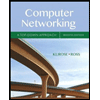
QUESTION 4 (use c++ to answer the following question)
-
Note: You need to add required constructors/destructors, member functions or data members/variables in your program to complete its execution.
Suppose we are designing a record-keeping program that has records for salaried
employees and hourly employees. There is a natural hierarchy for grouping these classes.
These are all classes of people who share the property of being employees.
Employees who are paid an hourly wage are one subset of employees. Another
subset consists of employees who are paid a fixed wage each month or week. Although the program may not need any type corresponding to the set of all employees, thinking in terms of the more general concept of employees can be useful. For example, all employees have names, Social Security numbers (ssn) and net pay, and the member functions for setting and changing the name, ssn and netpay would be same for both hourly and salaried employees.Implement the above mentioned record keeping program by creating an Employee class with data members name, ssn, and netpay. Include the default and parameterized constructors, member functions to set and get the data members. Also, write a printCheck function that should display error message that “printCheck Function Called for an undifferentiated employee”
Create HourlyEmployee class (inherit from Employee class) with data members wageRate and hours (think rationally about the data types). Include the default constructor, parameterized constructor, setter, getter and printCheck function. The printCheck function should display the following output:
Pay to the order of John Blue
The sum of 10000 Dollars
Check Stub: NOT NEGOTIABLE
Employee Number: <ssn>
Hourly Employee
Hours Worked: <hours> hours Rate: <wageRate> Pay: <netpay>
Similarly, create SalariedEmployee, inherit from Employee class, with data member salary. Define the constructors, setter and getter methods and printCheck function. The printCheck function output should be as follows:
_____________________________________________________________________
Pay to the order of John Blue
The sum of 10000 Dollars
Check Stub: NOT NEGOTIABLE
Employee Number: <ssn>
Salaried Employee
Regular Pay: <salary>

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Please see attached.arrow_forwardImplement a nested class composition relationship between any two class types from the following list: Advisor Вook Classroom Department Friend Grade School Student Teacher Tutor Write all necessary code for both classes to demonstrate a nested composition relationship including the following: a. one encapsulated data member for each class b. inline default constructor using constructor delegation for each class c. inline one-parameter constructor for each class d. inline accessors for all data members e. inline mutators for all data membersarrow_forwardStruggling with a c++ task. The class dateType was designed to implement the date in a program, but the member function setDate and the constructor do not check whether the date is valid before storing the date in the member variables. Rewrite the definitions of the function setDate and the constructor so that the values for the month, day, and year are checked before storing the date into the member variables. Add a member function, isLeapYear, to check whether a year is a leap year. Moreover, write a test program to test your class. Input should be format month day year with each separated by a space. Output should resemble the following: Date #: month-day-year An example of the program is shown below: Date 1: 3-15-2008 this is a leap year If the year is a leap year, print the date and a message indicating it is a leap year, otherwise print a message indicating that it is not a leap year. The header file for the class dateType has been provided: #ifndef date_H #define date_H…arrow_forward
- Write program in C++ using inhertiance and polymorphism. A car dealership wants you to keep track of their sold and leased vehicles. You have realized that all vehicles have a make, model and vehicle identification number so you are going to factor those attributes out and put them in the base class as private attributes. Additionally you realize that you need to create two derived classes: one that keeps track of vehicles that are sold and the other that keeps track of ones that are leased. Vehicles that are sold have a sale date and sale amount. Vehicles that are leased have a monthly lease payment and terms of the lease (number of years). For all classes, create a proper overloaded constructor and display method that properly displays the class’s attributes. For your display method make sure you are using proper run-time polymorphism techniques so that your code calls the correct display methods. Complete main.cpp and follow comments for instruction Given code of .cpp and .h classes…arrow_forwardWhat is the main difference between a struct and a class? (More than 1 answer can be chosen)arrow_forwardAn Abstract Data Type's interface is comprised of what? Here's where you put your reply.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
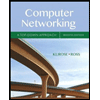
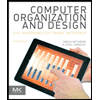
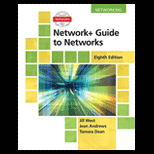
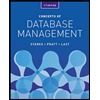
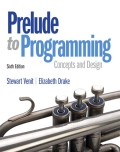
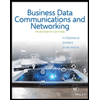