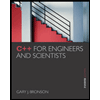
C++ for Engineers and Scientists
4th Edition
ISBN: 9781133187844
Author: Bronson, Gary J.
Publisher: Course Technology Ptr
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
4. Consolidation may be done by writing a C++ programme. Utilise the associated hub data and sort on the related rundown.
![On this page, we explore the definition of a structure in C++ to represent an employee with specific attributes. Below is an example of how to declare such a structure:
```cpp
struct employee {
char firstName[20];
char lastName[20];
unsigned int age;
char gender;
double hourlySalary;
};
```
#### Explanation:
1. **Struct Declaration (`struct employee`)**:
- Keyword `struct` is used to define a structure named `employee`.
2. **Members of the Structure**:
- `char firstName[20];`: An array of characters to store the first name of the employee with a maximum length of 20 characters.
- `char lastName[20];`: An array of characters to store the last name of the employee with a maximum length of 20 characters.
- `unsigned int age;`: An unsigned integer representing the age of the employee.
- `char gender;`: A single character to store the gender of the employee.
- `double hourlySalary;`: A double type to store the hourly salary of the employee.
#### Instructions:
- **Defining Classes and Functions**:
- According to your program requirements, define corresponding classes and functions that manage or utilize the `employee` structure.
- **Report Writing**:
- Write a detailed report explaining your work. Ensure that the explanation is provided line by line, detailing each step and its purpose.
This information and example serve as a foundational aid for students to understand how to define and use structures in C++. The explanation and requirements can be used for programming tasks, assignments, or projects related to employee data management.](https://content.bartleby.com/qna-images/question/975eedeb-cca9-452a-a623-85539861d2ea/f7d09ac4-6f1d-4a2c-a47c-fddc68d84fca/5sbsgjo_thumbnail.png)
Transcribed Image Text:On this page, we explore the definition of a structure in C++ to represent an employee with specific attributes. Below is an example of how to declare such a structure:
```cpp
struct employee {
char firstName[20];
char lastName[20];
unsigned int age;
char gender;
double hourlySalary;
};
```
#### Explanation:
1. **Struct Declaration (`struct employee`)**:
- Keyword `struct` is used to define a structure named `employee`.
2. **Members of the Structure**:
- `char firstName[20];`: An array of characters to store the first name of the employee with a maximum length of 20 characters.
- `char lastName[20];`: An array of characters to store the last name of the employee with a maximum length of 20 characters.
- `unsigned int age;`: An unsigned integer representing the age of the employee.
- `char gender;`: A single character to store the gender of the employee.
- `double hourlySalary;`: A double type to store the hourly salary of the employee.
#### Instructions:
- **Defining Classes and Functions**:
- According to your program requirements, define corresponding classes and functions that manage or utilize the `employee` structure.
- **Report Writing**:
- Write a detailed report explaining your work. Ensure that the explanation is provided line by line, detailing each step and its purpose.
This information and example serve as a foundational aid for students to understand how to define and use structures in C++. The explanation and requirements can be used for programming tasks, assignments, or projects related to employee data management.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Mark the following statements as true or false. All members of a struct must be of different types. (1) A struct is a definition, not a declaration. (1) A struct variable must be declared after the struct definition. (1) A struct member is accessed by using the operator :. (2) The only allowable operations on a struct are assignment and member selection. (2) Because a struct has a finite number of components, relational operations are allowed on a struct. (2) Some aggregate input/output operations are allowed on a struct variable. (2) A struct variable can be passed as a parameter either by value or by reference. (4) A function cannot return a value of the type struct. (4) An array can be a member of a struct. (6, 7) A member of a struct can be another struct. (8)arrow_forward1. Mark the following statements as true or false. a. An identifier must start with a letter and can be any sequence of characters. (1) b. In C++, there is no difference between a reserved word and a predefined identifier. (1) c. A C++ identifier cannot start with a digit. (1) d. The collating sequence of a character is its preset number in the character data set. (2) e. Only one of the operands of the modulus operator needs to be of type int. (3) f. If ; and ;, then after the statement ; the value of b is erased. (6) g. If the input is 7 and x is a variable of type int, then the statement ; assigns the value 7 to x. (6) h. In an output statement, the newline character may be a part of the string. (10) i. In C++, all variables must be initialized when they are declared. (7) j. In a mixed expression, all the operands are converted to floating-point numbers. (4) k. Suppose . After the statement ; executes, y is 5 and x is 6. (9) i. Suppose . After the statement ; executes, the value of a is still 5 because the value of the expression is not saved in another variable. (9)arrow_forwardIndicate whether each of the following C# programming language identifiers is legal or illegal. If it is legal, indicate whether it is a conventional identifier for a class. electricBill ElectricBill Electric bill Static void #ssn Ay56we Theater_Tickets 212AreaCode heightInCentimeters Zip23891 Voidarrow_forward
- (Data processing) A bank’s customer records are to be stored in a file and read into a set of arrays so that a customer’s record can be accessed randomly by account number. Create the file by entering five customer records, with each record consisting of an integer account number (starting with account number 1000), a first name (maximum of 10 characters), a last name (maximum of 15 characters), and a double-precision number for the account balance. After the file is created, write a C++ program that requests a user-input account number and displays the corresponding name and account balance from the file.arrow_forward(Data processing) a. Declare a single structure data type suitable for a car structure of the type in the following chart: b. Using the data type declared for Exercise 5a, write a C++ program that interactively accepts the chart’s data in an array of five structures. After the data has been entered, the program should create a report listing each car number and the car’s miles per gallon. At the end of the report, include the average miles per gallon for the entire fleet of cars.arrow_forward(Program) Include the Date class definition in Class 11.1 in a complete program. Your program should verify that each member function works correctly.arrow_forward
- (Conversion) Write a C++ program that converts gallons to liters. The program should display gallons from 10 to 20 in 1-gallon increments and the corresponding liter equivalents. Use the relationship that 1gallon=3.785liters.arrow_forwardWhat is the difference between a friend function of a class and a member function of a class? (3)arrow_forwardWhy do you need to include function prototypes in a program that contains user-defined functions? (5)arrow_forward
- A(n) ____________ is one instance or variable of a class.arrow_forwardUsing classes, design an online address book to keep track of the names, addresses, phone numbers, and dates of birth of family members, close friends, and certain business associates. Your program should be able to handle a maximum of 500 entries. Define a class addressType that can store a street address, city, state, and ZIP code. Use the appropriate functions to print and store the address. Also, use constructors to automatically initialize the member variables. Define a class extPersonType using the class personType (as defined in Example 10-10, Chapter 10), the class dateType (as designed in this chapters Programming Exercise 2), and the class addressType. Add a member variable to this class to classify the person as a family member, friend, or business associate. Also, add a member variable to store the phone number. Add (or override) the functions to print and store the appropriate information. Use constructors to automatically initialize the member variables. Define the class addressBookType using the previously defined classes. An object of the type addressBookType should be able to process a maximum of 500 entries. The program should perform the following operations: Load the data into the address book from a disk. Sort the address book by last name. Search for a person by last name. Print the address, phone number, and date of birth (if it exists) of a given person. Print the names of the people whose birthdays are in a given month. Print the names of all the people between two last names. Depending on the users request, print the names of all family members, friends, or business associates.arrow_forward(Program) a. Complete the following class by including functions corresponding to the two prototypes listed in the declaration section: classElevatorprivate:intelNum;//elevatornumberintcurrentFloor;//currentfloorinthighestFloor;//highestfloorpublic:Elevator( int=1,int=1,int=15);//constructorvoidrequest( int);; In this definition, the data member elNum is used to store the elevator’s number, the data member currentFloor is used to store the elevator’s current floor position, and the data member highestFloor is used to store the highest floor the elevator can reach.The constructor should allow initialization of an object’s three data members with the data passed to the constructor when an Elevator object is instantiated. The request function should code the following algorithm: If a request is made for a nonexistent floor, a floor higher than the topmost floor, or the current floor Do nothing ElseIf the request is for a floor above the current floor Display the current floor number While not at the designated floor Increment the floor number Display the new floor number EndWhile Display the ending floor number Else // the request must be for a floor below the current floor Display the current floor number While not at the designated floor Decrement the floor number Display the new floor number EndWhile Display the ending floor number EndIf b. Include the class written for Exercise 3a in a complete program, and verify that all member functions work correctly.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
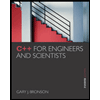
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
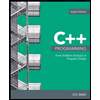
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
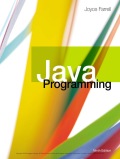
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
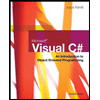
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
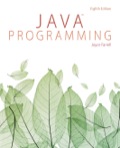
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
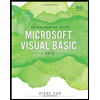
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning