Begin constants COLUMN_ID = 0 COLUMN_NAME = 1 COLUMN_HIGHWAY = 2 COLUMN_LAT = 3 COLUMN_LON = 4 COLUMN_YEAR_BUILT = 5 COLUMN_LAST_MAJOR_REHAB = 6 COLUMN_LAST_MINOR_REHAB = 7 COLUMN_NUM_SPANS = 8 COLUMN_SPAN_DETAILS = 9 COLUMN_DECK_LENGTH = 10
# Begin constants
COLUMN_ID = 0
COLUMN_NAME = 1
COLUMN_HIGHWAY = 2
COLUMN_LAT = 3
COLUMN_LON = 4
COLUMN_YEAR_BUILT = 5
COLUMN_LAST_MAJOR_REHAB = 6
COLUMN_LAST_MINOR_REHAB = 7
COLUMN_NUM_SPANS = 8
COLUMN_SPAN_DETAILS = 9
COLUMN_DECK_LENGTH = 10
COLUMN_LAST_INSPECTED = 11
COLUMN_BCI = 12
INDEX_BCI_YEARS = 0
INDEX_BCI_SCORES = 1
MISSING_BCI = -1.0
EARTH_RADIUS = 6371
# Sample data for docstring examples
def create_example_bridge_1() -> list:
"""Return a bridge in our list-format to use for doctest examples.
This bridge is the same as the bridge from row 3 of the dataset.
"""
return [
1, 'Highway 24 Underpass at Highway 403',
'403', 43.167233, -80.275567, '1965', '2014', '2009', 4,
[12.0, 19.0, 21.0, 12.0], 65.0, '04/13/2012',
[['2013', '2012', '2011', '2010', '2009', '2008', '2007',
'2006', '2005', '2004', '2003', '2002', '2001', '2000'],
[MISSING_BCI, 72.3, MISSING_BCI, 69.5, MISSING_BCI, 70.0, MISSING_BCI,
70.3, MISSING_BCI, 70.5, MISSING_BCI, 70.7, 72.9, MISSING_BCI]]
]
def create_example_bridge_2() -> list:
"""Return a bridge in our list-format to use for doctest examples.
This bridge is the same as the bridge from row 4 of the dataset.
"""
return [
2, 'WEST STREET UNDERPASS',
'403', 43.164531, -80.251582, '1963', '2014', '2007', 4,
[12.2, 18.0, 18.0, 12.2], 61.0, '04/13/2012',
[['2013', '2012', '2011', '2010', '2009', '2008', '2007',
'2006', '2005', '2004', '2003', '2002', '2001', '2000'],
[MISSING_BCI, 71.5, MISSING_BCI, 68.1, MISSING_BCI, 69.0, MISSING_BCI,
69.4, MISSING_BCI, 69.4, MISSING_BCI, 70.3, 73.3, MISSING_BCI]]
]
def create_example_bridge_3() -> list:
"""Return a bridge in our list-format to use for doctest examples.
This bridge is the same as the bridge from row 33 of the dataset.
"""
return [
3, 'STOKES RIVER BRIDGE', '6',
45.036739, -81.33579, '1958', '2013', '', 1,
[16.0], 18.4, '08/28/2013',
[['2013', '2012', '2011', '2010', '2009', '2008', '2007',
'2006', '2005', '2004', '2003', '2002', '2001', '2000'],
[85.1, MISSING_BCI, 67.8, MISSING_BCI, 67.4, MISSING_BCI, 69.2,
70.0, 70.5, MISSING_BCI, 75.1, MISSING_BCI, 90.1, MISSING_BCI]]
]
def create_example_bridges() -> List[list]:
"""Return a list containing three unique example bridges.
The bridges contained in the list are from row 3, 4, and 33 of the dataset
(in that order).
"""
return [
create_example_bridge_1(),
create_example_bridge_2(),
create_example_bridge_3()
]
# Part 1 - Querying the data
def find_bridge_by_id(bridges: List[list], bridge_id: int) -> list:
"""Return the data for the bridge with id bridge_id from bridges.
If there is no bridge with the given id in bridges, then return an empty
list.
>>> example_bridges = create_example_bridges()
>>> find_bridge_by_id(example_bridges, 4)
[]
>>> find_bridge_by_id(example_bridges, 2) == create_example_bridge_2()
True
"""
def find_bridges_in_radius(bridges: List[list], lat: float, lon: float,
radius: int, exclusions: List[int]) -> List[int]:
"""Return the IDs of the bridges that are within radius kilometres from
location (lat, lon). Include bridge IDs that are exactly radius kilometres
away. The bridge IDs in exclusions are not included in the result.
Preconditions:
- (lat, lon) is a valid location on Earth
- radius > 0
>>> example_bridges = create_example_bridges()
>>> find_bridges_in_radius(example_bridges, 43.10, -80.15, 50, [])
[1, 2]
>>> find_bridges_in_radius(example_bridges, 43.10, -80.15, 50, [1, 2])
[]
"""
def get_bridge_condition(bridges: List[list], bridge_id: int) -> float:
"""Return the most recent BCI score of the bridge in bridges with id
bridge_id.
The most recent BCI score is the BCI score given to the bridge in the
highest (i.e., most recent) year. If there is no score for every year,
return MISSING_BCI.
>>> example_bridges = create_example_bridges()
>>> get_bridge_condition(example_bridges, 1)
72.3
"""
def calculate_average_condition(bridge: list, start: int, stop: int) -> float:
"""Return the average BCI score of bridge between the year start (inclusive)
and stop (exclusive).
Scores with the value MISSING_BCI are invalid and should not be included in
the average. If there are no valid scores between start and stop, return
0.0.
Preconditions:
- start > 0
- start <= stop
>>> bridge_1 = create_example_bridge_1()
>>> calculate_average_condition(bridge_1, 2005, 2013)
70.525
>>> calculate_average_condition(bridge_1, 2013, 2021)
0.0
"""


Step by step
Solved in 2 steps

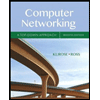
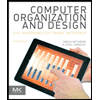
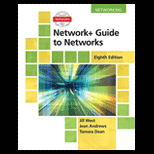
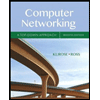
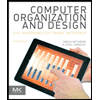
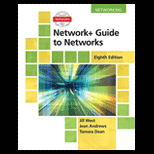
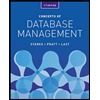
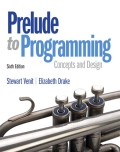
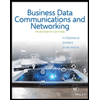