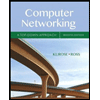
python:
def shakespeare_position(role, section):
"""
Question 2 - Regex
You are reading a Shakespeare play with your friends (as one frequently does) and are given a role.
You want to know what line immediately precedes YOUR first line in a given section so that you are ready to go
when it is your turn. Return this line as a string, excluding the character's name.
Lines will always begin with the character's name followed by a ':' and end in a "." or a "?"
Each line is separated by a single space.
THIS MUST BE DONE IN ONE LINE.
""
Args:
role (str)
section (str)
Returns:
str
section_1 = 'Benvolio: By my head, here come the Capulets. Mercutio: By my heel, I care not. ' +
'Tybalt: Gentlemen, good den - a word with one of you. Mercutio: And but one word with one of us?'
>>> shakespeare_position('Tybalt', section_1)
'By my heel, I care not.'
>>> shakespeare_position('Mercutio', section_1)
'By my head, here come the Capulets.'
"""
# section_1 = 'Benvolio: By my head, here come the Capulets. Mercutio: By my heel, I care not. ' + \
# 'Tybalt: Gentlemen, good den: a word with one of you. Mercutio: And but one word with one of us?'
# pprint(shakespeare_position('Tybalt', section_1))
# pprint(shakespeare_position('Mercutio', section_1))

Program to solve above problem using Python.
Let us Assume character name is followed by :
and Each line is end with '.'
Let us consider the section of Shakespeare playlet
Section: 'Benvolio: By my head, here come the Capulets. Mercutio: By my heel, I care not. ' +
'Tybalt: Gentlemen, good den - a word with one of you. Mercutio: And but one word with one of us?'
Roles: are Benvolio, Mercutio, Tybalt
First line by Benvolio : By my head, here come the Capulets.
Second line by Mercutio: By my heel, I care not.
Third line by Tybalt: Gentlemen, good den - a word with one of you.
Fourth line by Mercutio: And but one word with one of us?
We give Section and Roles are input to the function shakespeare_position()
You want to know what line immediately precedes, so that given role should get ready for his dialogue.
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

could you do it in one line of code and using regex please
could you do it in one line of code and using regex please
- JAVA PPROGRAM ASAP Please create this program ASAP BECAUSE IT IS MY LAB ASSIGNMENT so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #2. Word Counter (page 608) Write a method that accepts a String object as an argument and returns the number of words it contains. For instance, if the argument is “Four score and seven years ago” the method should return the number 6. Demonstrate the method in a program that asks the user to input a string and then passes it to the method. The number of words in the string should be displayed on the screen. Test Case 1 Please enter a string or type QUIT to exit:\nHello World!ENTERThere are 2 words in that string.\nPlease enter a string or type QUIT to exit:\nI have a dream.ENTERThere are 4 words in that string.\nPlease enter a string or type QUIT to exit:\nJava is great.ENTERThere are 3 words in that string.\nPlease enter a string or type QUIT to exit:\nquitENTERarrow_forwardIn cengage mindtap The credit plan at TidBit Computer Store specifies a 10% down payment and an annual interest rate of 12%. Monthly payments are 5% of the listed purchase price, minus the down payment. Write a program that takes the purchase price as input. The program should display a table, with appropriate headers, of a payment schedule for the lifetime of the loan. Each row of the table should contain the following items: The month number (beginning with 1) The current total balance owed The interest owed for that month The amount of principal owed for that month The payment for that month The balance remaining after payment The amount of interest for a month is equal to balance × rate / 12. The amount of principal for a month is equal to the monthly payment minus the interest owed.arrow_forwardWhat function do you use to copy a string to another string variable name? strcat() atof() atoi() strcpy()arrow_forward
- Python: numpy def serial_numbers(num_players):"""QUESTION 2- You are going to assign each player a serial number in the game.- In order to make the players feel that the game is very popular with a large player base,you don't want the serial numbers to be consecutive. - Instead, the serial numbers of the players must be equally spaced, starting from 1 and going all the way up to 100 (inclusive).- Given the number of players in the system, return a 1D numpy array of the serial numbers for the players.- THIS MUST BE DONE IN ONE LINEArgs:num_players (int)Returns:np.array>> serial_numbers(10)array([1. 12. 23. 34. 45. 56. 67. 78. 89. 100.])>> serial_numbers(12)array([1. 10. 19. 28. 37. 46. 55. 64. 73. 82. 91. 100.])""" # print(serial_numbers(10)) # print(serial_numbers(12))arrow_forwardX609: Magic Date A magic date is one when written in the following format, the month times the date equals the year e.g. 6/10/60. Write code that figures out if a user entered date is a magic date. The dates must be between 1 - 31, inclusive and the months between 1 - 12, inclusive. Let the user know whether they entered a magic date. If the input parameters are not valid, return false. Examples: magicDate(6, 10, 60) -> true magicDate(50, 12, 600) –> falsearrow_forwardJAVA PPROGRAM ASAP Please create this program ASAP BECAUSE IT IS MY LAB ASSIGNMENT #2 so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #8. 8. Sum of Numbers in a String (page 610) Write a program that asks the user to enter a series of numbers separated by commas. Here are two examples of valid input: 7,9,10,2,18,6 8.777,10.9,11,2.2,-18,6 The program should calculate and display the sum of all the numbers. Input Validation 1. Make sure the string only contains numbers and commas (valid symbols are '0'-'9', '-', '.', and ','). 2. If the string is empty, a messages should be displayed, saying that the string is empty. Test Case 1 Enter a series of numbers separated only by commas or type QUIT to exit:\n7,9,10,2,18,6ENTERThe sum of those numbers is 52.00\nEnter a series of numbers separated only by commas or type QUIT to exit:\nENTEREmpty string.\nEnter a series of numbers separated only by…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
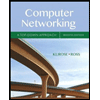
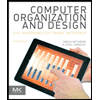
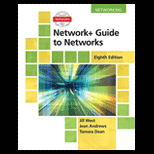
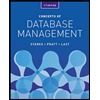
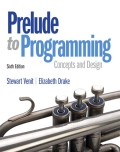
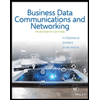