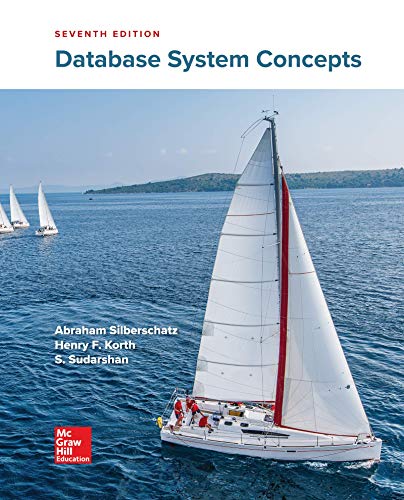
See https://pytorch.org/docs/stable/generated/torch.nn.CrossEntropyLoss.html for details. It expects an input of size Nsamples x Nclasses, which is un-normalized logits, and a target, which is y_train_l of size Nsamplesx1. You may also feed it y_train_T of size Nsample x Nclasses. Please see the documentation.
Cross entropy loss expects raw unnrormalized scores. Soft-max converts raw unnormalized scores to probabilities, which are used to plot the labels.
Use SGD and run 20,000 epochs using a learning rate of 1e-2 to train the neural network
code:
nn = NeuralNet(Nfeatures,Nclasses,20,20).to(device)
sm = N.Softmax(dim=1)
# Weight the cross entropy loss to balance the classes
Nsamples_per_class = y_train_T.sum(axis=0)
Weight = Nsamples_per_class.sum()/Nsamples_per_class
loss = torch.nn.CrossEntropyLoss(weight=Weight)
learning_rate = 0.01
#YOUR CODE HERE
optimizer= optim.SGD(net.parameters(), lr=learning_rate) # define optimizer
for epoch in range(20000):
#YOUR CODE HERE
predNN = ?? # Forward pass
error = ?? # find the loss
optimizer.zero_grad() # clear the gradients
backward.loss() # Send loss backward
optimizer.step() # update weights
if(np.mod(epoch,5000)==0):
print("Error =",error.detach().cpu().item())
fig,ax = plt.subplots(1,2,figsize=(12,4))
ax[0].plot(y_train_T[0:40].detach().cpu())
ax[1].plot(sm(predNN[0:40]).detach().cpu())
plt.show()
Need help with predNN and error formula

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Draw the function ? = 1⁄(1 + ?2) on 2 dimensions X and Z by dividing the -2π to 2π range of x from steps of 0.1 with color blue and dash-dotted line. Imagine that the drawn line of z is a ‘thread’ which if we can copy it multiple times and those copies are kept in parallel to the first one over the direction of a 3rd axis we will generate a surface representing the same curve. a. Use meshgrid function on the x vector and save the result on xx. b. Using size function find the size of xx. c. How does dimensions of xx relate to dimensions of x? d. Using the knowledge of vector multiplication in Octave and using in-built generating functions and size functions, for a given vector v write a single argument function to generate its ‘meshgrid version’ and return it in the variable rep. Avoid using loops! Name your function replicate. (Note: Check if the result is identical to the meshgrid function’s output. Cross check with a small vector) ChatGPTarrow_forwardGiven a data set x, the following R commands have been run: library(cluster); agnes (x=X)->AG; KPM Match the following objects with what you expect the R output to be. PM$id.med Choose... PM$clustering Choose... AG$order Choose... AG$ac Choose... AG$height Choose... [1] 1, 1, 2, 1, 1, 1, 2 [1] 1, 5, 6, 7, 2, 3, 4 [1] 6, 7 [1] 0.1517008 [1] 4, 4, 4, 4.7948, 4.4721, 4arrow_forwardExpanding randomness. Alice wants to implement AES in CTRmode on a small IoT device that doesn’t have a good source of randomness.She has observed that, in practice, she can obtain four randombits for each encryption. To produce the 64 bits of nonce needed foreach encryption, she proceeds as follows. She takes the SHA256 of thefour random bits. Then she uses the first 64 bits of the SHA256 outputas the nonce. Is this method secure? Explain your reasoning. (Hint:consider what happens if the adversary tries to guess the nonce. Howmany possibilities are there? Would guessing the nonce help themdecrypt the message?)arrow_forward
- Interchange Base Points AlgorithmOutput: a base B = [131,132..... 13j-1, 13L.+1, 13j, 13j+2, 13j+3..... 13k] for G; a strong generating set relative to B; procedure interchange(var B: sequence of points; vat S: set of elements; j: 1..k-1);Interchange Base Points AlgorithmInput: a group G; a base [131,132..... 13k] for G and a strong generating set; an integer j between 1 and k-l; Output: a base [131,132..... 13k] for G and a strong generating set.arrow_forwardC++ only. Do not copy. Correct code will upvoted else downvote. We should call an integer cluster a1,a2,… ,a decent if ai≠i for every I. Let F(a) be the number of sets (i,j) (1≤i<j≤n) to such an extent that ai+aj=i+j. Suppose that a cluster a1,a2,… ,an is fantastic if: an is decent; Write a program that outputs the coordinates of elements from a array of size n×m, which is filled likesnake. Snake array - which is filled in like this: • For all j and k (j < k): aij > a(i+1)j • If i is even then, for all j and k (j < k) : aij > aik . • If i is odd then, for all j and k (j < k) : aij < aik. Here is an example of 3×4 Snake array 25 23 20 19 13 15 17 18 12 10 9 8 Input l≤ai≤r for every I; F(a) is the most extreme conceivable among all great varieties of size n. Given n, l and r, work out the number of superb exhibits modulo 109+7 Input The primary line contains a solitary integer t (1≤t≤1000) — the number of experiments. The sole line of each…arrow_forwardN is not computing what is wrong with code?arrow_forward
- Write Algorithm to Removing Some RedundanciesInput : a base B = [91, ~2 ..... ~k];a strong generating set S of a group G;Output 9 a subset T of S that is also a strong generating set of G relative to B; write accurate ans otherwise you will get downvotearrow_forwardList experimental_data is read from input. The elements of experimental_data are separated into thirds. The first third of the data is collected in the beginning of a month, the second third in the middle of a month, and the last third in the end of a month. Integer slice_size represents the number of data in each third. Assign slice_size with len(experimental_data) // 3. Assign early_group with a slice of experimental_data from the beginning up to and excluding index slice_size. Assign mid_group with a slice of experimental_data from index slice_size up to and excluding index (2 * slice_size). Assign late_group with a slice of experimental_data from index (2 * slice_size) up to and including the end.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
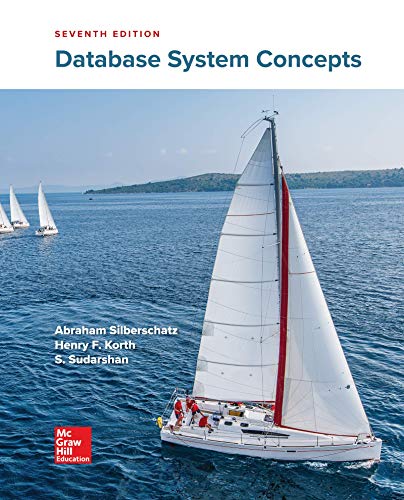
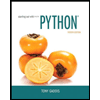
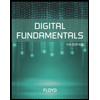
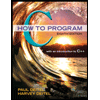
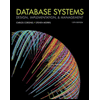
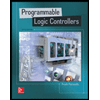