Random Numbers: Most computer programs do the same thing every time they run; programs like that are called deterministic. Usually, determinism is a good thing, since we expect the same calculation to yield the same result. But for some applications, we want the computer to be unpredictable. Games are an obvious example, but there are many others, like scientific simulations. We have already seen java.util.Random, which generates pseudorandom numbers. The method nextInt takes an integer argument, n, and returns a random integer between 0 and n - 1. Assignment: For this assignment you will write a program in java to simulate a guessing game. Your program will generate a random number in a certain range (let the user define the range) and ask the user to guess what number it is. Give the user a certain number of tries while you narrow down the possibilities.
Random Numbers:
Most computer programs do the same thing every time they run; programs like that are called deterministic. Usually, determinism is a good thing, since we expect the same calculation to yield the same result. But for some applications, we want the computer to be unpredictable. Games are an obvious example, but there are many others, like scientific simulations.
We have already seen java.util.Random, which generates pseudorandom numbers. The method nextInt takes an integer argument, n, and returns a random integer between 0 and n - 1.
Assignment:
For this assignment you will write a program in java to simulate a guessing game. Your program will generate a random number in a certain range (let the user define the range) and ask the user to guess what number it is. Give the user a certain number of tries while you narrow down the possibilities. For example, let's say the number generated is 67 and the user has 5 tries, this could be your program's output:
This is a guessing game.
Please enter the lower and highest values for the range: 1 100
I'm thinking of number between 1 and 100. Guess what it is:
50
Too low! Try again:
75
Too high! Try again:
65
To low! Try again:
70
To high! Try again:
68
You lost! The number was 67.

Step by step
Solved in 2 steps with 3 images

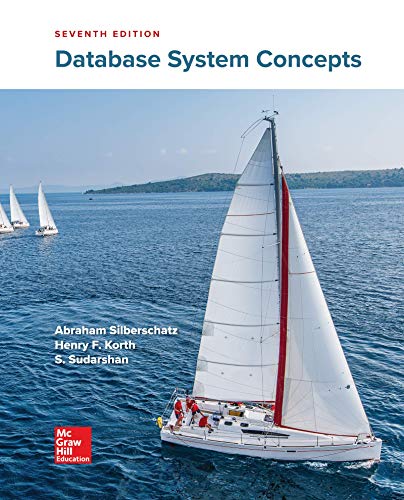
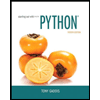
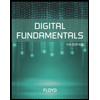
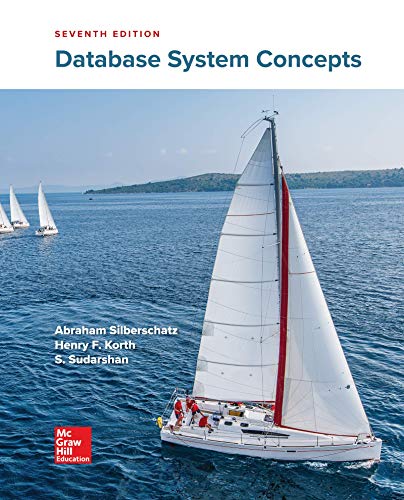
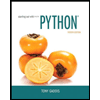
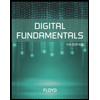
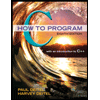
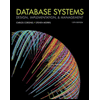
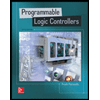