Study the following C program and answer the below questions Hint: you might run it in Dev C++ before starting answering the questions! Show a sample output of the program. Extract a function declaration (prototype) for: void functions with void argument ………………………………………………………………………………………………………………… void function with a single input argument ………………………………………………………………………………………………………………… function with a single input argument and return ………………………………………………………………………………………………………………… a recursive function ………………………………………………………………………………………………………………… What is the difference between normal functions and recursive functions? How many times are the function print_stars() called in the program? (mention the line number of each call). #include void print_stars(); void option_list(); void divisors(int n); int summation(int s); int factorial (int n) ; int main(void) { int number , result , op ; char flag; printf("Enter an integer number > "); scanf( "%d" , &number ); do{ option_list(); scanf("%d" , &op); switch(op) { case 1: divisors(number); break; case 2: result = summation(number) ; printf("Summation = %d\n" , result); break; case 3: result = factorial(number) ; printf("Factorial = %d\n" , result); break; default: printf("\nERROR: %d is unrecognized option!!! \n" , op); } print_stars(); printf("Do you want to check another function? (Y/N)> "); scanf(" %c" , &flag); } while (flag == 'Y' || flag == 'y'); return 0; } void option_list(){ print_stars(); printf("1: Find the divisors \n"); printf("2: Compute the summuation\n"); printf("3: Compute the factorial \n"); print_stars(); printf("Please enter your choice: "); } void print_stars(){ int i; for(i = 1 ; i <= 25 ; i++) printf("*"); printf("\n"); } void divisors(int n) { int i; printf("Divisors of %d are\n",n); for(i=1;i<=n;i++) if(n%i==0) printf(" %d",i); printf("\n"); } int factorial (int n){ int i , fact = 1 ; for (i = 2 ; i <= n ; i++) fact *= i; return fact; } int summation(int s){ if (s == 0) return 0; else return s + summation(s-1); } What does each function do? Hint: Write a simple description for each of the user-defined functions. void print_stars(): void option_list() void divisors(int n) Example: This function takes one argument of type int and prints its divisors on the screen, including, 1 and itself. int summation(int s) int factorial (int n)
Question #2:
Study the following C
Hint: you might run it in Dev C++ before starting answering the questions!
- Show a sample output of the program.
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
- Extract a function declaration (prototype) for:
- void functions with void argument
………………………………………………………………………………………………………………… |
- void function with a single input argument
………………………………………………………………………………………………………………… |
- function with a single input argument and return
………………………………………………………………………………………………………………… |
- a recursive function
………………………………………………………………………………………………………………… |
- What is the difference between normal functions and recursive functions?
|
|
|
- How many times are the function print_stars() called in the program? (mention the line number of each call).
|
|
|
#include<stdio.h>
void print_stars();
void option_list();
void divisors(int n);
int summation(int s);
int factorial (int n) ;
int main(void) {
int number , result , op ;
char flag;
printf("Enter an integer number > ");
scanf( "%d" , &number );
do{
option_list();
scanf("%d" , &op);
switch(op) {
case 1:
divisors(number);
break;
case 2:
result = summation(number) ;
printf("Summation = %d\n" , result);
break;
case 3:
result = factorial(number) ;
printf("Factorial = %d\n" , result);
break;
default:
printf("\nERROR: %d is unrecognized option!!! \n" , op);
}
print_stars();
printf("Do you want to check another function? (Y/N)> ");
scanf(" %c" , &flag);
} while (flag == 'Y' || flag == 'y');
return 0;
}
void option_list(){
print_stars();
printf("1: Find the divisors \n");
printf("2: Compute the summuation\n");
printf("3: Compute the factorial \n");
print_stars();
printf("Please enter your choice: ");
}
void print_stars(){
int i;
for(i = 1 ; i <= 25 ; i++)
printf("*");
printf("\n");
}
void divisors(int n) {
int i;
printf("Divisors of %d are\n",n);
for(i=1;i<=n;i++)
if(n%i==0)
printf(" %d",i);
printf("\n");
}
int factorial (int n){
int i , fact = 1 ;
for (i = 2 ; i <= n ; i++)
fact *= i;
return fact;
}
int summation(int s){
if (s == 0)
return 0;
else
return s + summation(s-1);
}
- What does each function do?
Hint: Write a simple description for each of the user-defined functions.
void print_stars(): |
|
|
|
void option_list() |
|
|
|
void divisors(int n) |
Example: |
This function takes one argument of type int and prints its divisors on the screen, including, 1 and itself. |
|
int summation(int s) |
|
|
|
|
|
int factorial (int n) |
|
|
|
|
|
|
|
|

Step by step
Solved in 2 steps

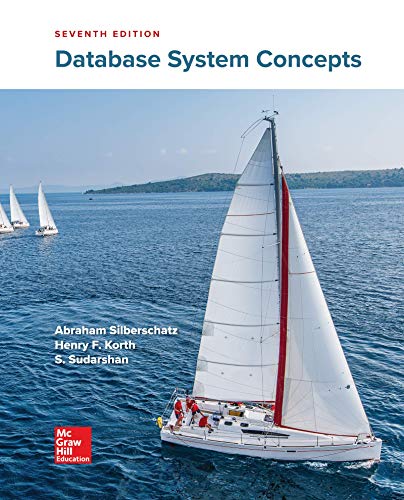
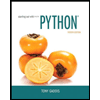
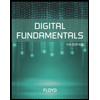
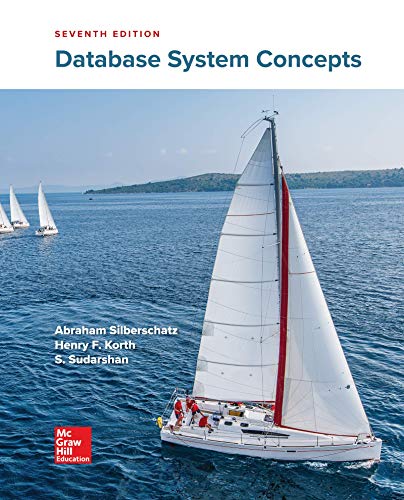
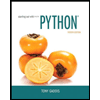
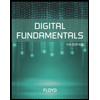
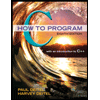
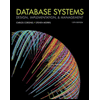
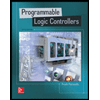