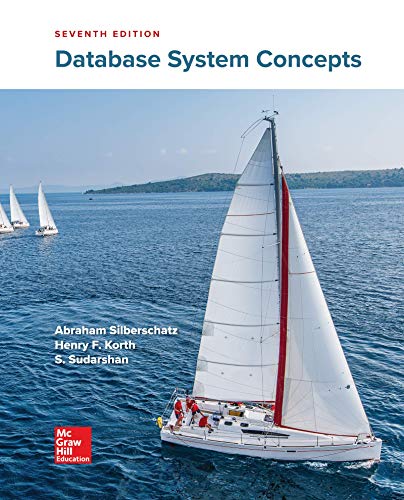
Write a C/C++ program that calculates the least squares line for two sets of values. The program consists of several functions which are described below.
int ReadFile (int *, int *);
Write a function that reads the contents of a text file (Data1.txt) into two separate integer arrays. The function receives two integer pointers to arrays as parameters.
The contents of the text file that is attached is structured as follows: Each line in the file contains two values that are separated by a comma. The values on the left of the comma, are stored in one array and the values on the right side is stored in the other array.
The number of lines that are read from the file and stored in the arrays are returned to the calling statement.
Tip: a single fscanf can read into multiple variables. Ex. the statement fscanf(fp, "%s %s\n", name,surname) will read the line John Doe and save the name and surname separately. (Note the space between %s and %s indicates that there is a space in the data between the two strings to be captured)
void LeastSquaresLine (int *, int *, double *, double *, int);
Write a function that determines the elements a0 and a1 of the least squares line for the contents of two arrays. The function receives two integer pointers for arrays (x and y), two reference parameters for the return of a0 and a1, and the length of the arrays.
The least squares line is determined as follows:
See Image 1
The function does not return any value to the calling statement.
void WriteFile (double, double);
Write a function that saves the formula of the least squares line to a text file. The function receives two real values for a0 and a1 after which the formula is saved in the form y = mx + c where m = a0 and c = a1. See the example output for the file contents.
See Output_File_Contene_image
If the file can not be opened for writing an appropriate error message is to be displayed. The function does not return any value to the calling statement.
int main ();
Implement the above functions in the main function to complete the program.
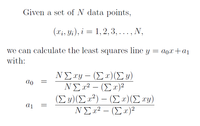
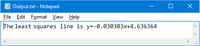

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Debug the following phython code. """This function reads in data from the file specified by filename. This data is read line by line and unpacked into a single variable called data. argumentsfilename - the name of the file to be read returnsdata - a list of data, each element is a list that corresponds to the set of entries separated by commas in the original file. To access the first entry use data[0]. If x = data[0], then x[0], through x[3] will correspond to entries in the file that are read in. """ def read_file(filename):fin = open(filename,'r')line = fin.readline()data = []while line:data.append(parse_line(line))line = fin.readline()return dataarrow_forwardin C++ pleasearrow_forwardC++ Problem!! Sort A List Of Integers Given a file of 100,000 unsorted integers, write a program that first prompt user to enter file name and reads those integers into an array and sorts the integers in the array. The program should then prompt the user for an index, and then display the integer at that index. For example, if the array contains ten integers and the contents are 79 4 42 51 12 22 33 17 91 10 after sorting, the integer at index 6 is 42 Download the "List of 100,000 Unsorted Integers" from below link and sort that list, then enter the value at index 10000 (ten thousand) for a screenshot. https://1drv.ms/t/s!AuFS4mkcuYyBjHZ_SIudb93bq2uy?e=CsyvZjarrow_forward
- How do I do this C programming question?arrow_forwardThis is for C++ Design an application that uses three identical arrays of at least 20 integers. It should call each module on a different array, and display the number of swaps made by each algorithm. 8 Sorting Benchmarks (bubble, selection & insertion sort) The following represents the output from 3 separate files: BubbleSort, SelectSort and InsertionSort. Don't ask the user to enter any numbers, but 'hard code' the values in the "Original order" as shown. Original order:26 45 56 12 78 74 39 22 5 90 87 32 28 11 93 62 79 53 22 51Bubble Sorted:5 11 12 22 22 26 28 32 39 45 51 53 56 62 74 78 79 87 90 93Number of location swaps: 89 Original order:26 45 56 12 78 74 39 22 5 90 87 32 28 11 93 62 79 53 22 51Selection Sorted:5 11 12 22 22 26 28 32 39 45 51 53 56 62 74 78 79 87 90 93Number of location swaps: 19 Original order:26 45 56 12 78 74 39 22 5 90 87 32 28 11 93 62 79 53 22 51Insertion Sorted:5 11 12 22 22 26 28 32 39 45 51 53 56 62 74 78 79 87 90 93Number of location…arrow_forwardC++ Create a new file named array_ptrs.cpp and in this file, write a program that does the following: Defines an array of integers called my_ints with a pre-specified size of 4. Populates my_ints with input data provided by the user Defines an array of pointers called my_ptrs of the same size as my_ints and initializes the pointers in my_ptrs to point to corresponding elements of the array my_ints (refer to the “initial state” in the example shown below) Sort the array of pointers my_ptrs in a way such that the pointer at index 0 (i.e., the first element of my_ptrs) points to the smallest element in my_ints, the pointer at index 1 points to the next larger element in my_ints, etc. (refer to the “State after sorting my_ptrs” in the example shown below). Traverse the array my_ptrs and print the values being pointed to by each pointer in this array. Take a screenshot of a sample output and upload the picture as part of your assignment submission.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
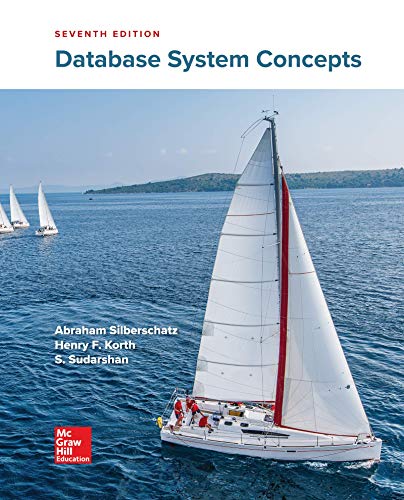
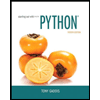
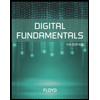
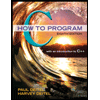
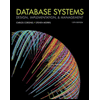
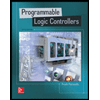