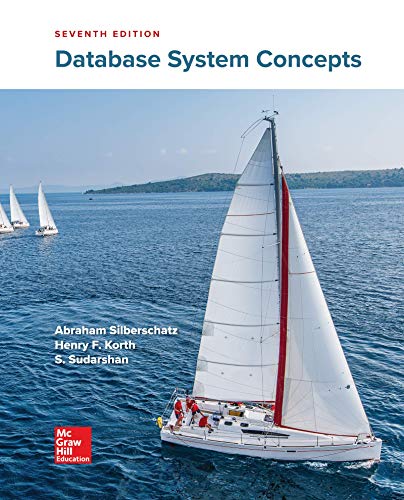
Implement in C
7.6.1: LAB: Simple car
Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar variable that performs the following operations:
- Drives input number of miles forward
- Drives input number of miles in reverse
- Honks the horn
- Reports car status
SimpleCar.h contains the struct definition and related function declarations. SimpleCar.c contains related function definitions.
Ex: If the input is:
100 4
the output is:
beep beep Car has driven: 96 miles
main.c
#include <stdio.h>
#include "SimpleCar.h"
int main() {
/* Type your code here. */
return 0;
}
SimpleCar.h
#ifndef SIMPLE_CAR_H
#define SIMPLE_CAR_H
typedef struct SimpleCar_struct {
int miles;
} SimpleCar;
SimpleCar InitCar();
SimpleCar Drive(int dist, SimpleCar car);
SimpleCar Reverse(int dist, SimpleCar car);
int GetOdometer(SimpleCar car);
void HonkHorn(SimpleCar car);
void Report(SimpleCar car);
#endif
SimpleCar.c
#include <stdio.h>
#include <string.h>
#include "SimpleCar.h"
SimpleCar InitCar(){
SimpleCar newCar;
newCar.miles = 0;
return newCar;
}
SimpleCar Drive(int dist, SimpleCar car){
car.miles = car.miles + dist;
return car;
}
SimpleCar Reverse(int dist, SimpleCar car){
car.miles = car.miles - dist;
return car;
}
int GetOdometer(SimpleCar car){
return car.miles;
}
void HonkHorn(SimpleCar car){
printf("beep beep\n");
}
void Report(SimpleCar car){
printf("Car has driven: %d miles\n", car.miles);
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- Set-up and implementation code for a void function MaxYou are not required to write a complete C++ program but must write and submit just your responses to the four specific function related questions below: QC1: Write the heading for a void function called Max that has three intparameters: num1, num2 and greatest. The first two parameters receive data from the caller, and greatest is used to return a value as a reference parameter. Document the data flow of the parameters with appropriate comments*. QC2: Write the function prototype for the function in QC1. QC3: Write the function definition of the function in QC1 so that it returns the greatest of the two input parameters via greatest, a reference parameter. QC4: Add comments to the function definition* you wrote in QC3 that also states its precondition and postcondition.arrow_forwardTrue or False : The concept of function abstraction hinders our code development by confusing us with the details of the function.arrow_forwardIN C++arrow_forward
- Write the following in C++arrow_forwardC++ Code Step 1: Preparation For the moment, "comment out" the following under-construction code: In dynamicarray.h: All function prototypes except the constructors and destructor. Keep the member variables (but we will be replacing them shortly). In dynamicarray.cpp: All function implementations except the constructors and destructor. You should also remove (not just comment out) INITIAL_CAP, and replace it with a hard-coded 10 inside the default constructor. This will also eventually go away. In main: Comment out all the code inside the RunPart1Tests function between the linesbool pass = true; and return pass; • Also in main: Comment out all the code in the main function starting with the "Equality comparison" comment and just before the "return 0;" line. Step 2: Replacing member data and the two constructors You're going to replace the current member data (arr, len, and capacity) with a single vector of integers. Remember that vectors keep track of their own size and capacity, so…arrow_forwardPython without Def function Problem Statement Define and implement a function time_delta that takes two string parameters (time1 and time2) and returns the difference between them (as a string). You may assume that the second time will always be after the first time, both times will always be on the same date, and military time will be used (no a.m. or p.m.). Examples time_delta(04:15:36, 16:35:27) ➞ (12:19:51) time_delta(02:06:05, 06:09:10) ➞ (04:03:05) Sample Input 1 "04:15:36" "16:35:27" Sample Output 1 "12:19:51"arrow_forward
- C++ Write the defnition of the function int computeProd() with parameters that receives as arguments two integer values computes their produc and returns it to the celing function.arrow_forwardHelp me with C++ please: Rewrite the definitions of the function setDate and the constructor so that the values for the month, day, and year are checked before storing the date into the member variables. Add a member function, isLeapYear, to check whether a year is a leap year. Moreover, write a test program to test your class. Input should be format month day year with each separated by a space. Output should resemble the following: Date #: month-day-year An example of the program is shown below: Date 1: 3-15-2008 this is a leap year There are 3 tabs: dateType.h, dateTypelmp.cpp, main.cpp dateType.h: #ifndef date_H #define date_H class dateType { public: void setDate(int month, int day, int year); //Function to set the date. //The member variables dMonth, dDay, and dYear are set //according to the parameters //Postcondition: dMonth = month; dDay = day; // dYear = year int getDay() const; //Function to return the day.…arrow_forwardDefine a struct fruitType to store the following data about a fruit: Fruit name (string), color (string), fat (int), sugar (int), and carbohydrate (int). Declare a variable of type fruitType to store the following data: Fruit name—banana, color—yellow, fat—1, sugar—15, carbohydrate—22. Write a C++ function, getFruitInput to get and store data into a variable of fruitType. Write a C++ function, printFruitInfo to output data stored into a variable of fruitType. Use appropriate labels to identify each component. Test your solution by calling all funtions.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
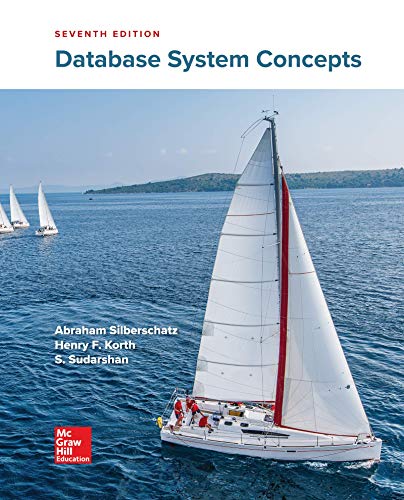
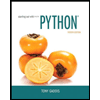
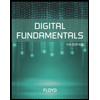
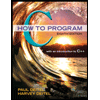
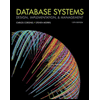
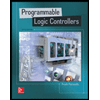