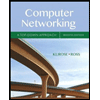
Question1: Continue with Vehicle class:
a) Copy the previous
b) implement a non-member function names output that will print all information of
Vehicle object.
Use following main() to test your class.
int main(){
Vehicle a("Ford","Focus",25000);
output(a);
This program will print:
Brand: Ford
Model: Focus
Price: 25000
}
#include <iostream>
using namespace std;
class Vehicle {
private:
string brand;
string model;
int price;
public:
void setBrand(string brand) {
this->brand = brand;
}
void setModel(string model) {
this->model = model;
}
void setPrice(int price) {
this->price = price;
}
string getBrand() {
return brand;
}
string getModel() {
return model;
}
int getPrice() {
return price;
}
};
int main() {
Vehicle a;
a.setBrand("BMW");
a.setModel("X5");
a.setPrice(60000);
cout<<a.getBrand()<<endl;
cout<<a.getModel()<<endl;
cout<<a.getPrice()<<endl;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- C++ Coding Help (Operators: new delete) Assign pointer myGas with a new Gas object. Call myGas's Read() to read the object's fields. Then, call myGas's Print() to output the values of the fields. Finally, delete myGas. Ex: If the input is 14 45, then the output is: Gas's volume: 14 Gas's temperature: 45 Gas with volume 14 and temperature 45 is deallocated. #include <iostream>using namespace std; class Gas { public: Gas(); void Read(); void Print(); ~Gas(); private: int volume; int temperature;};Gas::Gas() { volume = 0; temperature = 0;}void Gas::Read() { cin >> volume; cin >> temperature;} void Gas::Print() { cout << "Gas's volume: " << volume << endl; cout << "Gas's temperature: " << temperature << endl;} Gas::~Gas() { // Covered in section on Destructors. cout << "Gas with volume " << volume << " and temperature " << temperature << " is deallocated."…arrow_forwardC++ coding project just need some help getting the code thank you!arrow_forwarda. Write a class BumbleBee that inherits from Insect. Add the public member function void sting() const . This function simply prints "sting!" and a newline. b. Write a class GrassHopper that inherits from Insect. Add the public member function void hop() const . This function simply prints "hop!" and a newline. When you are done your program output should look exactly like the output provided at the end of the file. Do not modify the main program. Do not modify the Insect class.arrow_forward
- **please see question attached** (previous program below) #include <iostream>using namespace std;class House{//instance variablesprivate:string location;int price; public://constructorsHouse(){location = "TBD";price = 0;}House(string location,int price){this->location = location;this->price = price;}//Get and set methodsvoid setLocation(string location){this->location = location;}string getLocation(){return location;}void setPrice(int price){this->price = price;}int getPrice(){return price;} };//member functionvoid output(House a){cout <<"Location: " << a.getLocation()<<endl;cout <<"Price: "<<a.getPrice()<<endl;}int main(){House a("1234 qcc st, Bayside, NY",1000000);output(a);House b;output(b); return 0;}arrow_forwardQ1: Implement a class called Animal. a) A class named Animal b) Private data members: species and age. c) Accessors and Mutators function for species, and age. Use following main() to test your class. int main() { Animal a; a.setSpecies("dog"); a.setAge(10); cout<arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
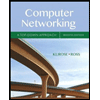
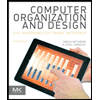
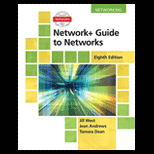
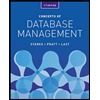
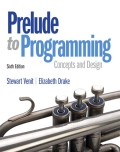
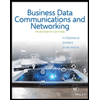