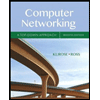
Pure Abstract Base Class Project
Define a pure abstract base class called BasicShape. The BasicShape class should
have the following members:
Private Member Variable:
area, a double used to hold the shape's area.
Public Member Functions:
getArea. This function should return the value in the member variable area.
calcArea. This function should be a pure virtual function.
Next, define a class named Circle. It should be derived from the BasicShape class. It
should have the following members:
Private Member Variables:
centerX, a long integer used to hold the x coordinate of the circle’s center.
centerY, a long integer used to hold the y coordinate of the circle’s center.
radius, a double used to hold the circle's radius.
Public Member Functions:
constructor—accepts values for centerX, centerY, and radius. Should call
the overridden calcArea function described below.
getCenterX—returns the value in centerX.
getCenterY—returns the value in centerY.
calcArea—calculates the area of the circle (area = 3.14159 * radius * radius)
and stores the result in the inherited member area.
Next, define a class named Rectangle. It should be derived from the BasicShape
class. It should have the following members:
Private Member Variables:
width, a long integer used to hold the width of the rectangle.
length, a long integer used to hold the length of the rectangle.
Public Member Functions:
constructor—accepts values for width and length. Should call the overridden
calcArea function described below.
getWidth—returns the value in width.
getLength—returns the value in length.
calcArea—calculates the area of the rectangle (area = length * width) and stores
the result in the inherited member area.
After you have created these classes, create a driver program that defines a Circle
object and a Rectangle object. Demonstrate that each object properly calculates and
reports its area.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- 1. Employee and ProductionWorker Classes Write an Employee class that keeps data attributes for the following pieces of information: Employee name Employee number Next, write a class named ProductionWorker that is a subclass of the Employee class. The ProductionWorker class should keep data attributes for the following information: Shift number (an integer, such as 1, 2, or 3) Hourly pay rate The workday is divided into two shifts: day and night. The shift attribute will hold an integer value representing the shift that the employee works. The day shift is shift 1 and the night shift is shift 2. Write the appropriate accessor and mutator methods for each class. Once you have written the classes, write a program that creates an object of the ProductionWorker class and prompts the user to enter data for each of the object’s data attributes. Store the data in the object, then use the object’s accessor methods to retrieve it and display it on the screen. 2. ShiftSupervisor Class In a…arrow_forwardThis type of member function may be called from a function that is a member of the same class or a derived class. static private protected O None of thesearrow_forwardIn the Member class, complete the function definition for SetNumPoints() that takes in the integer parameter newNumPoints. Ex: If the input is 4.5 62, then the output is: Height: 4.5 Number of points: 62 3 4 class Member { 5 6 7 8 9 10 11 12 17 } 1's public: void SetHeight (double newHeight); void SetNumPoints (int newNumPoints); 13 20 21 } double GetHeight() const; int GetNumPoints() const; private: 13 }; 14 15 void Member::SetHeight (double newHeight) { height= newHeight; 16 double height; int numPoints; ce es erabyCamScanner ur numPoints = newNumPoints;arrow_forward
- Programming Assignment #5 Educational Objectives: After completing this assignment the student should have the following knowledge, ability, and skills: Define a class hierarchy using inheritance Define virtual member functions in a class hierarchy Implement a class hierarchy using inheritance Implement virtual member functions in a class hierarchy Use initialization lists to call parent class constructors for derived class constructors Operational Objectives: Create (define and implement) a base class Shape classes that will inherit this class called Box, Rectangle, Circle, and Triangle Description: Programming Specifications: You will have a base class of Shape which will have an area and perimeter of type double. You must also create acceptable Gets and Sets for the private data items. All of the other classes will inherit this class. You will have a class Square which will have just one length of type double. You should create acceptable Gets and Sets for the one…arrow_forwardFill-in-the-Blank A constructor that takes a single parameter of a type different from the class type is a __________ constructor.arrow_forwardWhen a class declares an entire class as its friend, the friendship status is reciprocal. That is, each class's member functions have access to the other's private members. True Falsearrow_forward
- Using C++ define the class called Student. The Student class has the following: Private data members: name(string), age(int), units(int). The units represent the number of quarter units student is enrolled in. Define a default constructor as well as a constructor with parameters for the class Student. The class must have get and set functions for all private data members. The set function for the data member units must throw “out_of_range” exception if the number of units is not between 1 and 15. Include a function called tuition (double feePerUnit) that computes and returns the cost of registering for the number of units (in the private data member). The function receives the cost per unit as a parameter. Overload the operator (<<) to display student name and age. Test the class Student by writing a main program in which a Student object is created and displayed. Call the function tution(), you may pass any value as feePerUnit parameter to this function and display the…arrow_forward7. PersonData and CustomerData classesDesign a class named PersonData with the following member variables:lastNamefirstNameaddresscitystatezipphoneWrite the appropriate accessor and mutator functions for these member variables. Next, design a class named CustomerData, which is derived from the PersonData class. The CustomerData class should have the following member variables:customerNumbermailingListThe customerNumber variable will be used to hold a unique integer for each customer. The mailingList variable should be a bool. It will be set to true if the customer wishes to be on a mailing list, or false if the customer does not wish to be on a mail- ing list. Write appropriate accessor and mutator functions for these member variables. Demonstrate an object of the CustomerData class in a simple program.arrow_forwardWrite a class named Patient that has member variables for first name, middle name, last name, phone number, name and phone number of emergency contact. The Patient class should have a constructor that accepts and argument for each member variable. The Patient class should also have accessor and mutator functions for each member variable. Write a class named Procedure that has member variables for name of procedure, date of procedure, name of physician, and charges for the procedure. This Procedure class represents a medical procedure that is performed on a patient. The Procedure class should have a constructor that accepts an argument for each member variable. The Procedure class should also have accessor and mutator functions for each member variable. Then write a program that creates an instance of the Patient class initialized with sample data that is entered by the user. Then, create an instance of the Procedure class for each procedure that is being performed on the patient. There…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
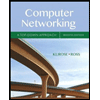
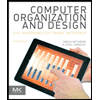
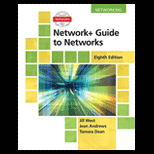
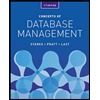
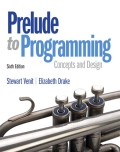
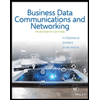