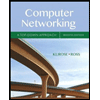
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Please help with C++ hashing question in C++ language. Sample output also in image. Thank you.
![Suppose that we have a hash table `data[CAPACITY]`. The hash table uses open addressing with quadratic probing. The table size is a global constant called `CAPACITY`. Locations of the table that have never been used will contain the key `-1`. All valid keys will be non-negative, and the hash function is:
```c
int hash_function(int key) {
return (key % CAPACITY);
}
```
Complete the implementation of the following functions. There is no need to check the precondition, but your code must be as efficient as possible.
**Implement the following functions:**
```c
const int CAPACITY = 10;
void initTable(int data[]); // data[CAPACITY] = {-1, -1, ..., -1}
void printTable(int data[]);
int hash_function(int key);
void hashInsert(int data[], int key);
bool key_occurs(int data[], int search_key);
```
**Part 1: Insert keys {10, 22, 11, 31, 24, 88, 38, 21} into an empty hash table with `CAPACITY = 10` using quadratic probing (`c1=0` and `c2=1`) to resolve collision (You shouldn't use a for/while loop except to increment the value of `i` for quadratic probing).**
**Part 2: Check whether the following numbers (11, 31, and 23) are in the table. (You shouldn't use a for/while loop except to increment the value of `i` for quadratic probing).**](https://content.bartleby.com/qna-images/question/620c60a1-10fe-416e-8b85-1e75a34b227e/e1b04271-6df5-4e79-a113-b8cfb7927e83/gxnhamn_thumbnail.png)
Transcribed Image Text:Suppose that we have a hash table `data[CAPACITY]`. The hash table uses open addressing with quadratic probing. The table size is a global constant called `CAPACITY`. Locations of the table that have never been used will contain the key `-1`. All valid keys will be non-negative, and the hash function is:
```c
int hash_function(int key) {
return (key % CAPACITY);
}
```
Complete the implementation of the following functions. There is no need to check the precondition, but your code must be as efficient as possible.
**Implement the following functions:**
```c
const int CAPACITY = 10;
void initTable(int data[]); // data[CAPACITY] = {-1, -1, ..., -1}
void printTable(int data[]);
int hash_function(int key);
void hashInsert(int data[], int key);
bool key_occurs(int data[], int search_key);
```
**Part 1: Insert keys {10, 22, 11, 31, 24, 88, 38, 21} into an empty hash table with `CAPACITY = 10` using quadratic probing (`c1=0` and `c2=1`) to resolve collision (You shouldn't use a for/while loop except to increment the value of `i` for quadratic probing).**
**Part 2: Check whether the following numbers (11, 31, and 23) are in the table. (You shouldn't use a for/while loop except to increment the value of `i` for quadratic probing).**
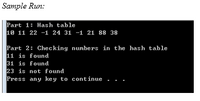
Transcribed Image Text:**Sample Run:**
**Part 1: Hash table**
10 11 22 -1 24 31 -1 21 88 38
**Part 2: Checking numbers in the hash table**
11 is found
31 is found
23 is not found
Press any key to continue . . .
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

Knowledge Booster
Similar questions
- Which header file is necessary for C++ OOP? What attribute permits open recursion?arrow_forwardWhat is a string in c-programming? Give the Importance of strings in c-programming, types of strings in c, and give an example of a program code with strings that can be executed.arrow_forwardCreate a list of some common C++ classes, and describe when each class is used.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
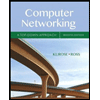
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
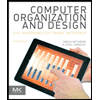
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
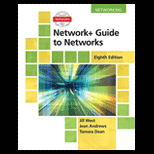
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
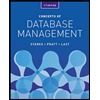
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
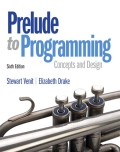
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
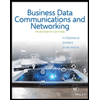
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY