python dont use input use return In this question, you will complete the FootballPlayer class, which is used to compute some features of a Football Player. The class should be constructed with the name and age, as well as shooting, passing, tackling and saving skill points of a player. The constructor method as well as the defenderScore method is provided below. You should implement the remaining methods. The definitons of the methods are presented below as comments. An example execution and the corresponding outputs are provided below: >>> burkay = FootballPlayer("Burkay", 42, 15, 30, 10, 15) >>> cemil = FootballPlayer("Cemil", 30, 70, 30, 45, 20) >>> ronaldo = FootballPlayer("Ronaldo", 36, 85, 95, 35, 5) >>> print(burkay) (Burkay,42,13640000) >>> print(cemil) (Cemil,30,144270000) >>> print(ronaldo) (Ronaldo,36,322445000) >>> print(burkay > cemil) False >>> print(ronaldo > burkay) True """ class FootballPlayer: def __init__(self, na, ag, sh, pa, ta, sa): # age is in [18,40] # skills are in [10,100] self.name = na self.age = ag self.shooting = sh self.passing = pa self.tackling = ta self.saving = sa def defenderScore(self): # The defensive score is defined as 80% tackling, 15% passing and 5% shooting # It should be reported as integer return int(0.80 * self.tackling + 0.15 * self.passing + 0.05 * self.shooting) def midfielderScore(self): # The midfielder score is defined as 50% passing, 25% shooting and 25% tackling # It should be reported as integer return # Remove this line to answer this question def forwardScore(self): # The forward score is defined as 70% shooting, 25% passing and 5% tackling # It should be reported as integer return # Remove this line to answer this question def goalieScore(self): # The goalie score is defined as 90% saving and 10% passing # It should be reported as integer return # Remove this line to answer this question def playerValue(self): # Player value is defined as # * 15000$ per unit of the square of defensive score # * 25000$ per unit of the square of midfielder score # * 20000$ per unit of the square of forward score # * 5000$ per unit of the square of goalie score # * -30000$ per (age-26)^2 # A player's value is never reported as negative. The minimum is 0. # It should be reported as integer return # Remove this line to answer this question def __str__(self): # This method returns a string representation of the player, such as # "(name,age,playerValue)" return # Remove this line to answer this question def __gt__(self, other): # This method returns True if the player value of self is greater than # the player value of other. Otherwise, it returns False. return # Remove this line to answer this question
python
dont use input
use return
In this question, you will complete the FootballPlayer class, which is used to
compute some features of a Football Player. The class should be constructed with
the name and age, as well as shooting, passing, tackling and saving skill
points of a player. The constructor method as well as the defenderScore method
is provided below. You should implement the remaining methods. The definitons
of the methods are presented below as comments.
An example execution and the corresponding outputs are provided below:
>>> burkay = FootballPlayer("Burkay", 42, 15, 30, 10, 15)
>>> cemil = FootballPlayer("Cemil", 30, 70, 30, 45, 20)
>>> ronaldo = FootballPlayer("Ronaldo", 36, 85, 95, 35, 5)
>>> print(burkay)
(Burkay,42,13640000)
>>> print(cemil)
(Cemil,30,144270000)
>>> print(ronaldo)
(Ronaldo,36,322445000)
>>> print(burkay > cemil)
False
>>> print(ronaldo > burkay)
True
"""
class FootballPlayer:
def __init__(self, na, ag, sh, pa, ta, sa):
# age is in [18,40]
# skills are in [10,100]
self.name = na
self.age = ag
self.shooting = sh
self.passing = pa
self.tackling = ta
self.saving = sa
def defenderScore(self):
# The defensive score is defined as 80% tackling, 15% passing and 5% shooting
# It should be reported as integer
return int(0.80 * self.tackling + 0.15 * self.passing + 0.05 * self.shooting)
def midfielderScore(self):
# The midfielder score is defined as 50% passing, 25% shooting and 25% tackling
# It should be reported as integer
return # Remove this line to answer this question
def forwardScore(self):
# The forward score is defined as 70% shooting, 25% passing and 5% tackling
# It should be reported as integer
return # Remove this line to answer this question
def goalieScore(self):
# The goalie score is defined as 90% saving and 10% passing
# It should be reported as integer
return # Remove this line to answer this question
def playerValue(self):
# Player value is defined as
# * 15000$ per unit of the square of defensive score
# * 25000$ per unit of the square of midfielder score
# * 20000$ per unit of the square of forward score
# * 5000$ per unit of the square of goalie score
# * -30000$ per (age-26)^2
# A player's value is never reported as negative. The minimum is 0.
# It should be reported as integer
return # Remove this line to answer this question
def __str__(self):
# This method returns a string representation of the player, such as
# "(name,age,playerValue)"
return # Remove this line to answer this question
def __gt__(self, other):
# This method returns True if the player value of self is greater than
# the player value of other. Otherwise, it returns False.
return # Remove this line to answer this question

Step by step
Solved in 3 steps with 1 images

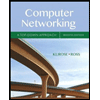
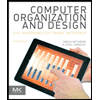
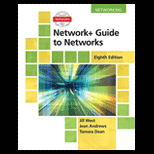
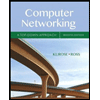
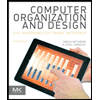
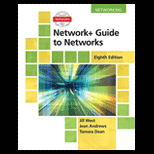
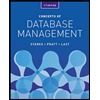
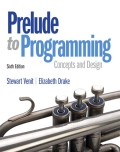
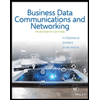