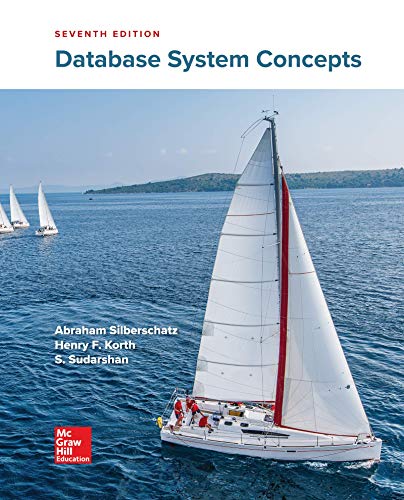
Question:
Submit document with methods for your automobile class, and pseudo code indicating functionality of each method.
Example:
public String RemoveVehicle(String
autoMake, String autoModel, String autoColor, int autoYear)
If
values entered match values stored in private variables
remove vehicle information
else
return message indicating mismatch
Assignment details/background
Create an automobile class that will be used by a dealership as a vehicle inventory program. The following attributes should be present in your automobile class:
private string make
private string model
private string color
private int year
private int mileage
Your program should have appropriate methods such as:
default constructor
parameterized constructor
add a new vehicle method
list vehicle information (return string array)
remove a vehicle method
update vehicle attributes method.
All methods should include try..catch constructs. Except as noted all methods should return a success or failure message (failure message defined in "catch").
Create an additional class to call your automobile class (e.g., Main or AutomobileInventory). Include a try..catch construct and print it to the console.
Call automobile class with parameterized constructor (e.g., "make, model, color, year, mileage").
Then call the method to list the values. Loop through the array and print to the screen.
Call the remove vehicle method to clear the variables.
Print the return value.
Add a new vehicle.
Print the return value.
Call the list method and print the new vehicle information to the screen.
Update the vehicle.
Print the return value.
Call the listing method and print the information to the screen.
Display a message asking if the user wants to print the information to a file (Y or N).
Use a scanner to capture the response. If "Y", print the file to a predefined location (e.g., C:\Temp\Autos.txt). Note: you may want to create a method to print the information in the main class.
If "N", indicate that a file will not be printed.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- CSC 1302: PRINCIPLES OF COMPUTER SCIENCE II Lab 6 How to Submit Please submit your answers to the lab instructor once you have completed. Failure to submit will result in a ZERO FOR THIS LAB. NO EXCEPTIONS. The class diagram with four classes Student, Graduate, Undergraduate, and Exchange is given. Write the classes as described in the class diagram. The fields and methods for each class is given below. Student Exchange Graduate Undergraduate Student: Fields: major (String) gpa (double) creditHours (int) Methods: getGpa: returns gpa getYear: returns “freshman”, “sophomore”, “junior” or “senior” as determined by earned credit hours: Freshman: Less than 32 credit hours Sophomore: At least 32 credit hours but less than 64 credit hours Junior: At least credit hours 64 but less than 96 credit hours Senior: At least 96 credit hours Graduate:…arrow_forwardclass Widget: """A class representing a simple Widget === Instance Attributes (the attributes of this class and their types) === name: the name of this Widget (str) cost: the cost of this Widget (int); cost >= 0 === Sample Usage (to help you understand how this class would be used) === >>> my_widget = Widget('Puzzle', 15) >>> my_widget.name 'Puzzle' >>> my_widget.cost 15 >>> my_widget.is_cheap() False >>> your_widget = Widget("Rubik's Cube", 6) >>> your_widget.name "Rubik's Cube" >>> your_widget.cost 6 >>> your_widget.is_cheap() True """ # Add your methods here if __name__ == '__main__': import doctest # Uncomment the line below if you prefer to test your examples with doctest # doctest.testmod()arrow_forwardSelect the correct option for the question shown below.arrow_forward
- In order to do a range of tasks, both code and forms employ the ACTION and METHOD attributes.arrow_forwardComputer programmingarrow_forwardCar Class Project The car classwill havethe following attributes: •year: an integer that holds the car's model year •model: a string that holds the make of the car •make: a string that holds the model of the car •speed: an integer that holds the car's current speed Your class should contain thefollowing: A docstring that briefly describes the class and lists the attributes. The docstring will serve as the documentation for your class. •A constructor (__init__ method) that takes the car's year model and make as optional arguments. The constructor will set the value of the speed attribute to 0.•An __str__ method that returns the car's year model and makein a string. •To test your class, create acar object and use the print function to verify that the constructor and __str__ methodsare working correctly. •An accessor method which returns the value stored in the speed instance variable. Call this method getSpeed(). •A modifier method called accelerate() which adds 5 to the speed variable…arrow_forward
- Describe the class's private and public components.arrow_forwardDouble Bubble For this exercise you need to create a Bubble class and construct two instances of the Bubble object. You will then take the two Bubble objects and combine them to create a new, larger combined Bubble object. This will be done using functions that take in these Bubble objects as parameters. The Bubble class contains one data member, radius_, and the corresponding accessor and mutator methods for radius_, GetRadius and SetRadius. Create a member function called CalculateVolume that computes for the volume of a bubble (sphere). Use the value 3.1415 for PI. Your main function has some skeleton code that asks the user for the radius of two bubbles. You will use this to create the two Bubble objects. You will create a CombineBubbles function that receives two references (two Bubble objects) and returns a Bubble object. Combining bubbles simply means creating a new Bubble object whose radius is the sum of the two Bubble objects' radii. Take note that the CombineBubbles function…arrow_forwardCIST 1305 UNIT 10 DROP BOX ASSIGNMENT Create the class diagram and write the pseudocode for a class named Computer that holds the make, model, and amount of memory of a computer. Include methods to set the values for each data field, and include a method that displays all the values for each field.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
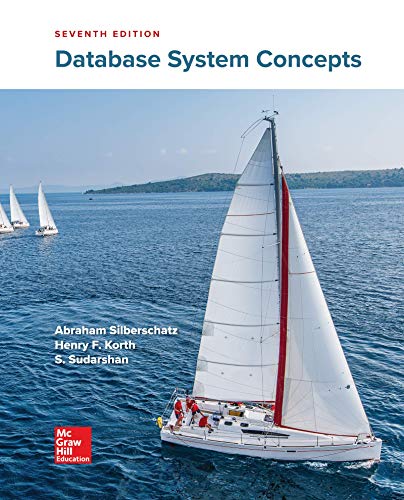
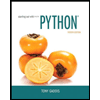
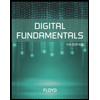
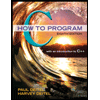
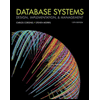
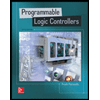