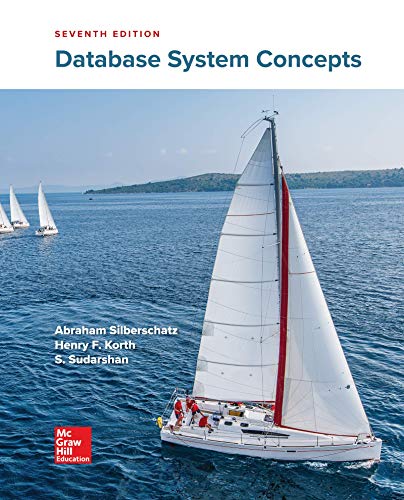
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
thumb_up100%
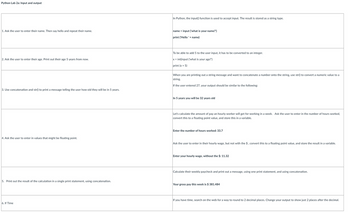
Transcribed Image Text:Python Lab 2a: Input and output
1. Ask the user to enter their name. Then say hello and repeat their name.
2. Ask the user to enter their age. Print out their age 5 years from now.
3. Use concatenation and str() to print a message telling the user how old they will be in 5 years.
4. Ask the user to enter in values that might be floating point.
5. Print out the result of the calculation in a single print statement, using concatenation.
6. If Time
In Python, the input() function is used to accept input. The result is stored as a string type.
name = input ('what is your name?')
print ('Hello ' + name)
To be able to add 5 to the user input, it has to be converted to an integer.
x = int(input ('what is your age?')
print (x + 5)
When you are printing out a string message and want to concatenate a number onto the string, use str() to convert a numeric value to a
string.
If the user entered 27, your output should be similar to the following:
In 5 years you will be 32 years old
Let's calculate the amount of pay an hourly worker will get for working in a week. Ask the user to enter in the number of hours worked,
convert this to a floating point value, and store this in a variable.
Enter the number of hours worked: 33.7
Ask the user to enter in their hourly wage, but not with the $, convert this to a floating point value, and store the result in a variable.
Enter your hourly wage, without the $: 11.32
Calculate their weekly paycheck and print out a message, using one print statement, and using concatenation.
Your gross pay this week is $ 381.484
If you have time, search on the web for a way to round to 2 decimal places. Change your output to show just 2 places after the decimal.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 2. Need help creating a SPIM program for question #1.arrow_forwardCalculating Truth Value of Formulas If A, B, and C are true statements and X, Y, and Z are false statements, calculate the truth value of the following formulas. ~(((~A & B) & (~X & Z)) & ~((A & ~B) v ~(~Y & ~Z))) X is False; H, G are unknown. Can you calculate the value of the following formulas? Yes, or No. Show and explain. (G -> (H -> G)) -> X Yes, or No. Show and explain.arrow_forwardDon't give me AI generated answer or plagiarised answer.arrow_forward
- A and BonlyIn this program, you are asked to write a program in assembly which works as a simple calculator.The program will get two integer numbers, and based on the requested operation, the result shouldbe shown to the user.a. The program should print a meaningful phrase for each input, and the result.i. “Enter the first number”ii. “Enter the second number”iii. “Enter the operation type”iv. “The result is”b. The user should enter 0, 1, and 2 to tell the program the types of operation add, sub, andmultiply, respectively.c. How many registers do you need to implement this program?d. What system calls do you need to write this program?arrow_forwardDevelop a pseudocode or flowchart for all the following problems : 1. Write a program which will display “I AM GOOD” on the computer screen for 10x2. Write a program which will display “I AM GREAT” on the computer screen for 100x3. Write a program which will display “I AM SUPERMAN” on the computer screen for 1000x4. Accept two integers from computer user and check whether they are equal or not.5. Write a program to check whether a given number is even or odd.6. Write a program to check whether a given number is positive or negative7. Write a program to read the value of an integer m and display the value of n is 1 when m is larger than 0, 0when m is 0 and -1 when m is less than 08. Write a program which accept the user’s height in cm then display :a. You are so tall → if the height is more than 180cmb. You are not that tall → if the height is between 100 – 179cmc. You are short ha ha ha → if the height is less than 100cm9. Write a program to read 10 numbers from keyboard and find their…arrow_forward3. Read input two positive integers p1, p2 from the user and check whether both the integers have the same last digit or not. Programming language: JAVAarrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
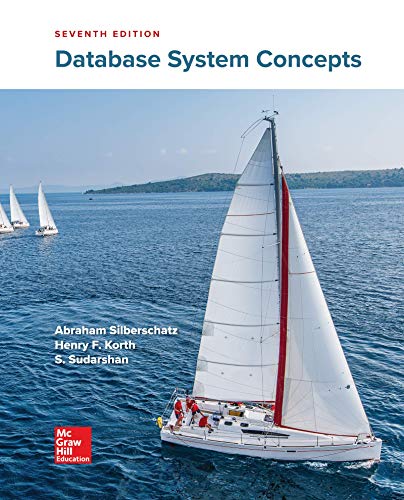
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
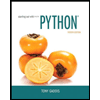
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
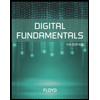
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
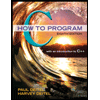
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
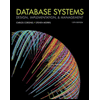
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
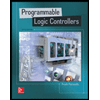
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education