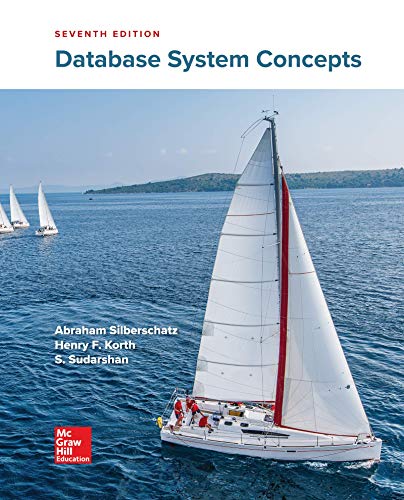
Concept explainers
Sets are collections (1) without defined order and (2) not allowing duplication. Multisets, also called “bags” are collections without defined order but which permit duplication, i.e., more than one element. We define the function #(a B) to be the number of occurrences of the element a in the bag B. For example, #(1, [1 1 2 3 4 4 5]) is 2 and #(5, [1 1 2 3 4 4 5]) = 1. Bag union and intersection are defined in terms of #.
bag-union: List × List -> List
This function should take as arguments two lists representing bags and should return the list representing their bag-union.
bag-intersection : List × List -> List
This function should take as arguments two lists representing bags and should return the list representing their bag-intersection.
Allowed functions. Your code must use only the following functions:
1. define, let
2. lambda, curry
3. cons, car, cdr, list, list?, append, empty?, length, equal?
4. and, or, not
5. if, cond
6. map, append-map, andmap, ormap, filter, apply
7. +, -, /, *
Racket code only please. Thank you!

Here's the implementation of bag-union and bag-intersection in Racket:
(define (bag-union bag1 bag2)
(let ((merged (append bag1 bag2)))
(filter (lambda (x) (not (zero? (apply + (map (lambda (y) (if (equal? x y) 1 0)) merged))))) merged)))
(define (bag-intersection bag1 bag2)
(let ((merged (append bag1 bag2)))
(filter (lambda (x) (not (zero? (min (apply + (map (lambda (y) (if (equal? x y) 1 0)) bag1)) (apply + (map (lambda (y) (if (equal? x y) 1 0)) bag2))))))) merged)))
this is the implementation of bag-union and bag-intersection in Racket as described in my previous answer. The bag-union function first appends the two bags bag1 and bag2 to form a merged list, and then uses the filter function to remove duplicates. The filter function takes as input a function (lambda expression) that checks if the number of occurrences of an element in the merged list is greater than 0. The bag-intersection function works in a similar manner, but instead of filtering elements that have a non-zero count in the merged list, it filters elements that have non-zero counts in both bag1 and bag2.
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Write a function named cumulative that accepts as a parameter a reference to a vectorof integers, and modifies it so that each element contains the cumulative sum of the elements up through that index. For example, if the vector passed contains {1, 1, 2, 3, 5}, your function should modify it to store {1, 2, 4, 7, 12}.arrow_forwardDouble linked list in Java Double linked list Textbook reference Data structures and algorithms Micheal Goodricharrow_forwardFinding the Minimum of a Vector Write a function to return the index of the minimum value in a vector, or -1 if the vector doesn't have any elements. In this problem you will implement the IndexOfMinimumElement function in minimum.cc and then call that function from main.cc. You do not need to edit minimum.h. Index of minimum value with std::vector Complete IndexOfMinimumElement in minimum.cc. This function takes a std::vector containing doubles as input and should return the index of the smallest value in the vector, or -1 if there are no elements. Complete main.cc Your main function asks the user how many elements they want, construct a vector, then prompts the user for each element and fills in the vector with values. Your only task in main is to call the IndexOfMinimumElement function declared in minimum.h, and print out the minimum element's index. Here's an example of how this might look: How many elements? 3 Element 0: 7 Element 1: -4 Element 2: 2 The minimum value in your…arrow_forward
- Write a program that will sort a prmiitive array of data using the following guidelines - DO NOT USE VECTORS, COLLECTIONS, SETS or any other data structures from your programming language. The codes for both the money and currency should stay the same, the main focus should be on the main file code (Programming language Java) Create a helper function called 'RecurInsSort' such that: It is a standalone function not part of any class from the prior lab or any new class you feel like creating here, Takes in the same type of parameters as any standard Insertion Sort with recursion behavior, i.e. void RecurInsSort(Currency arr[], int size) Prints out how the array looks every time a recursive step returns back to its caller The objects in the array should be Money objects added or manipulated using Currency references/pointers. It is OK to print out the array partially when returning from a particular step as long as the process of sorting is clearly demonstrated in the output. In…arrow_forwardComplete the function: bool search(vector<int> v, int key). This function searches for value key in vector v and return true if key is found else return false. In C++arrow_forwardC++.Write a function, named SecondNegative, that takes a const reference to a vector of doubles, it should return an const_iterator to the element in the vector that is the second value that is negative. If no such element exists, have the iterator point to one past the end.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
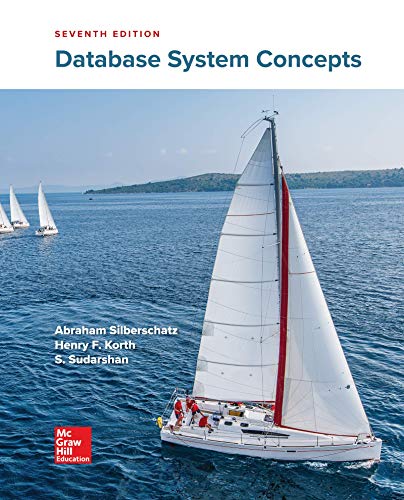
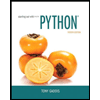
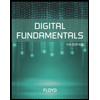
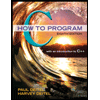
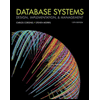
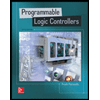