Question: May I ask for your help in giving me an idea for a C function that can sort my nodes based on their priority number through the linked list? I have my code attached below, but not the text file needed for this. I can provide a screenshot of it. Code: #include #include #include // Struct to hold each member related to my variable. typedef struct { char variable[10]; int Burst_time; int Wait_time; int Turn_time; int priority; } element_t; // Struct that acts as my node in a linked list. typedef struct priority_node { element_t data; struct priority_node *next; } node_t; // Assigning the functions here that will be used throughout main. node_t *insert_node (int priority); void display_list (); // Here is my main function. int main(void) { int i = 0; char line[25]; // Place that stores all of my text. char *variable, *priority, *Burst_time, *Wait_time; int elem, sum1 = 0, sum2 = 0, priority2; element_t elem_struct; node_t *head = NULL, *temporary; // Creating a file pointer to read stuff from the file named known 'elements.txt' // File looks like this: // Line 1: P1 2 6 14 // Line 2: P2 4 12 3 // Line 3: P3 0 3 1 // Line 4: P4 1 10 15 FILE *fptr; fptr = fopen ("elements.txt", "r"); // Looping until end of the file is reached. while (!feof(fptr)) { fgets(line, 25, fptr); // Reading in a line. // Appending each string within file to the correct pointer------ variable = strtok(line, " "); priority = strtok(NULL, " "); Burst_time = strtok(NULL, " "); Wait_time = strtok(NULL, " ");//--------------------------------- printf("Variable: %s\t\t", variable); // Testing from here to printf("Priority: %s\t\t", priority); // | printf("Burst time: %s\t\t", Burst_time); // | printf("Waiting time: %s\n", Wait_time);// v here sum1 = atoi(Burst_time) + atoi(Wait_time); // Assigning each element to the member node ----- strcpy(elem_struct.variable, variable); elem_struct.priority = atoi(priority); elem_struct.Burst_time = atoi(Burst_time); elem_struct.Wait_time = atoi(Wait_time); elem_struct.Turn_time = sum1;// ------------------------------ // Insertion function is placed here temporary = insert_node(elem_struct.priority); temporary -> next = head; head = temporary; // Just random stuff that will be used later------------------- sum1 = 0; sum2 += sum1; // Placeholder for total sum of waiting time. i++; // ------------------------------------------------------- } fclose(fptr); // Closing file pointer to prevent errors. // Printing the linked list here to show what got inserted in it. display_list(head); return0; } // Here is my print function for printing out the linked list void display_list (node_t *Head) { node_t *temporary; temporary = Head; printf("Variable Priority Burst Time Waiting Time Turnaround time\n"); while (temporary != NULL) { printf("Var: %s\t", temporary -> data.variable); printf("Pr: %d\t", temporary -> data.priority); printf("Bt: %d\t", temporary -> data.Burst_time); printf("Wt: %d\t", temporary -> data.Wait_time); printf("Turn: %d\n", temporary -> data.Turn_time); temporary = temporary -> next; } } // Here is my insertion function node_t *insert_node (int priority) { node_t *newPtr = (node_t *)malloc(sizeof(node_t)); newPtr -> data.priority = priority; newPtr -> next = NULL; return newPtr; } /* All I need now is a function that can sort my nodes based on their priority number/member */
Question:
May I ask for your help in giving me an idea for a C function that can sort my nodes based on their priority number through the linked list? I have my code attached below, but not the text file needed for this. I can provide a screenshot of it.
Code:


Note: Assuming 0 is the highest priority. If you want to take 4 as the highest priority change if(temp1->data.priority>temp2->data.priority) in the sort function to if(temp1->data.priority<temp2->data.priority). In the output, it is showing the garbage value for burst, turn around time, waiting time because in the insert you are just initializing the priority variable.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// Struct to hold each member related to my variable.
typedef struct {
char variable[10];
int Burst_time;
int Wait_time;
int Turn_time;
int priority;
} element_t;
// Struct that acts as my node in a linked list.
typedef struct priority_node {
element_t data;
struct priority_node *next;
} node_t;
// Assigning the functions here that will be used throughout main.
node_t *insert_node (int priority);
void display_list ();
node_t *sort(node_t *Head);
// Here is my main function.
int main(void) {
int i = 0;
char line[25]; // Place that stores all of my text.
char *variable, *priority, *Burst_time, *Wait_time;
int elem, sum1 = 0, sum2 = 0, priority2;
element_t elem_struct;
node_t *head = NULL, *temporary;
// Creating a file pointer to read stuff from the file named known 'elements.txt'
// File looks like this:
// Line 1: P1 2 6 14
// Line 2: P2 4 12 3
// Line 3: P3 0 3 1
// Line 4: P4 1 10 15
FILE *fptr;
fptr = fopen ("C:\\Users\\dell\\OneDrive\\Desktop\\elements.txt", "r");
// Looping until end of the file is reached.
while (!feof(fptr)) {
fgets(line, 25, fptr); // Reading in a line.
// Appending each string within file to the correct pointer------
variable = strtok(line, " ");
priority = strtok(NULL, " ");
Burst_time = strtok(NULL, " ");
Wait_time = strtok(NULL, " ");//---------------------------------
printf("Variable: %s\t\t", variable); // Testing from here to
printf("Priority: %s\t\t", priority); // |
printf("Burst time: %s\t\t", Burst_time); // |
printf("Waiting time: %s\n", Wait_time);// v here
sum1 = atoi(Burst_time) + atoi(Wait_time); // Assigning each element to the member node -----
strcpy(elem_struct.variable, variable);
elem_struct.priority = atoi(priority);
elem_struct.Burst_time = atoi(Burst_time);
elem_struct.Wait_time = atoi(Wait_time);
elem_struct.Turn_time = sum1;// ------------------------------
// Insertion function is placed here
temporary = insert_node(elem_struct.priority);
temporary -> next = head;
head = temporary;
// Just random stuff that will be used later-------------------
sum1 = 0;
sum2 += sum1; // Placeholder for total sum of waiting time.
i++; // -------------------------------------------------------
}
fclose(fptr); // Closing file pointer to prevent errors.
// Printing the linked list here to show what got inserted in it.
display_list(head);
head = sort(head);
display_list(head);
return 0;
}
// Here is my print function for printing out the linked list
void display_list (node_t *Head)
{
node_t *temporary;
temporary = Head;
printf("Variable Priority Burst Time Waiting Time Turnaround time\n");
while (temporary != NULL) {
printf("Var: %s\t", temporary -> data.variable);
printf("Pr: %d\t", temporary -> data.priority);
printf("Bt: %d\t", temporary -> data.Burst_time);
printf("Wt: %d\t", temporary -> data.Wait_time);
printf("Turn: %d\n", temporary -> data.Turn_time);
temporary = temporary -> next;
}
}
// Here is my insertion function
node_t *insert_node (int priority) {
node_t *newPtr = (node_t *)malloc(sizeof(node_t));
newPtr -> data.priority = priority;
newPtr -> next = NULL;
return newPtr;
}
node_t *sort(node_t *Head)
{
node_t *temp1,*temp2;
for(temp1=Head;temp1->next!=NULL;temp1=temp1->next)
{
for(temp2=temp1->next;temp2!=NULL;temp2=temp2->next)
{
if(temp1->data.priority>temp2->data.priority)
{
element_t d = temp1->data;
temp1->data = temp2->data;
temp2->data = d;
}
}
}
return Head;
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

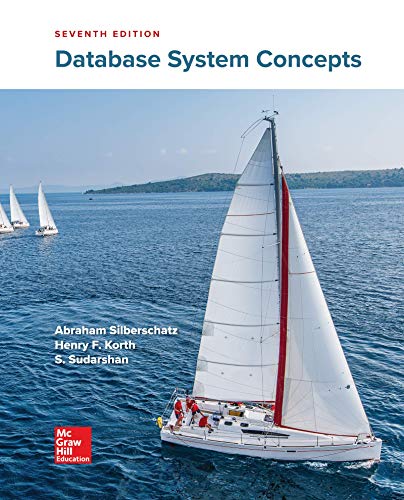
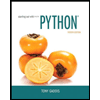
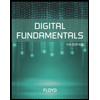
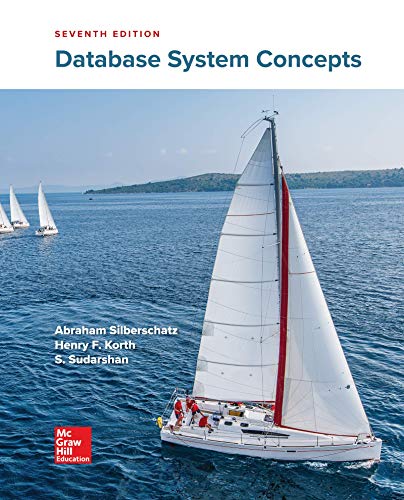
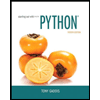
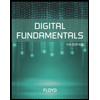
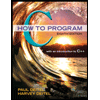
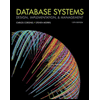
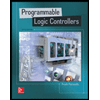