//Server.c #include "csapp.h" void *thread(void *vargp); int main(int argc, char *argv[]) { int listenfd; socklen_t clientlen; char client_hostname[MAXLINE], client_port[MAXLINE]; struct sockaddr_storage clientaddr; pthread_t tid; if (argc != 2) { fprintf(stderr, "usage: %s \n", argv[0]); exit(0); } listenfd = Open_listenfd(argv[1]); while (1) { clientlen = sizeof(struct sockaddr_storage); int *connfdp = Malloc(sizeof(int)); *connfdp = Accept(listenfd, (SA *)&clientaddr, &clientlen); Getnameinfo((SA *)&clientaddr, clientlen, client_hostname, MAXLINE, client_port, MAXLINE, 0); printf("Connected to (%s, %s)\n", client_hostname, client_port); Pthread_create(&tid, NULL, thread, connfdp); } } void *thread(void *vargp) { int connfd = *((int *)vargp); Pthread_detach(pthread_self()); Free(vargp); size_t n; rio_t rio; int option; char firstName[256]; char lastName[256]; char filefirstName[256]; char filelastName[256]; int age; char major[256]; char buffer[256]; Rio_readinitb(&rio, connfd); while (1) { bzero(buffer, 256); if ((n = Rio_readlineb(&rio, buffer, 255)) == 0) { printf("Connection closed\n"); break; } option = atoi(buffer); if (option == 1) { bzero(firstName, 256); n = rio_readlineb(&rio, firstName, 255); if (n < 0) { perror("ERROR reading from socket\n"); exit(1); } bzero(lastName, 256); n = rio_readlineb(&rio, lastName, 255); if (n < 0) { perror("ERROR reading from socket\n"); exit(1); } firstName[strlen(firstName) - 1] = '\0'; lastName[strlen(lastName) - 1] = '\0'; FILE *fptr; int found = 0; fptr = fopen("studentRecords.txt", "r"); if (fptr == NULL) { printf("Error opening file!\n"); exit(1); } bzero(buffer, 256); while (fscanf(fptr, "%[^,],%[^,],%d,%[^\n]\n", filefirstName, filelastName, &age, major) != EOF) { if (strcmp(firstName, filefirstName) == 0 && strcmp(lastName, filelastName) == 0) { found = 1; sprintf(buffer, "%s,%s,%d,%s\n", firstName, lastName, age, major); n = rio_writen(connfd, buffer, strlen(buffer)); if (n < 0) { perror("ERROR writing to socket\n"); exit(1); } } } fclose (fptr); if (!found) { bzero(buffer, 256); sprintf(buffer, "No record found!!\n"); n = rio_writen(connfd, buffer, strlen(buffer)); if (n < 0) { perror("ERROR writing to socket\n"); exit(1); } } bzero(buffer, 256); sprintf(buffer, "\n"); n = rio_writen(connfd, buffer, strlen(buffer)); if (n < 0) { perror("ERROR writing to socket\n"); exit(1); } } else if (option == 2) { // Close the connection Close(connfd); break; } } return NULL; } //client.c #include "csapp.h" int main(int argc, char *argv[]) { int sockfd; ssize_t n; rio_t rio; char buffer[256]; int option; char firstName[256]; char lastName[256]; char major[256]; if (argc < 3) { fprintf(stderr, "usage %s hostname port\n", argv[0]); exit(0); } sockfd = Open_clientfd(argv[1], argv[2]); rio_readinitb(&rio, sockfd); while (1) { printf("\n(1) Search record\n(2) Terminate\nSelect an option [1 or 2]: "); bzero(buffer, 256); if (fgets(buffer, 255, stdin) == NULL) { perror("ERROR reading value"); exit(1); } option = atoi(buffer); n = rio_writen(sockfd, buffer, strlen(buffer)); if (n < 0) { perror("ERROR writing to socket"); exit(1); } if (option == 1) { printf("Enter first name: "); bzero(firstName, 256); if (fgets(firstName, 255, stdin) == NULL) { perror("ERROR reading value"); exit(1); } printf("Enter last name: "); bzero(lastName, 256); if (fgets(lastName, 255, stdin) == NULL) { perror("ERROR reading value"); exit(1); } n = rio_writen(sockfd, firstName, strlen(firstName)); if (n < 0) { perror("ERROR writing to socket"); exit(1); } n = rio_writen(sockfd, lastName, strlen(lastName)); if (n < 0) { perror("ERROR writing to socket"); exit(1); } bzero(buffer, 256); printf("Message from server:\n"); while ((n = rio_readlineb(&rio, buffer, 255)) != 0) { if (strlen(buffer) == 1) { break; } buffer[strlen(buffer) - 1] = '\0'; printf("%s \n", buffer); if (strcmp(buffer, "No record found!!") == 0) { break; } } if (n < 0) { perror("ERROR reading from socket\n"); exit(1); } } else if (option == 2) { close(sockfd); printf("Connection Closed!!\n"); break; } } exit(0); } Can you help me demonstrate of these two files? as well as a problem solving approach, discussion of data structures, algorithms, user define functions, discussion of challanges that I faced, references you used (if any).
//Server.c
#include "csapp.h"
void *thread(void *vargp);
int main(int argc, char *argv[])
{
int listenfd;
socklen_t clientlen;
char client_hostname[MAXLINE], client_port[MAXLINE];
struct sockaddr_storage clientaddr;
pthread_t tid;
if (argc != 2)
{
fprintf(stderr, "usage: %s <port>\n", argv[0]);
exit(0);
}
listenfd = Open_listenfd(argv[1]);
while (1)
{
clientlen = sizeof(struct sockaddr_storage);
int *connfdp = Malloc(sizeof(int));
*connfdp = Accept(listenfd, (SA *)&clientaddr, &clientlen);
Getnameinfo((SA *)&clientaddr, clientlen,
client_hostname, MAXLINE,
client_port, MAXLINE, 0);
printf("Connected to (%s, %s)\n", client_hostname, client_port);
Pthread_create(&tid, NULL, thread, connfdp);
}
}
void *thread(void *vargp)
{
int connfd = *((int *)vargp);
Pthread_detach(pthread_self());
Free(vargp);
size_t n;
rio_t rio;
int option;
char firstName[256];
char lastName[256];
char filefirstName[256];
char filelastName[256];
int age;
char major[256];
char buffer[256];
Rio_readinitb(&rio, connfd);
while (1)
{
bzero(buffer, 256);
if ((n = Rio_readlineb(&rio, buffer, 255)) == 0)
{
printf("Connection closed\n");
break;
}
option = atoi(buffer);
if (option == 1)
{
bzero(firstName, 256);
n = rio_readlineb(&rio, firstName, 255);
if (n < 0)
{
perror("ERROR reading from socket\n");
exit(1);
}
bzero(lastName, 256);
n = rio_readlineb(&rio, lastName, 255);
if (n < 0)
{
perror("ERROR reading from socket\n");
exit(1);
}
firstName[strlen(firstName) - 1] = '\0';
lastName[strlen(lastName) - 1] = '\0';
FILE *fptr;
int found = 0;
fptr = fopen("studentRecords.txt", "r");
if (fptr == NULL)
{
printf("Error opening file!\n");
exit(1);
}
bzero(buffer, 256);
while (fscanf(fptr, "%[^,],%[^,],%d,%[^\n]\n", filefirstName, filelastName, &age, major) != EOF)
{
if (strcmp(firstName, filefirstName) == 0 && strcmp(lastName, filelastName) == 0)
{
found = 1;
sprintf(buffer, "%s,%s,%d,%s\n", firstName, lastName, age, major);
n = rio_writen(connfd, buffer, strlen(buffer));
if (n < 0)
{
perror("ERROR writing to socket\n");
exit(1);
}
}
}
fclose
(fptr);
if (!found)
{
bzero(buffer, 256);
sprintf(buffer, "No record found!!\n");
n = rio_writen(connfd, buffer, strlen(buffer));
if (n < 0)
{
perror("ERROR writing to socket\n");
exit(1);
}
}
bzero(buffer, 256);
sprintf(buffer, "\n");
n = rio_writen(connfd, buffer, strlen(buffer));
if (n < 0)
{
perror("ERROR writing to socket\n");
exit(1);
}
}
else if (option == 2)
{
// Close the connection
Close(connfd);
break;
}
}
return NULL;
}
//client.c
#include "csapp.h"
int main(int argc, char *argv[])
{
int sockfd;
ssize_t n;
rio_t rio;
char buffer[256];
int option;
char firstName[256];
char lastName[256];
char major[256];
if (argc < 3)
{
fprintf(stderr, "usage %s hostname port\n", argv[0]);
exit(0);
}
sockfd = Open_clientfd(argv[1], argv[2]);
rio_readinitb(&rio, sockfd);
while (1)
{
printf("\n(1) Search record\n(2) Terminate\nSelect an option [1 or 2]: ");
bzero(buffer, 256);
if (fgets(buffer, 255, stdin) == NULL)
{
perror("ERROR reading value");
exit(1);
}
option = atoi(buffer);
n = rio_writen(sockfd, buffer, strlen(buffer));
if (n < 0)
{
perror("ERROR writing to socket");
exit(1);
}
if (option == 1)
{
printf("Enter first name: ");
bzero(firstName, 256);
if (fgets(firstName, 255, stdin) == NULL)
{
perror("ERROR reading value");
exit(1);
}
printf("Enter last name: ");
bzero(lastName, 256);
if (fgets(lastName, 255, stdin) == NULL)
{
perror("ERROR reading value");
exit(1);
}
n = rio_writen(sockfd, firstName, strlen(firstName));
if (n < 0)
{
perror("ERROR writing to socket");
exit(1);
}
n = rio_writen(sockfd, lastName, strlen(lastName));
if (n < 0)
{
perror("ERROR writing to socket");
exit(1);
}
bzero(buffer, 256);
printf("Message from server:\n");
while ((n = rio_readlineb(&rio, buffer, 255)) != 0)
{
if (strlen(buffer) == 1)
{
break;
}
buffer[strlen(buffer) - 1] = '\0';
printf("%s \n", buffer);
if (strcmp(buffer, "No record found!!") == 0)
{
break;
}
}
if (n < 0)
{
perror("ERROR reading from socket\n");
exit(1);
}
}
else if (option == 2)
{
close(sockfd);
printf("Connection Closed!!\n");
break;
}
}
exit(0);
}
Can you help me demonstrate of these two files?
as well as a problem solving approach, discussion of data structures,

Step by step
Solved in 3 steps

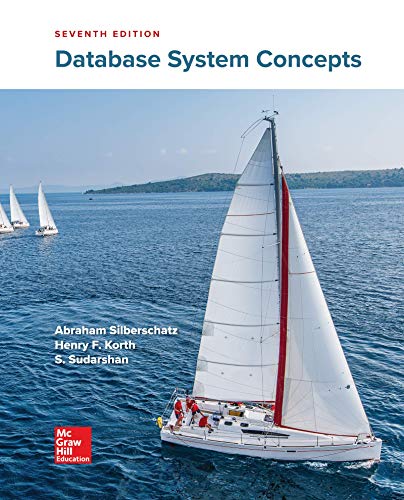
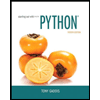
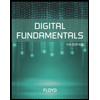
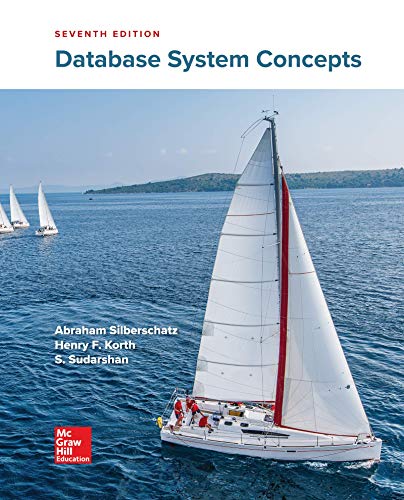
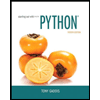
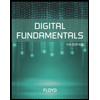
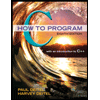
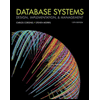
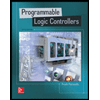