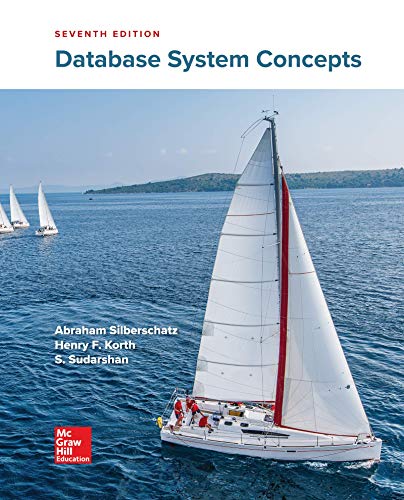
Q27 for java programing:
public static void main(String[] args) {
Dog[] dogs = { new Dog(), new Dog()};
for(int i = 0 ; i < dogs.length; i++)
dogs[i].wo();
Cat[] cats = { new Cat(),new Cat(),new Cat()};
for(int i = 0 ; i < cats.length; i++)
cats[i].me();
System.out.print(Dog.getCount()+" woofs and");
System.out.println(Cat.getCount()+" mews");
System.out.println("=====>>>"+decision()); }
class Counter
{ private static int count;
public static void inc() { count++;}
public static int getCount() {return count;}
}
class Dog extends Counter{
public Dog(){}
public void wo(){inc();}
}
class Cat extends Counter{
public Cat(){}
public void me( ){inc();}
}
The Correct answer:
public static void main(String[] args) {
Dog[] dogs = { new Dog(), new Dog()};
for(int i = 0 ; i < dogs.length; i++)
dogs[i].wo();
Cat[] cats = { new Cat(),new Cat(),new Cat()};
for(int i = 0 ; i < cats.length; i++)
cats[i].me();
System.out.print(Dog.getCount()+" woofs and");
System.out.println(Cat.getCount()+" mews");
System.out.println("=====>>>"+decision()); }
class Counter
{ private static int count;
public static void inc() { count++;}
public static int getCount() {return count;}
}
class Dog extends Counter{
public Dog(){}
public void wo(){inc();}
}
class Cat extends Counter{
public Cat(){}
public void me( ){inc();}
}
The Correct answer:

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Javaarrow_forwardWhat is causing this error?arrow_forwardpublic class arrayOutput { public static void main (String [] args) { final int NUM_ELEMENTS = 3; int[] userVals = new int [NUM_ELEMENTS]; int i; int sumVal; userVals [0] = 3; userVals [1] = 5; userVals [2] = 8; Type the program's output sumVal = 0; for (i = 0; i < userVals.length; ++i) { sumVal= sumVal + userVals [i]; System.out.println (sumVal); } ? ? ??arrow_forward
- USING C++ Implement the Rectangle class below: *******RECTANGLE CLASS******* class Rectangle { private: double width; double length; public: void setWidth(double); void setLength(double); double getWidth() const; double getLength() const; double getArea() const; }; *******END OF RECTANGLE CLASS******* Enhance the Rectangle class with two new member functions: double getPerimeter() - returns the perimeter of the rectangle, i.e. 2*width+2*length bool isSquare() - returns true if the rectangle is a square, i.e. length is equal to width, returns false if not Demonstrate your class works with the main function that instantiates a Rectangle object and shows sample output for these functions workingarrow_forwardFind the errors in this code: public class TstArray { public static void main(String args[]){ int a[] = {5, 4, 9}; int avg; int total; for(int i=0; i< a.length; i++){ total = 0; total += a[i]; } avg = total/a.length; } }arrow_forwardmethods and parameters in javaarrow_forward
- Image attachedarrow_forwardpublic static int countIt (int n) { int count = 0; for (int i = n; i > 0; i /= 2) for (int j = 0; j < 10; j++) count += 1; return count; } Big-O notation: Explanation:arrow_forwardOOP (Object-Oriented Programming) in Java Applying the composite pattern, you may create a replica of any environment you choose. Any number of media may be used, from photographs to simulators to movies to video games, and so on.arrow_forward
- Code 16-1 /** This class has a recursive method. */ public class EndlessRecursion { public static void message() { System.out.println("This is a recursive method."); message(); } } Code 16.2 /** This class has a recursive method message, which displays a message n times. */ public class Recursive { public static void messge (int n) { if (n>0) { System.out.println (" This is a recursive method."); message(n-1); } } } Task #1 Tracing Recursive Methods 1. Copy the file Recursion.java (see Code Listing 16.1) from the Student Files or as directed by your instructor. 2. Run the program to confirm that the generated answer is correct. Modify the factorial method in the following ways: a. Add these lines above the first if statement: int temp; System.out.println("Method call -- " + "calculating " + "Factorial of: " + n); Copyright © 2019 Pearson Education, Inc., Hoboken NJ b. Remove this line in the recursive section at the end of the method: return…arrow_forwardvoid listEmployees (void) { for (int i=0; i 10000. Make a guess about why the comparison function takes 2 struct Employee parameters (as opposed to struct Employee *) **arrow_forwardThis is a subclass and doesn't has a main method yet. class Vector{Object items[];int length;int size; void Grow(){// Duplicate sizesize = size * 2; // Allocate new itemsObject new_items[] = new Object[size]; // Copy old itemsfor (int i = 0; i < length; i++)new_items[i] = items[i]; // Discard old itemsitems = new_items; // MessageSystem.out.println("Growing capacity to " + size + " elements");} public Vector(){size = 2;items = new Object[2];} public void Print(){// MessageSystem.out.println("Content:"); // Traversefor (int i = 0; i < length; i++)System.out.println(items[i]);} public void Insert(int index, Object item){// Check indexif (index < 0 || index > length){System.out.println("Invalid index");return;} // Grow if necessaryif (length == size)Grow(); // Shiftfor (int i = length - 1; i >= index; i--)items[i + 1] = items[i]; // Insertitems[index] = item; // One more itemlength++; // MessageSystem.out.println("Inserted " + item);} public void Add(Object…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
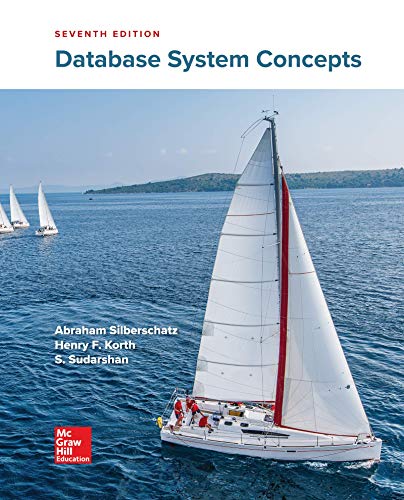
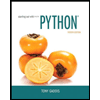
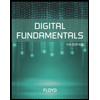
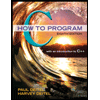
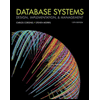
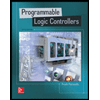