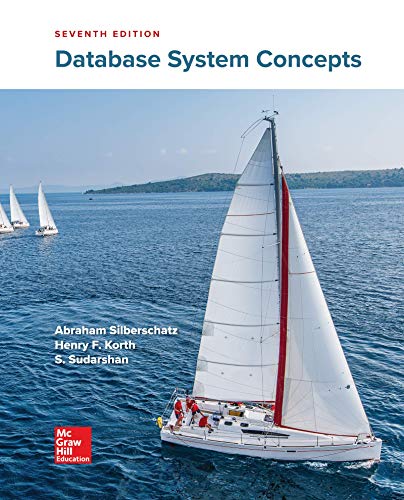
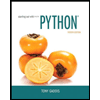
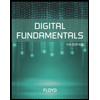
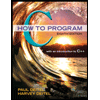
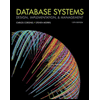
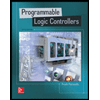