#SumCalculator Class: Create a class named SumCalculator that extends the Thread class. This class calculates the sum of numbers within a provided range. Declare three instance variables: start, end, and sum in the SumCalculator class . start and end are the lower and upper bounds of the range, respectively, while sum stores the sum of the numbers within the range. Create a constructor that takes in two parameters: start and end, and initializes the corresponding instance variables. The sum variable should be initialized to 0. Define the run method to calculate the sum of the numbers within the range. Inside of the run method use a for-loop to iterate over the numbers within the range and add each number to the sum variable. Define the accessor method getSum that returns the value of the sum variable. #Main Class: Create a class named Main that contains the main method, the entry point of the program. Create two objects of the SumCalculator class , with the first object calculating the sum of the numbers from 1 to 50, and the second object calculating the sum of the numbers from 51 to 100. Call the start method on both instances of the SumCalculator class to start the calculation of the sum in separate threads. Call the join method on both instances to wait for the calculation of the sum to complete in both threads. Call the getSum method on both instances to get the sum of the numbers calculated in each thread. This should add the results to get the total sum. Print the total sum to the console. ======================================================================================= class SumCalculator extends Thread { // The start point of the range private int start; // The end point of the range private int end; // The sum of the numbers in the range private int sum; // Constructor to set the start and end points of the range public SumCalculator(int start, int end) { //Initialize the instance variables start and end // Initialize the sum to 0 } // Override the run method to calculate the sum of the numbers in the range public void run() { // Iterate through the numbers in the range and add them to the sum } // Method to get the sum of the numbers in the range public int getSum() { return sum; } } public class Main { public static void main(String[] args) { // Create two SumCalculator threads to calculate the sum of two different ranges SumCalculator sumCalculator1 = new SumCalculator(1, 50); SumCalculator sumCalculator2 = new SumCalculator(51, 100); // Start both threads // Wait for both threads to finish using join() method try { } catch (InterruptedException e) { // Print the stack trace if an interruption occurs e.printStackTrace(); } // Calculate the total sum by adding the sum of both ranges int totalSum = sumCalculator1.getSum() + sumCalculator2.getSum(); // Print the total sum System.out.println("Total sum: " + totalSum); } }
#SumCalculator Class:
-
Create a class named SumCalculator that extends the Thread class. This class calculates the sum of numbers within a provided range.
-
Declare three instance variables: start, end, and sum in the SumCalculator class . start and end are the lower and upper bounds of the range, respectively, while sum stores the sum of the numbers within the range.
-
Create a constructor that takes in two parameters: start and end, and initializes the corresponding instance variables. The sum variable should be initialized to 0.
-
Define the run method to calculate the sum of the numbers within the range. Inside of the run method use a for-loop to iterate over the numbers within the range and add each number to the sum variable.
-
Define the accessor method getSum that returns the value of the sum variable.
#Main Class:
-
Create a class named Main that contains the main method, the entry point of the program.
-
Create two objects of the SumCalculator class , with the first object calculating the sum of the numbers from 1 to 50, and the second object calculating the sum of the numbers from 51 to 100.
-
Call the start method on both instances of the SumCalculator class to start the calculation of the sum in separate threads.
-
Call the join method on both instances to wait for the calculation of the sum to complete in both threads.
-
Call the getSum method on both instances to get the sum of the numbers calculated in each thread. This should add the results to get the total sum.
-
Print the total sum to the console.
=======================================================================================

Step by step
Solved in 4 steps with 3 images

Try commenting out your thread code and summing all of the integers from 1 to 2000. How long does it take?
Now, uncomment that thread code and split your sums into the two portions,
How long does it take now?
What are some other ways you could improve this code? When the numbers got bigger, what did you notice? What surprised you?
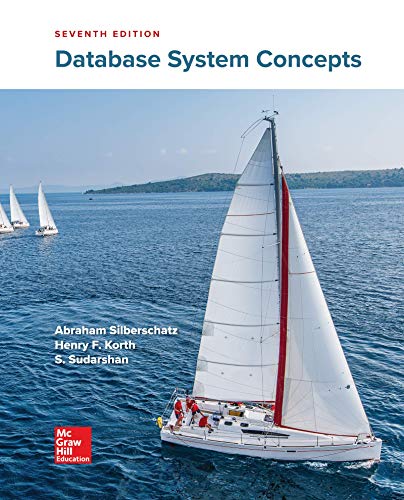
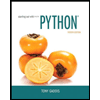
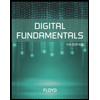
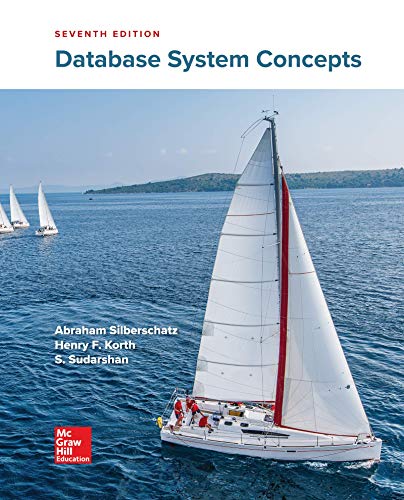
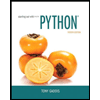
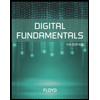
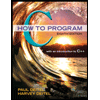
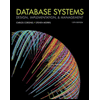
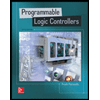