Python question please include all steps and screenshot of code. Also please provide a docstring, and comments throughout the code, and test the given examples below. Thanks. Implement function heads() that takes no input and simulates a sequence of coin flips. The simulation should continue as long as 'HEAD' is flipped. When the outcome of a flip is 'TAIL', the function should return the number of 'HEAD' flips made up to that point. For example, if the simulation results in outcomes 'HEAD', 'HEAD', 'HEAD', 'TAIL', the function should return 3. NOTE: Recall that random.choice(['HEAD', 'TAIL']) returns 'HEAD' or 'TAIL' with equal probability. >>> heads() 0 # TAIL is the outcome of the first coin flip >>> heads() 2 # The coin flips were HEAD, HEAD, TAIL >>> heads() 1 # The coin flips were HEAD, TAIL >>> heads() 5 # The coin flips were HEAD, HEAD, HEAD, HEAD, HEAD, TAIL
Python question please include all steps and screenshot of code. Also please provide a docstring, and comments throughout the code, and test the given examples below. Thanks.
Implement function heads() that takes no input and simulates a sequence of coin flips.
The simulation should continue as long as 'HEAD' is flipped. When the outcome of a flip
is 'TAIL', the function should return the number of 'HEAD' flips made up to that point.
For example, if the simulation results in outcomes 'HEAD', 'HEAD', 'HEAD', 'TAIL', the
function should return 3. NOTE: Recall that random.choice(['HEAD', 'TAIL']) returns
'HEAD' or 'TAIL' with equal probability.
>>> heads()
0 # TAIL is the outcome of the first coin flip
>>> heads()
2 # The coin flips were HEAD, HEAD, TAIL
>>> heads()
1 # The coin flips were HEAD, TAIL
>>> heads()
5 # The coin flips were HEAD, HEAD, HEAD, HEAD, HEAD, TAIL

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

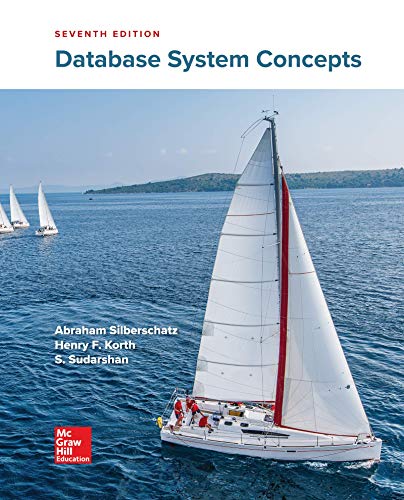
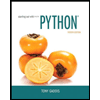
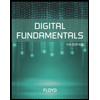
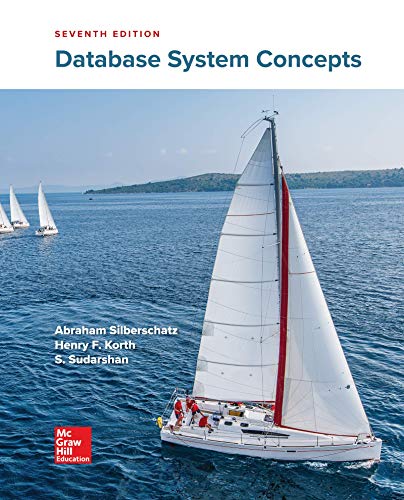
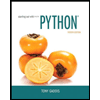
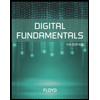
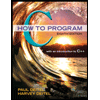
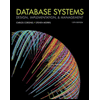
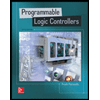