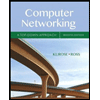
Put the following code in a main.py file and a module.py file. Define the names sort, list_max and sum_of_list after putting the code into a main file and a module file
#predefined modules
import random
import math
#function to sort the list in ascending order
def sort(x):
#predefined function sort()
x.sort()
#print the sorted list
print("\nSorted list is: ",str(x))
#function to find the sum of list elements
def sum_of_list(x):
#predefined function sum()
Sum=sum(x)
#return the sum of list elements
return Sum
#function to list the maximum from the list
def list_max(x):
#predefined function max()
maximum=max(x)
#return maximum
return maximum
#function to test the above three function
def main():
#set a flag variable
flag=True
#create a list
list1=list()
#initialize the list element by using randrange() predefined function of random module
list1=[random.randrange(1, 50, 1) for i in range(0,7)]
#print the original list
print("\nThe list element is shown below:\n" + str(list1))
#repeatative strurure while
while(flag):
# print the menu
print("\n******** Menu ********\n1. Sort the element\n2. Find the maximum from the list\n3. Find the sum of list element\n4. To exit the
# input user choice
ch = int(input("\nEnter your choice: "))
#decision struture
#if ch is 1
if(ch==1):
# sort the list
sort(list1)
#otherwise, if ch is 2
elif(ch==2):
# print the maximum from list
print("\nMaximum from the list element is: ", list_max(list1))
#otherwise, if ch is 3
elif(ch==3):
#print the sum of list
print("\nSum of list element is: ",sum_of_list(list1))
#otherwise
else:
flag=False
#function call
main()

Step by stepSolved in 4 steps with 5 images

- OCaml Code: Please read the attached instructions carefully and show the correct code with the screenshot of the output. I really need help with this assignment.arrow_forward5 partition_list (head) This is a little like split_list() from the Short problem, except that, instead of splitting the list into two by cutting it into the middle, you will now build two lists to return, using alternate values. The first value in the input list should be returned at the head of the first new list; the second value should be the head of the second list. Keep on alternating from there, putting one new value on the first list, and one on the second. (But remember that the length of the input list might be odd.) Example Suppose you have the following input list: 10 - 13 -> -1 -> 1000 - 0 It should return the following two lists: 10 1 0 13 -> 1000arrow_forwardC Programming Language Task: Deviation Write a program that prompts the user to enter N numbers and calculates which of the numbers has the largest deviation from the average of all numbers. You program should first prompt the user to enter how many numbers that will specify. The program should then scan for each number, separated by a newline. You should calculate the average value and return the number from the list which is furthest away from this average (to 2dp). Try using dynamic memory functions to store the incoming array of numbers on the heap. Code to build from: + 1 #include 2 #include 3 4 int main(void) { 5 6} 7 Output Example: deviation.c How many numbers? 5 Enter them: 1.0 2.0 6.0 3.0 4.0 Average: 3.20 Largest deviation from average: 6.00arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
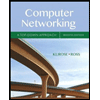
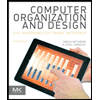
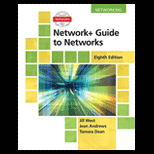
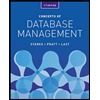
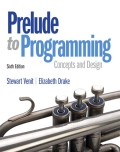
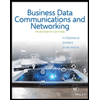